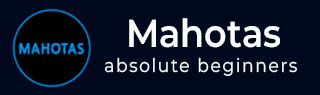
- Mahotas Tutorial
- Mahotas - Home
- Mahotas - Introduction
- Mahotas - Computer Vision
- Mahotas - History
- Mahotas - Features
- Mahotas - Installation
- Mahotas Handling Images
- Mahotas - Handling Images
- Mahotas - Loading an Image
- Mahotas - Loading Image as Grey
- Mahotas - Displaying an Image
- Mahotas - Displaying Shape of an Image
- Mahotas - Saving an Image
- Mahotas - Centre of Mass of an Image
- Mahotas - Convolution of Image
- Mahotas - Creating RGB Image
- Mahotas - Euler Number of an Image
- Mahotas - Fraction of Zeros in an Image
- Mahotas - Getting Image Moments
- Mahotas - Local Maxima in an Image
- Mahotas - Image Ellipse Axes
- Mahotas - Image Stretch RGB
- Mahotas Color-Space Conversion
- Mahotas - Color-Space Conversion
- Mahotas - RGB to Gray Conversion
- Mahotas - RGB to LAB Conversion
- Mahotas - RGB to Sepia
- Mahotas - RGB to XYZ Conversion
- Mahotas - XYZ to LAB Conversion
- Mahotas - XYZ to RGB Conversion
- Mahotas - Increase Gamma Correction
- Mahotas - Stretching Gamma Correction
- Mahotas Labeled Image Functions
- Mahotas - Labeled Image Functions
- Mahotas - Labeling Images
- Mahotas - Filtering Regions
- Mahotas - Border Pixels
- Mahotas - Morphological Operations
- Mahotas - Morphological Operators
- Mahotas - Finding Image Mean
- Mahotas - Cropping an Image
- Mahotas - Eccentricity of an Image
- Mahotas - Overlaying Image
- Mahotas - Roundness of Image
- Mahotas - Resizing an Image
- Mahotas - Histogram of Image
- Mahotas - Dilating an Image
- Mahotas - Eroding Image
- Mahotas - Watershed
- Mahotas - Opening Process on Image
- Mahotas - Closing Process on Image
- Mahotas - Closing Holes in an Image
- Mahotas - Conditional Dilating Image
- Mahotas - Conditional Eroding Image
- Mahotas - Conditional Watershed of Image
- Mahotas - Local Minima in Image
- Mahotas - Regional Maxima of Image
- Mahotas - Regional Minima of Image
- Mahotas - Advanced Concepts
- Mahotas - Image Thresholding
- Mahotas - Setting Threshold
- Mahotas - Soft Threshold
- Mahotas - Bernsen Local Thresholding
- Mahotas - Wavelet Transforms
- Making Image Wavelet Center
- Mahotas - Distance Transform
- Mahotas - Polygon Utilities
- Mahotas - Local Binary Patterns
- Threshold Adjacency Statistics
- Mahotas - Haralic Features
- Weight of Labeled Region
- Mahotas - Zernike Features
- Mahotas - Zernike Moments
- Mahotas - Rank Filter
- Mahotas - 2D Laplacian Filter
- Mahotas - Majority Filter
- Mahotas - Mean Filter
- Mahotas - Median Filter
- Mahotas - Otsu's Method
- Mahotas - Gaussian Filtering
- Mahotas - Hit & Miss Transform
- Mahotas - Labeled Max Array
- Mahotas - Mean Value of Image
- Mahotas - SURF Dense Points
- Mahotas - SURF Integral
- Mahotas - Haar Transform
- Highlighting Image Maxima
- Computing Linear Binary Patterns
- Getting Border of Labels
- Reversing Haar Transform
- Riddler-Calvard Method
- Sizes of Labelled Region
- Mahotas - Template Matching
- Speeded-Up Robust Features
- Removing Bordered Labelled
- Mahotas - Daubechies Wavelet
- Mahotas - Sobel Edge Detection
Mahotas - Template Matching
Template matching is a technique that is used to locate a specific image (a template) within a larger image. In simple terms, the goal is to find a place where the smaller image matches the larger image.
Template matching involves comparing the template image with different regions of the bigger image. The different properties of the template image such as size, shape, color, and intensity value are matched against the bigger image during comparison.
The comparison occurs until a region with the best match is found between the template image and the bigger image.
Template Matching in Mahotas
In Mahotas, we can use the mahotas.template_match() function to perform template matching. The function compares the template image to every region of the bigger image having the same size as the template image.
The function uses the sum of squared differences (SSD) method to perform template matching. The SSD method works in the following way −
The first step is to calculate the difference between the pixel values of the template image and the larger image.
In the next step, the differences are squared.
Finally, the squared differences are summed for all pixels in the larger image.
The final SSD values determine the similarity between the template image and the larger image. The smaller the value, the greater is the match between the template image and the larger image.
The mahotas.template_match() function
The mahotas.template_match() function takes an image and a template image as input.
It returns a region from the larger image that best matches the input template image.
The best match is the region which has the lowest SSD value.
Syntax
Following is the basic syntax of the template_match() function in mahotas −
mahotas.template_match(f, template, mode='reflect', cval=0.0, out=None)
Where,
f − It is the input image.
template − It is the pattern that will be matched against the input image.
mode (optional) − It determines how input image is extended when the template is applied near its boundaries (default is 'reflect').
cval (optional) − It is the constant value used in padding when mode is 'constant' (default is 0.0).
out (optional) − It defines the array in which the output image is stored (default is None).
Example
In the following example, we are performing template matching using the mh.template_match() function.
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the images image = mh.imread('tree.tiff', as_grey=True) template = mh.imread('cropped tree.tiff', as_grey=True) # Applying template matching algorithm template_matching = mh.template_match(image, template) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 3) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the template image axes[1].imshow(template, cmap='gray') axes[1].set_title('Template Image') axes[1].set_axis_off() # Displaying the matched image axes[2].imshow(template_matching, cmap='gray') axes[2].set_title('Matched Image') axes[2].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
Output
Following is the output of the above code −
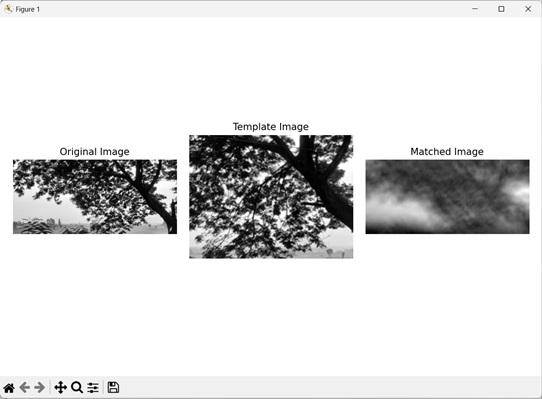
Matching by Wrapping Boundaries
We can wrap the boundaries of an image when performing template matching in Mahotas. Wrapping boundaries refers to folding the image boundaries to the opposite side of the image.
Thus, the pixels that are outside the boundary are repeated on the other side of the image.
This helps us in handling the pixels that are outside of the image boundaries during template matching.
In mahotas, we can wrap the boundaries of an image when performing template matching by specifying the value 'wrap' to the mode parameter of the template_match() function.
Example
In the example mentioned below, we are performing template matching by wrapping the boundaries of an image.
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the images image = mh.imread('sun.png', as_grey=True) template = mh.imread('cropped sun.png', as_grey=True) # Applying template matching algorithm template_matching = mh.template_match(image, template, mode='wrap') # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 3) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the template image axes[1].imshow(template, cmap='gray') axes[1].set_title('Template Image') axes[1].set_axis_off() # Displaying the matched image axes[2].imshow(template_matching, cmap='gray') axes[2].set_title('Matched Image') axes[2].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
Output
Output of the above code is as follows −
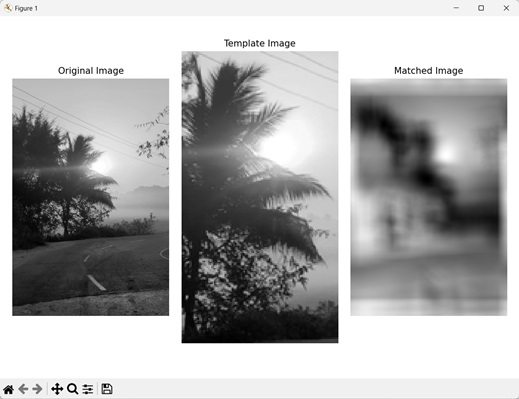
Matching by Ignoring Boundaries
We can also perform template matching by ignoring the boundaries of an image. The pixels that are beyond the boundaries of an image are excluded when performing template matching by ignoring boundaries.
In mahotas, we specify the value 'ignore' to the mode parameter of template_match() function to ignore the boundaries of an image, when performing template matching.
Example
In here, we are ignoring the boundaries of an image when performing template matching.
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the images image = mh.imread('nature.jpeg', as_grey=True) template = mh.imread('cropped nature.jpeg', as_grey=True) # Applying template matching algorithm template_matching = mh.template_match(image, template, mode='ignore') # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 3) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the template image axes[1].imshow(template, cmap='gray') axes[1].set_title('Template Image') axes[1].set_axis_off() # Displaying the matched image axes[2].imshow(template_matching, cmap='gray') axes[2].set_title('Matched Image') axes[2].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
Output
After executing the above code, we get the following output −
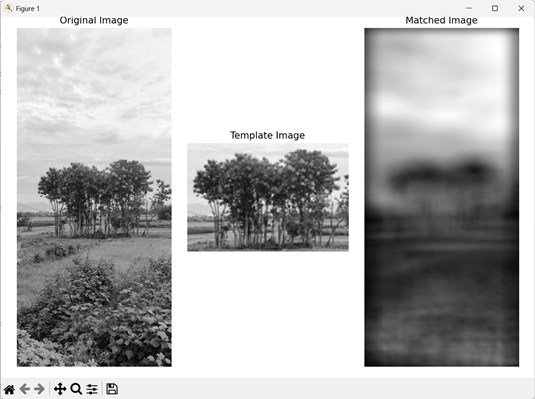