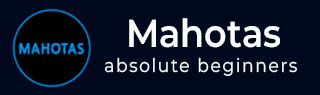
- Mahotas Tutorial
- Mahotas - Home
- Mahotas - Introduction
- Mahotas - Computer Vision
- Mahotas - History
- Mahotas - Features
- Mahotas - Installation
- Mahotas Handling Images
- Mahotas - Handling Images
- Mahotas - Loading an Image
- Mahotas - Loading Image as Grey
- Mahotas - Displaying an Image
- Mahotas - Displaying Shape of an Image
- Mahotas - Saving an Image
- Mahotas - Centre of Mass of an Image
- Mahotas - Convolution of Image
- Mahotas - Creating RGB Image
- Mahotas - Euler Number of an Image
- Mahotas - Fraction of Zeros in an Image
- Mahotas - Getting Image Moments
- Mahotas - Local Maxima in an Image
- Mahotas - Image Ellipse Axes
- Mahotas - Image Stretch RGB
- Mahotas Color-Space Conversion
- Mahotas - Color-Space Conversion
- Mahotas - RGB to Gray Conversion
- Mahotas - RGB to LAB Conversion
- Mahotas - RGB to Sepia
- Mahotas - RGB to XYZ Conversion
- Mahotas - XYZ to LAB Conversion
- Mahotas - XYZ to RGB Conversion
- Mahotas - Increase Gamma Correction
- Mahotas - Stretching Gamma Correction
- Mahotas Labeled Image Functions
- Mahotas - Labeled Image Functions
- Mahotas - Labeling Images
- Mahotas - Filtering Regions
- Mahotas - Border Pixels
- Mahotas - Morphological Operations
- Mahotas - Morphological Operators
- Mahotas - Finding Image Mean
- Mahotas - Cropping an Image
- Mahotas - Eccentricity of an Image
- Mahotas - Overlaying Image
- Mahotas - Roundness of Image
- Mahotas - Resizing an Image
- Mahotas - Histogram of Image
- Mahotas - Dilating an Image
- Mahotas - Eroding Image
- Mahotas - Watershed
- Mahotas - Opening Process on Image
- Mahotas - Closing Process on Image
- Mahotas - Closing Holes in an Image
- Mahotas - Conditional Dilating Image
- Mahotas - Conditional Eroding Image
- Mahotas - Conditional Watershed of Image
- Mahotas - Local Minima in Image
- Mahotas - Regional Maxima of Image
- Mahotas - Regional Minima of Image
- Mahotas - Advanced Concepts
- Mahotas - Image Thresholding
- Mahotas - Setting Threshold
- Mahotas - Soft Threshold
- Mahotas - Bernsen Local Thresholding
- Mahotas - Wavelet Transforms
- Making Image Wavelet Center
- Mahotas - Distance Transform
- Mahotas - Polygon Utilities
- Mahotas - Local Binary Patterns
- Threshold Adjacency Statistics
- Mahotas - Haralic Features
- Weight of Labeled Region
- Mahotas - Zernike Features
- Mahotas - Zernike Moments
- Mahotas - Rank Filter
- Mahotas - 2D Laplacian Filter
- Mahotas - Majority Filter
- Mahotas - Mean Filter
- Mahotas - Median Filter
- Mahotas - Otsu's Method
- Mahotas - Gaussian Filtering
- Mahotas - Hit & Miss Transform
- Mahotas - Labeled Max Array
- Mahotas - Mean Value of Image
- Mahotas - SURF Dense Points
- Mahotas - SURF Integral
- Mahotas - Haar Transform
- Highlighting Image Maxima
- Computing Linear Binary Patterns
- Getting Border of Labels
- Reversing Haar Transform
- Riddler-Calvard Method
- Sizes of Labelled Region
- Mahotas - Template Matching
- Speeded-Up Robust Features
- Removing Bordered Labelled
- Mahotas - Daubechies Wavelet
- Mahotas - Sobel Edge Detection
Mahotas - Majority Filter
The majority filter is used to remove the noise from an image. It works by looking at a pixel in an image and considering its neighboring pixels.
The majority filter calculates the most common pixel value among its neighbors and replaces the original pixel value with that common value.
Imagine you have a black−and−white image where white represents the object you're interested in and black represents the background.
However, due to various reasons, there might be some small black dots (noise) scattered around the object.
So to reduce the noise, it counts how many neighboring pixels are black and how many are white. Then, it replaces the original pixel value with the color (black or white) that appears most frequently among its neighbors.
Majority Filter in Mahotas
To apply the majority filter in mahotas, we can use the majority_filter() function.
The majority filter in Mahotas uses a structuring element to examine pixels in a neighborhood.
The structuring element counts the pixel values within the neightborhood and replaces the value of each pixel with the most common value to reduce noise.
The size of the structuring element determines the extent of smoothing. A larger neighborhood results in stronger smoothing effect, while reducing some finer details, whereas a smaller neightborhood results in less smoothing but maintains more details.
The mahotas.majority_filter() function
The majority_filter() function applies the majority filter to the input image using the specified neighborhood size.
It replaces each pixel value with the majority value among its neighbors. The filtered image is stored in the output array.
Syntax
Following is the basic syntax of the majority filter in mahotas −
mahotas.majority_filter(img, N=3, out={np.empty(img.shape, bool)})
Where,
img − It is the input image.
N − It is the size of the filter. It must be an odd integer. The default value is 3.
Out (optional) − It specifies the output array where the filtered image will be stored. It must be an empty boolean array with the same size as the input image.
Example
Following is the basic example to filter the image using the majority_filter() function −
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('picture.jpg', as_grey = True) filtered_image = mh.majority_filter(image) # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the majority filtered image axes[1].imshow(filtered_image, cmap='gray') axes[1].set_title('Majority Filtered') axes[1].axis('off') mtplt.show()
Output
After executing the above code, we get the following output −

By Specifying Window Size
To specify the window size in Mahotas, we need to pass it as a parameter to the majority_filter() function.
The window size is the number of pixels that will be used to determine the majority value for each pixel in the image.
The size of window must be an odd integer. This is because the majority filter works by finding the most common value in a neighborhood of pixels.
If the window size is even, there will be two pixels with the same value in the center of the window, and the majority filter will not be able to determine which value is the most common.
Example
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('picture.jpg', as_grey = True) # Specify a filter size filter_size = 19 # Apply majority filter with the specified filter size filtered_image = mh.majority_filter(image, N=filter_size) # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the majority filtered image axes[1].imshow(filtered_image, cmap='gray') axes[1].set_title('Majority Filtered') axes[1].axis('off') mtplt.show()
Output
Following is the output of the above code −
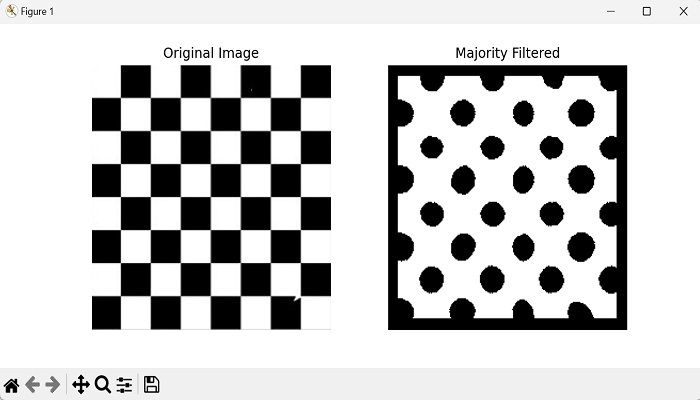
By Storing Result in an Output Array
We can store the result of the majority filter in an output array as well using Mahotas. To achieve this, we first need to create an empty array using the NumPy library.
This array is initialized with the same shape as the input image to store the resultant filtered image. The data type of the array is specified as bool, assuming a Boolean image.
Finally, we store the resultant filtered image in the output array by passing it as a parameter to the majority_filter() function.
Example
In here, we are trying to apply majority filter to a grayscale image and store the result in a specific output array −
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('pic.jpg', as_grey = True) # Create an output array for the filtered image output = np.empty(image.shape, dtype=bool) # Apply majority filter with a 3x3 neighborhood # store the result in the output array mh.majority_filter(image, N=3, out=output) # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the majority filtered image axes[1].imshow(output, cmap='gray') axes[1].set_title('Majority Filtered') axes[1].axis('off') mtplt.show()
Output
Output of the above code is as follows −
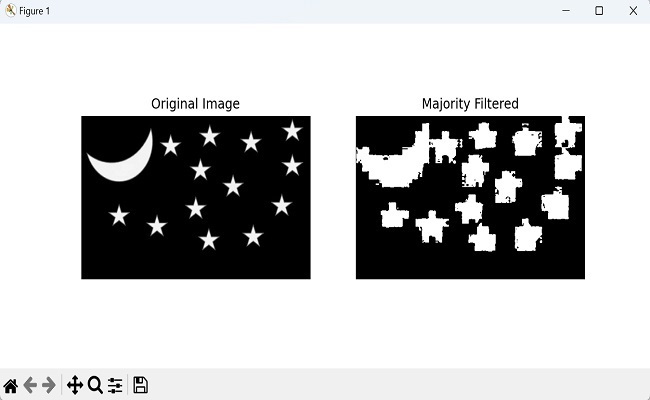