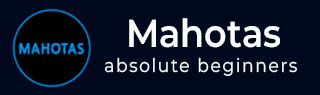
- Mahotas Tutorial
- Mahotas - Home
- Mahotas - Introduction
- Mahotas - Computer Vision
- Mahotas - History
- Mahotas - Features
- Mahotas - Installation
- Mahotas Handling Images
- Mahotas - Handling Images
- Mahotas - Loading an Image
- Mahotas - Loading Image as Grey
- Mahotas - Displaying an Image
- Mahotas - Displaying Shape of an Image
- Mahotas - Saving an Image
- Mahotas - Centre of Mass of an Image
- Mahotas - Convolution of Image
- Mahotas - Creating RGB Image
- Mahotas - Euler Number of an Image
- Mahotas - Fraction of Zeros in an Image
- Mahotas - Getting Image Moments
- Mahotas - Local Maxima in an Image
- Mahotas - Image Ellipse Axes
- Mahotas - Image Stretch RGB
- Mahotas Color-Space Conversion
- Mahotas - Color-Space Conversion
- Mahotas - RGB to Gray Conversion
- Mahotas - RGB to LAB Conversion
- Mahotas - RGB to Sepia
- Mahotas - RGB to XYZ Conversion
- Mahotas - XYZ to LAB Conversion
- Mahotas - XYZ to RGB Conversion
- Mahotas - Increase Gamma Correction
- Mahotas - Stretching Gamma Correction
- Mahotas Labeled Image Functions
- Mahotas - Labeled Image Functions
- Mahotas - Labeling Images
- Mahotas - Filtering Regions
- Mahotas - Border Pixels
- Mahotas - Morphological Operations
- Mahotas - Morphological Operators
- Mahotas - Finding Image Mean
- Mahotas - Cropping an Image
- Mahotas - Eccentricity of an Image
- Mahotas - Overlaying Image
- Mahotas - Roundness of Image
- Mahotas - Resizing an Image
- Mahotas - Histogram of Image
- Mahotas - Dilating an Image
- Mahotas - Eroding Image
- Mahotas - Watershed
- Mahotas - Opening Process on Image
- Mahotas - Closing Process on Image
- Mahotas - Closing Holes in an Image
- Mahotas - Conditional Dilating Image
- Mahotas - Conditional Eroding Image
- Mahotas - Conditional Watershed of Image
- Mahotas - Local Minima in Image
- Mahotas - Regional Maxima of Image
- Mahotas - Regional Minima of Image
- Mahotas - Advanced Concepts
- Mahotas - Image Thresholding
- Mahotas - Setting Threshold
- Mahotas - Soft Threshold
- Mahotas - Bernsen Local Thresholding
- Mahotas - Wavelet Transforms
- Making Image Wavelet Center
- Mahotas - Distance Transform
- Mahotas - Polygon Utilities
- Mahotas - Local Binary Patterns
- Threshold Adjacency Statistics
- Mahotas - Haralic Features
- Weight of Labeled Region
- Mahotas - Zernike Features
- Mahotas - Zernike Moments
- Mahotas - Rank Filter
- Mahotas - 2D Laplacian Filter
- Mahotas - Majority Filter
- Mahotas - Mean Filter
- Mahotas - Median Filter
- Mahotas - Otsu's Method
- Mahotas - Gaussian Filtering
- Mahotas - Hit & Miss Transform
- Mahotas - Labeled Max Array
- Mahotas - Mean Value of Image
- Mahotas - SURF Dense Points
- Mahotas - SURF Integral
- Mahotas - Haar Transform
- Highlighting Image Maxima
- Computing Linear Binary Patterns
- Getting Border of Labels
- Reversing Haar Transform
- Riddler-Calvard Method
- Sizes of Labelled Region
- Mahotas - Template Matching
- Speeded-Up Robust Features
- Removing Bordered Labelled
- Mahotas - Daubechies Wavelet
- Mahotas - Sobel Edge Detection
Mahotas - Otsu's Method
Otsu's method is a technique used for image segmentation to separate the foreground from the background. It works by finding a threshold value that maximizes the inter−class variance.
The inter−class variance is a measure of the separation between the foreground and the background regions.
The threshold value that maximizes the inter−class variance is considered the optimal threshold value for image segmentation.
Otsu's Method in Mahotas
In Mahotas, we can utilize the thresholding.otsu() function to calculate the threshold value using Otsu's method. The function operates in the following manner −
First it finds the histogram of the image. The histogram is a plot of the number of pixels in the image at each grayscale level.
Next, the threshold value is set to the average grayscale value of the image.
Then, the inter−class variance is calculated for the current threshold value.
The threshold value is then increased, and the inter−class variance is recalculated.
Steps 2 to 4 are repeated until an optimal threshold value is reached.
The mahotas.thresholding.otsu() function
The mahotas.thresholding.otsu() function takes a grayscale image as input and returns its threshold value calculated using Otsu's method. The pixels of the grayscale image are then compared to the threshold value to create a segmented image.
Syntax
Following is the basic syntax of the otsu() function in mahotas −
mahotas.thresholding.otsu(img, ignore_zeros=False)
Where,
img − It is the input grayscale image.
ignore_zeros (optional) − It a flag which specifies whether to ignore zero valued pixels (default is false).
Example
In the following example, we are using mh.thresholding.otsu() function to find the threshold value.
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('sea.bmp') # Converting it to grayscale image = mh.colors.rgb2gray(image).astype(np.uint8) # Calculating threshold value using Otsu method otsu_threshold = mh.thresholding.otsu(image) # Creating image from the threshold value final_image = image > otsu_threshold # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the threshold image axes[1].imshow(final_image, cmap='gray') axes[1].set_title('Otsu Threshold Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
Output
Following is the output of the above code −
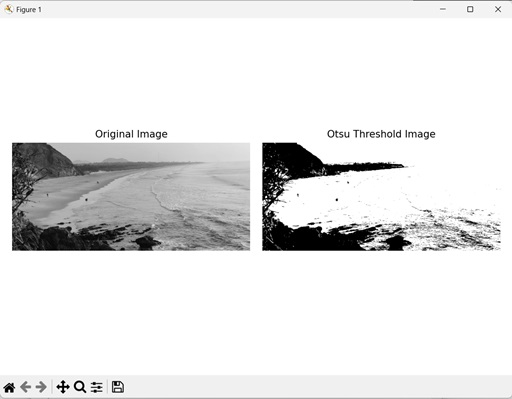
Ignoring the Zero Valued Pixels
We can also find Otsu's threshold value by ignoring the zero valued pixels. Zero valued pixels are pixels that have an intensity value of 0.
They usually represent the background pixels of an image, but in some images, they may also represent noise.
In grayscale images, zero valued pixels are pixels represented by the color 'black'.
To exclude zero valued pixels in mahotas, we can set the ignore_zeros parameter to the boolean value 'True'.
Example
In the example mentioned below, we are ignoring pixels with the value zero when calculating the threshold value using Otsu's method.
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('tree.tiff') # Converting it to grayscale image = mh.colors.rgb2gray(image).astype(np.uint8) # Calculating threshold value using Otsu method otsu_threshold = mh.thresholding.otsu(image, ignore_zeros=True) # Creating image from the threshold value final_image = image > otsu_threshold # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the threshold image axes[1].imshow(final_image, cmap='gray') axes[1].set_title('Otsu Threshold Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
Output
After executing the above code, we get the following output −
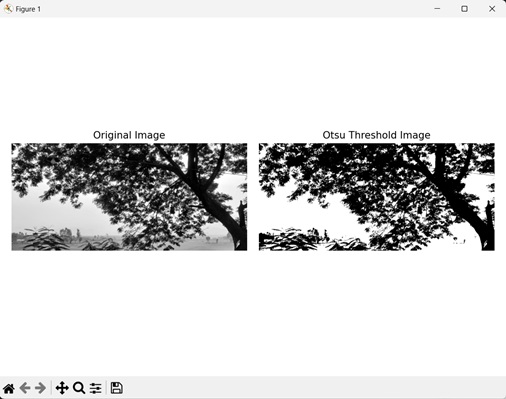