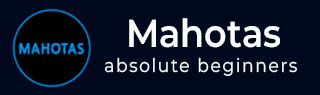
- Mahotas Tutorial
- Mahotas - Home
- Mahotas - Introduction
- Mahotas - Computer Vision
- Mahotas - History
- Mahotas - Features
- Mahotas - Installation
- Mahotas Handling Images
- Mahotas - Handling Images
- Mahotas - Loading an Image
- Mahotas - Loading Image as Grey
- Mahotas - Displaying an Image
- Mahotas - Displaying Shape of an Image
- Mahotas - Saving an Image
- Mahotas - Centre of Mass of an Image
- Mahotas - Convolution of Image
- Mahotas - Creating RGB Image
- Mahotas - Euler Number of an Image
- Mahotas - Fraction of Zeros in an Image
- Mahotas - Getting Image Moments
- Mahotas - Local Maxima in an Image
- Mahotas - Image Ellipse Axes
- Mahotas - Image Stretch RGB
- Mahotas Color-Space Conversion
- Mahotas - Color-Space Conversion
- Mahotas - RGB to Gray Conversion
- Mahotas - RGB to LAB Conversion
- Mahotas - RGB to Sepia
- Mahotas - RGB to XYZ Conversion
- Mahotas - XYZ to LAB Conversion
- Mahotas - XYZ to RGB Conversion
- Mahotas - Increase Gamma Correction
- Mahotas - Stretching Gamma Correction
- Mahotas Labeled Image Functions
- Mahotas - Labeled Image Functions
- Mahotas - Labeling Images
- Mahotas - Filtering Regions
- Mahotas - Border Pixels
- Mahotas - Morphological Operations
- Mahotas - Morphological Operators
- Mahotas - Finding Image Mean
- Mahotas - Cropping an Image
- Mahotas - Eccentricity of an Image
- Mahotas - Overlaying Image
- Mahotas - Roundness of Image
- Mahotas - Resizing an Image
- Mahotas - Histogram of Image
- Mahotas - Dilating an Image
- Mahotas - Eroding Image
- Mahotas - Watershed
- Mahotas - Opening Process on Image
- Mahotas - Closing Process on Image
- Mahotas - Closing Holes in an Image
- Mahotas - Conditional Dilating Image
- Mahotas - Conditional Eroding Image
- Mahotas - Conditional Watershed of Image
- Mahotas - Local Minima in Image
- Mahotas - Regional Maxima of Image
- Mahotas - Regional Minima of Image
- Mahotas - Advanced Concepts
- Mahotas - Image Thresholding
- Mahotas - Setting Threshold
- Mahotas - Soft Threshold
- Mahotas - Bernsen Local Thresholding
- Mahotas - Wavelet Transforms
- Making Image Wavelet Center
- Mahotas - Distance Transform
- Mahotas - Polygon Utilities
- Mahotas - Local Binary Patterns
- Threshold Adjacency Statistics
- Mahotas - Haralic Features
- Weight of Labeled Region
- Mahotas - Zernike Features
- Mahotas - Zernike Moments
- Mahotas - Rank Filter
- Mahotas - 2D Laplacian Filter
- Mahotas - Majority Filter
- Mahotas - Mean Filter
- Mahotas - Median Filter
- Mahotas - Otsu's Method
- Mahotas - Gaussian Filtering
- Mahotas - Hit & Miss Transform
- Mahotas - Labeled Max Array
- Mahotas - Mean Value of Image
- Mahotas - SURF Dense Points
- Mahotas - SURF Integral
- Mahotas - Haar Transform
- Highlighting Image Maxima
- Computing Linear Binary Patterns
- Getting Border of Labels
- Reversing Haar Transform
- Riddler-Calvard Method
- Sizes of Labelled Region
- Mahotas - Template Matching
- Speeded-Up Robust Features
- Removing Bordered Labelled
- Mahotas - Daubechies Wavelet
- Mahotas - Sobel Edge Detection
Mahotas - Zernike Features
Zernike features are a set of mathematical values that describe the shape of objects in an image. These value reflects specific details about the shape, such as its roundness, symmetry, or the presence of certain patterns.
The Zernike features have some special properties that make them useful for shape analysis. For example, they can describe the shape regardless of its size, meaning that the features remain the same even if the shape is rotated or scaled.
This property helps in recognizing objects under different conditions.
Zernike features work by breaking down the shape into smaller pieces using mathematical functions called Zernike polynomials. These polynomials act like building blocks, and by combining them, we can recreate and represent the shape of the object.
Zernike Features in Mahotas
To calculate the Zernike features in mahotas, we can use the mahotas.features.zernike() function.
In Mahotas, Zernike features are calculated by performing the following steps −
Step1 − Generate a set of Zernike polynomials, which are special mathematical functions representing various shapes and contours. These polynomials act as building blocks for analyzing the object's shape.
Step2 − Compute Zernike moments by projecting the shape of the object onto the Zernike polynomials. These moments capture important shape characteristics.
Step3 − Extract the Zernike features from the computed moments, which represent the essential shape information.
The mahotas.features.zernike() function
The mahotas.features.zernike() function takes three arguments: the image object, the maximum radius for the Zernike polynomials, and the number of features (degree) to compute. The function returns a 1−D array of the Zernike features for the image.
The degree of a Zernike moment is a measure of the complexity of the moment. The higher the degree, the more complex the moment.
Syntax
Following is the basic syntax of the mahotas.features.zernike() function −
mahotas.features.zernike(im, degree, radius, cm={center_of_mass(im)})
Where,
im − It is the input image on which the Zernike moments will be computed.
degree − It specifies the maximum number of the Zernike moments to be calculated.
radius − It defines the radius of the circular region, in pixels, over which the Zernike moments will be calculated. The area outside the circle defined by this radius, centered around the center of mass, is ignored.
cm (optional) − It specifies the center of mass of the image. By default, the center of mass of the image is used.
Example
Following is the basic example to calculate the Zernike features for shape recognition of an image −
import mahotas as mh # Load images of shapes image1 = mh.imread('sun.png', as_grey=True) # Compute Zernike features features = mh.features.zernike(image1, degree=8, radius=10) # Printing the features for shape recognition print(features)
Output
After executing the above code, we get the output as follows −
[0.31830989 0.00534998 0.00281258 0.0057374 0.01057919 0.00429721 0.00178094 0.00918145 0.02209622 0.01597089 0.00729495 0.00831211 0.00364554 0.01171028 0.02789188 0.01186194 0.02081316 0.01146935 0.01319499 0.03367388 0.01580632 0.01314671 0.02947629 0.01304526 0.00600012]
Using Custom Center of Mass
The center of mass of an image is the point in the image where the mass is evenly distributed. The custom center of mass is a point in an image that is not necessarily the center of mass of the image.
This can be useful in cases where you want to use a different center of mass for your calculations.
For example, you might want to use the custom center of mass of an object in an image to calculate the Zernike moments of the object.
To calculate the Zernike moments of an image using a custom center of mass in mahotas, we need to pass the cm parameter to the mahotas.features.zernike() function.
The cm parameter takes a tuple of two numbers, which represent the coordinates of the custom center of mass.
Example
In here, we are trying to calculate the Zernike features of an image using the custom center of mass −
import mahotas import numpy as np # Load the image image = mahotas.imread('nature.jpeg', as_grey = True) # Calculate the center of mass of the image center_of_mass = np.array([100, 100]) # Calculate the Zernike features of the image, using the custom center of mass zernike_features = mahotas.features.zernike(image, degree= 5, radius = 5, cm=center_of_mass) # Print the Zernike features print(zernike_features)
Output
Following is the output of the above code −
[3.18309886e-01 3.55572603e-04 3.73132619e-02 5.98944983e-04 3.23622041e-04 1.72293481e-04 9.16757235e-02 3.35704966e-04 7.09426259e-02 1.17847972e-04 2.12625026e-04 3.06537827e-04]
Calculating Zernike Features of Multiple Images
We can also calculate the Zernike features of multiple images in different formats. Following is the approach to achieve this −
Creates an empty list.
Use a for loop to iterate over the list of images.
Calculate the Zernike features of each image.
The features.zernike() function returns a vector of Zernike moments, which is then appended to the list of Zernike features.
Example
Now, we are trying to calculate the Zernike features of multiple images of different formats altogether −
import mahotas import numpy as np # Load the images images = [mahotas.imread('sun.png', as_grey = True), mahotas.imread('nature.jpeg', as_grey = True), mahotas.imread('tree.tiff', as_grey = True)] # Calculate the Zernike features of the images zernike_features = [] for image in images: zernike_features.append(mahotas.features.zernike(image, degree=2, radius = 2)) # Print the Zernike features print(zernike_features)
Output
Output of the above code is as follows −
[array([0.31830989, 0.05692079, 0.10311168, 0.01087613]), array([0.31830989, 0.02542476, 0.11556386, 0.01648607]), array([0.31830989, 0.12487805, 0.07212079, 0.03351757])]