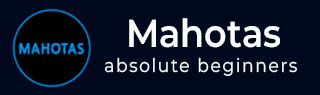
- Mahotas Tutorial
- Mahotas - Home
- Mahotas - Introduction
- Mahotas - Computer Vision
- Mahotas - History
- Mahotas - Features
- Mahotas - Installation
- Mahotas Handling Images
- Mahotas - Handling Images
- Mahotas - Loading an Image
- Mahotas - Loading Image as Grey
- Mahotas - Displaying an Image
- Mahotas - Displaying Shape of an Image
- Mahotas - Saving an Image
- Mahotas - Centre of Mass of an Image
- Mahotas - Convolution of Image
- Mahotas - Creating RGB Image
- Mahotas - Euler Number of an Image
- Mahotas - Fraction of Zeros in an Image
- Mahotas - Getting Image Moments
- Mahotas - Local Maxima in an Image
- Mahotas - Image Ellipse Axes
- Mahotas - Image Stretch RGB
- Mahotas Color-Space Conversion
- Mahotas - Color-Space Conversion
- Mahotas - RGB to Gray Conversion
- Mahotas - RGB to LAB Conversion
- Mahotas - RGB to Sepia
- Mahotas - RGB to XYZ Conversion
- Mahotas - XYZ to LAB Conversion
- Mahotas - XYZ to RGB Conversion
- Mahotas - Increase Gamma Correction
- Mahotas - Stretching Gamma Correction
- Mahotas Labeled Image Functions
- Mahotas - Labeled Image Functions
- Mahotas - Labeling Images
- Mahotas - Filtering Regions
- Mahotas - Border Pixels
- Mahotas - Morphological Operations
- Mahotas - Morphological Operators
- Mahotas - Finding Image Mean
- Mahotas - Cropping an Image
- Mahotas - Eccentricity of an Image
- Mahotas - Overlaying Image
- Mahotas - Roundness of Image
- Mahotas - Resizing an Image
- Mahotas - Histogram of Image
- Mahotas - Dilating an Image
- Mahotas - Eroding Image
- Mahotas - Watershed
- Mahotas - Opening Process on Image
- Mahotas - Closing Process on Image
- Mahotas - Closing Holes in an Image
- Mahotas - Conditional Dilating Image
- Mahotas - Conditional Eroding Image
- Mahotas - Conditional Watershed of Image
- Mahotas - Local Minima in Image
- Mahotas - Regional Maxima of Image
- Mahotas - Regional Minima of Image
- Mahotas - Advanced Concepts
- Mahotas - Image Thresholding
- Mahotas - Setting Threshold
- Mahotas - Soft Threshold
- Mahotas - Bernsen Local Thresholding
- Mahotas - Wavelet Transforms
- Making Image Wavelet Center
- Mahotas - Distance Transform
- Mahotas - Polygon Utilities
- Mahotas - Local Binary Patterns
- Threshold Adjacency Statistics
- Mahotas - Haralic Features
- Weight of Labeled Region
- Mahotas - Zernike Features
- Mahotas - Zernike Moments
- Mahotas - Rank Filter
- Mahotas - 2D Laplacian Filter
- Mahotas - Majority Filter
- Mahotas - Mean Filter
- Mahotas - Median Filter
- Mahotas - Otsu's Method
- Mahotas - Gaussian Filtering
- Mahotas - Hit & Miss Transform
- Mahotas - Labeled Max Array
- Mahotas - Mean Value of Image
- Mahotas - SURF Dense Points
- Mahotas - SURF Integral
- Mahotas - Haar Transform
- Highlighting Image Maxima
- Computing Linear Binary Patterns
- Getting Border of Labels
- Reversing Haar Transform
- Riddler-Calvard Method
- Sizes of Labelled Region
- Mahotas - Template Matching
- Speeded-Up Robust Features
- Removing Bordered Labelled
- Mahotas - Daubechies Wavelet
- Mahotas - Sobel Edge Detection
Mahotas - Removing Bordered Labelled
Removing bordered labeled refers to removing border regions in a labeled image. A labeled image consists of distinct regions that are assigned unique labels.
A border region of a labeled image refers to regions present along the edges (boundaries) of an image.
Border regions often make it difficult to analyze an image and, in some cases, represent significant noise.
Hence, it is important to remove border regions to improve accuracy of image segmentation algorithms and to reduce the overall size of the image.
Removing Bordered Labeled in Mahotas
In Mahotas, we can use mahotas.labeled.remove_bordering() function to remove border labels from an image. It analyzes the image to check for presence of any border label. If it finds a border label, it determines the value associated with that border label.
The value of the border label is then updated to 0, to remove it from the image. Since, value 0 is associated with the background, all the border labels become part of the background.
Sometimes, a border label can be far away from the image boundary. If we want to retain these border labels, we need to specify the minimum distance between a border region and the boundary. Any border region that exceeds this distance will be retained by the function.
The mahotas.labeled.remove_bordering() function
The mahotas.labeled.remove_bordering() function takes a labeled image as an input and returns a labeled image as output without having any border regions.
The function remove borders of all sizes, hence the output image consumes significantly less space than the input image.
Syntax
Following is the basic syntax of the remove_bordering() function in mahotas −
mahotas.labeled.remove_bordering(labeled, rsize=1, out={np.empty_like(im)})
Where,
labeled − It is the input labeled image.
rsize (optional) − It determines the minimum distance that regions must have from the image boundary in order to avoid being removed (default is 1).
out (optional) − It specifies where to store the output image (default is an array of same size as labeled).
Example
In the following example, we are removing border regions from an image by using the mh.labeled.remove_bordering() function.
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('nature.jpeg') # Converting it to grayscale image = mh.colors.rgb2gray(image) # Applying gaussian filtering image = mh.gaussian_filter(image, 4) # Thresholding the image image = image > image.mean() # Labeling the image labeled, num_objects = mh.label(image) # Removing bordering labels remove_border = mh.labeled.remove_bordering(labeled) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image) axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the image without borders axes[1].imshow(remove_border) axes[1].set_title('Border Removed Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
Output
Following is the output of the above code −
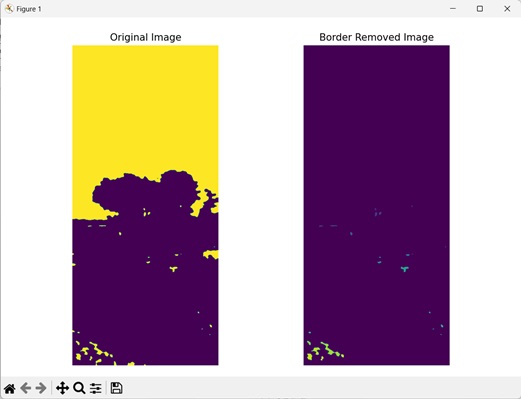
Removing Regions at a Specific Distance
We can also remove bordered regions that are at a specific distance, away from the image boundary. This allows us to remove any region that may have been considered as the bordered region because of its closeness to the image boundary.
In mahotas, the rsize parameter determines how far away a border region must be to be retained in the image. We need to set an integer value for this parameter and then pass it to the mh.labeled.remove_bordering() function.
For example, let’s say we have set ‘200’ as the value for rsize. Then, only the bordered regions that are at least 200 pixels away from the image boundary will be retained.
Example
In the example mentioned below, we are removing border regions that are within a specific distance of the image boundary.
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('sun.png') # Converting it to grayscale image = mh.colors.rgb2gray(image) # Applying gaussian filtering image = mh.gaussian_filter(image, 4) # Thresholding the image image = image > image.mean() # Labeling the image labeled, num_objects = mh.label(image) # Removing bordering labels remove_border = mh.labeled.remove_bordering(labeled, rsize=200) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image) axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the image without borders axes[1].imshow(remove_border) axes[1].set_title('Border Removed Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
Output
Output of the above code is as follows −
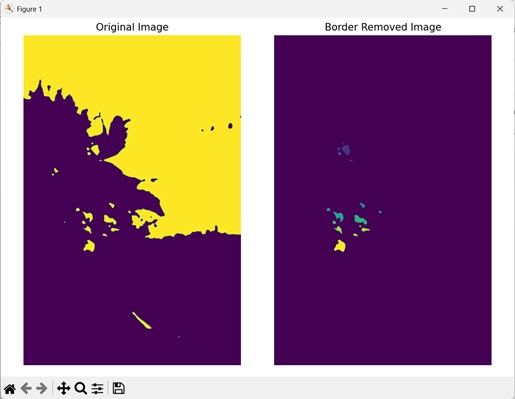
Removing Regions from a Specific part of an Image
Another way of removing bordered regions is to remove them from a specific part of an image.
Specific parts of an image refer to a small portion of the larger image obtained by cropping the larger image.
In mahotas, to remove regions from a specific part of an image, we first identify a region of interest from the original image.
Then, we crop the identified part of the image. We then remove the border regions from this part.
For example, if we specify the values as [:800, :800], then the region will start from 0 pixel and go up to 800 pixels in both the vertical (y−axis) and horizontal (x−axis) direction.
Example
In here, we are removing bordered regions from a specific part of an image.
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('tree.tiff') # Converting it to grayscale image = mh.colors.rgb2gray(image) image = image[:800, :800] # Applying gaussian filtering image = mh.gaussian_filter(image, 4) # Thresholding the image image = image > image.mean() # Labeling the image labeled, num_objects = mh.label(image) # Removing bordering labels remove_border = mh.labeled.remove_bordering(labeled) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image) axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the image without borders axes[1].imshow(remove_border) axes[1].set_title('Border Removed Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
Output
After executing the above code, we get the following output −
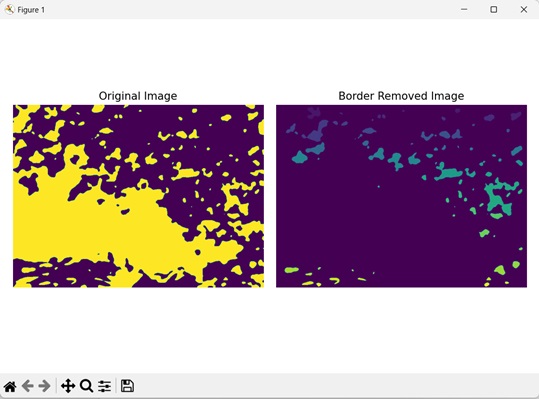