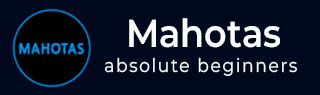
- Mahotas Tutorial
- Mahotas - Home
- Mahotas - Introduction
- Mahotas - Computer Vision
- Mahotas - History
- Mahotas - Features
- Mahotas - Installation
- Mahotas Handling Images
- Mahotas - Handling Images
- Mahotas - Loading an Image
- Mahotas - Loading Image as Grey
- Mahotas - Displaying an Image
- Mahotas - Displaying Shape of an Image
- Mahotas - Saving an Image
- Mahotas - Centre of Mass of an Image
- Mahotas - Convolution of Image
- Mahotas - Creating RGB Image
- Mahotas - Euler Number of an Image
- Mahotas - Fraction of Zeros in an Image
- Mahotas - Getting Image Moments
- Mahotas - Local Maxima in an Image
- Mahotas - Image Ellipse Axes
- Mahotas - Image Stretch RGB
- Mahotas Color-Space Conversion
- Mahotas - Color-Space Conversion
- Mahotas - RGB to Gray Conversion
- Mahotas - RGB to LAB Conversion
- Mahotas - RGB to Sepia
- Mahotas - RGB to XYZ Conversion
- Mahotas - XYZ to LAB Conversion
- Mahotas - XYZ to RGB Conversion
- Mahotas - Increase Gamma Correction
- Mahotas - Stretching Gamma Correction
- Mahotas Labeled Image Functions
- Mahotas - Labeled Image Functions
- Mahotas - Labeling Images
- Mahotas - Filtering Regions
- Mahotas - Border Pixels
- Mahotas - Morphological Operations
- Mahotas - Morphological Operators
- Mahotas - Finding Image Mean
- Mahotas - Cropping an Image
- Mahotas - Eccentricity of an Image
- Mahotas - Overlaying Image
- Mahotas - Roundness of Image
- Mahotas - Resizing an Image
- Mahotas - Histogram of Image
- Mahotas - Dilating an Image
- Mahotas - Eroding Image
- Mahotas - Watershed
- Mahotas - Opening Process on Image
- Mahotas - Closing Process on Image
- Mahotas - Closing Holes in an Image
- Mahotas - Conditional Dilating Image
- Mahotas - Conditional Eroding Image
- Mahotas - Conditional Watershed of Image
- Mahotas - Local Minima in Image
- Mahotas - Regional Maxima of Image
- Mahotas - Regional Minima of Image
- Mahotas - Advanced Concepts
- Mahotas - Image Thresholding
- Mahotas - Setting Threshold
- Mahotas - Soft Threshold
- Mahotas - Bernsen Local Thresholding
- Mahotas - Wavelet Transforms
- Making Image Wavelet Center
- Mahotas - Distance Transform
- Mahotas - Polygon Utilities
- Mahotas - Local Binary Patterns
- Threshold Adjacency Statistics
- Mahotas - Haralic Features
- Weight of Labeled Region
- Mahotas - Zernike Features
- Mahotas - Zernike Moments
- Mahotas - Rank Filter
- Mahotas - 2D Laplacian Filter
- Mahotas - Majority Filter
- Mahotas - Mean Filter
- Mahotas - Median Filter
- Mahotas - Otsu's Method
- Mahotas - Gaussian Filtering
- Mahotas - Hit & Miss Transform
- Mahotas - Labeled Max Array
- Mahotas - Mean Value of Image
- Mahotas - SURF Dense Points
- Mahotas - SURF Integral
- Mahotas - Haar Transform
- Highlighting Image Maxima
- Computing Linear Binary Patterns
- Getting Border of Labels
- Reversing Haar Transform
- Riddler-Calvard Method
- Sizes of Labelled Region
- Mahotas - Template Matching
- Speeded-Up Robust Features
- Removing Bordered Labelled
- Mahotas - Daubechies Wavelet
- Mahotas - Sobel Edge Detection
Mahotas - Haar Transform
Haar transform is a technique used to convert an image from pixel intensity values to wavelet coefficients. Wavelet coefficients are numerical values representing the contribution of different frequencies to an image.
In Haar transform, an image is broken into a set of orthonormal basis functions called Haar wavelets.
An orthonormal basis function refers to a mathematical function that satisfies two important properties : it is perpendicular (or orthogonal) to other basis functions, and its coefficients have a length of 1.
The basis functions are generated from a single wavelet by scaling and shifting. Scaling refers to changing the duration of the wavelet function, while shifting involves moving the wavelet function along the x−axis.
Haar Transform in Mahotas
In Mahotas, we can perform Haar transformation by using the mahotas.haar() function on an image. Following is the basic approach to perform Haar transformation on an image −
Image Partitioning − The first step involves dividing the input image into nonoverlapping blocks of equal size.
Averaging and Differencing − Next, the low and high frequency coefficients are computed within each block. The low frequency coefficient represents the smooth, global features of the image and is calculated as the average of pixel intensities. The high frequency coefficient represents the sharp, local features of the image and is calculated by finding differences between neighboring pixels.
Subsampling − The resultant low and high frequency coefficients are then down sampled (degraded) by discarding alternate values in each row and column.
Steps 2 and 3 are repeated until are repeated until the entire image has been transformed.
The mahotas.haar() function
The mahotas.haar() function takes a grayscale image as input and returns the wavelet coefficients as an image. The wavelet coefficients are a tuple of arrays.
The first array contains the low−frequency coefficients, and the second array contains the high−frequency coefficients.
Syntax
Following is the basic syntax of the haar() function in mahotas −
mahotas.haar(f, preserve_energy=True, inline=False)
Where,
f − It is the input image.
preserve_energy (optional) − It specifies whether to preserve the energy of the output image (default is True).
inline (optional) − It specifies whether to return a new image or modify input image (default is False).
Example
In the following example, we are applying Haar transformation on an image using the mh.haar() function.
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('sea.bmp') # Converting it to grayscale image = mh.colors.rgb2gray(image) # Applying Haar transformation haar_transform = mh.haar(image) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the Haar transformed image axes[1].imshow(haar_transform, cmap='gray') axes[1].set_title('Haar Transformed Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
Output
Following is the output of the above code −
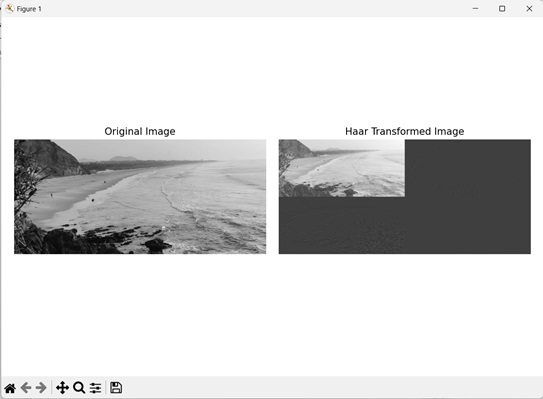
Without Preserving Energy
We can also perform Haar transformation on an image without preserving energy. The energy of the image refers to its brightness, and it can change when an image is being transformed from one domain to another.
In mahotas, the preserve_energy parameter of the mh.haar() function determines whether to preserve the energy of the output image. If we don’t want to preserve the energy, we can set this parameter to False.
Hence, the brightness of the output image will be different from the brightness of the input image.
If this parameter is set to True, then the output image and the input image will have the same brightness.
Example
In the example mentioned below, we are performing Haar transformation on an image without preserving its energy.
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('tree.tiff') # Converting it to grayscale image = mh.colors.rgb2gray(image) # Applying Haar transformation haar_transform = mh.haar(image, preserve_energy=False) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the Haar transformed image axes[1].imshow(haar_transform, cmap='gray') axes[1].set_title('Haar Transformed Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
Output
Output of the above code is as follows −
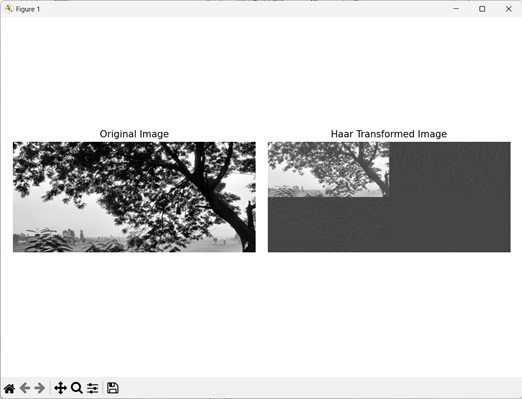
Inline Haar Transformation
We can also perform inline Haar transformation on an input image. Inline refers to applying transformation on the original image itself without creating a new image.
This allows us to save space when applying transformations on an image.
In mahotas, inline Haar transformation can be achieved by setting the inline parameter to boolean value True in the mh.haar() function. This way, a new image need not be created to store the output.
Example
In here, we are performing inline Haar transformation on an input image.
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('sun.png') # Converting it to grayscale image = mh.colors.rgb2gray(image) # Applying Haar transformation mh.haar(image, preserve_energy=False, inline=True) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 1) # Displaying the transformed image axes.imshow(image, cmap='gray') axes.set_title('Haar Transformed Image') axes.set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
Output
After executing the above code, we get the following output −

Note − Since the input image is getting overridden during transformation, the output screen will only contain a single image as seen above.