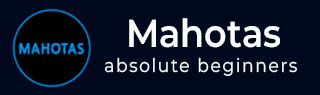
- Mahotas Tutorial
- Mahotas - Home
- Mahotas - Introduction
- Mahotas - Computer Vision
- Mahotas - History
- Mahotas - Features
- Mahotas - Installation
- Mahotas Handling Images
- Mahotas - Handling Images
- Mahotas - Loading an Image
- Mahotas - Loading Image as Grey
- Mahotas - Displaying an Image
- Mahotas - Displaying Shape of an Image
- Mahotas - Saving an Image
- Mahotas - Centre of Mass of an Image
- Mahotas - Convolution of Image
- Mahotas - Creating RGB Image
- Mahotas - Euler Number of an Image
- Mahotas - Fraction of Zeros in an Image
- Mahotas - Getting Image Moments
- Mahotas - Local Maxima in an Image
- Mahotas - Image Ellipse Axes
- Mahotas - Image Stretch RGB
- Mahotas Color-Space Conversion
- Mahotas - Color-Space Conversion
- Mahotas - RGB to Gray Conversion
- Mahotas - RGB to LAB Conversion
- Mahotas - RGB to Sepia
- Mahotas - RGB to XYZ Conversion
- Mahotas - XYZ to LAB Conversion
- Mahotas - XYZ to RGB Conversion
- Mahotas - Increase Gamma Correction
- Mahotas - Stretching Gamma Correction
- Mahotas Labeled Image Functions
- Mahotas - Labeled Image Functions
- Mahotas - Labeling Images
- Mahotas - Filtering Regions
- Mahotas - Border Pixels
- Mahotas - Morphological Operations
- Mahotas - Morphological Operators
- Mahotas - Finding Image Mean
- Mahotas - Cropping an Image
- Mahotas - Eccentricity of an Image
- Mahotas - Overlaying Image
- Mahotas - Roundness of Image
- Mahotas - Resizing an Image
- Mahotas - Histogram of Image
- Mahotas - Dilating an Image
- Mahotas - Eroding Image
- Mahotas - Watershed
- Mahotas - Opening Process on Image
- Mahotas - Closing Process on Image
- Mahotas - Closing Holes in an Image
- Mahotas - Conditional Dilating Image
- Mahotas - Conditional Eroding Image
- Mahotas - Conditional Watershed of Image
- Mahotas - Local Minima in Image
- Mahotas - Regional Maxima of Image
- Mahotas - Regional Minima of Image
- Mahotas - Advanced Concepts
- Mahotas - Image Thresholding
- Mahotas - Setting Threshold
- Mahotas - Soft Threshold
- Mahotas - Bernsen Local Thresholding
- Mahotas - Wavelet Transforms
- Making Image Wavelet Center
- Mahotas - Distance Transform
- Mahotas - Polygon Utilities
- Mahotas - Local Binary Patterns
- Threshold Adjacency Statistics
- Mahotas - Haralic Features
- Weight of Labeled Region
- Mahotas - Zernike Features
- Mahotas - Zernike Moments
- Mahotas - Rank Filter
- Mahotas - 2D Laplacian Filter
- Mahotas - Majority Filter
- Mahotas - Mean Filter
- Mahotas - Median Filter
- Mahotas - Otsu's Method
- Mahotas - Gaussian Filtering
- Mahotas - Hit & Miss Transform
- Mahotas - Labeled Max Array
- Mahotas - Mean Value of Image
- Mahotas - SURF Dense Points
- Mahotas - SURF Integral
- Mahotas - Haar Transform
- Highlighting Image Maxima
- Computing Linear Binary Patterns
- Getting Border of Labels
- Reversing Haar Transform
- Riddler-Calvard Method
- Sizes of Labelled Region
- Mahotas - Template Matching
- Speeded-Up Robust Features
- Removing Bordered Labelled
- Mahotas - Daubechies Wavelet
- Mahotas - Sobel Edge Detection
Mahotas - Median Filter
The median filter is another commonly used technique for noise reduction in an image. It works by calculating the middle (median) value among the neighboring pixels and replaces the original pixel value with that middle value.
To understand the median filter, let's consider the same black−and−white image scenario with small black dots representing noise. Each pixel in the image has a binary value − white (representing the object of interest) or black (representing the background).
For each pixel, the median filter takes the pixel values of its neighboring pixels within the window. It then arranges them in ascending order based on their intensity value.
After that, it selects the middle value, which is the median, and replaces the original pixel value with that median value.
Median Filter in Mahotas
To apply the median filter in Mahotas, you can use the median_filter() function.
The median filter function in Mahotas uses a structuring element to examine pixels in a neighborhood.
The structuring element replaces the value of each pixel by calculating the middle value within its neighborhood.
The size of the structuring element determines the extent of smoothing applied by the median filter.
A larger neighborhood will result in a stronger smoothing effect, while reducing finer details of the image. On the other hand, a smaller neighborhood will result in less smoothing but maintains more details.
The mahotas.median_filter() function
The median_filter() function applies the median filter to the input image using the specified neighborhood size. It replaces each pixel value with the median value calculated among its neighbors. The filtered image is stored in the output array.
Syntax
Following is the basic syntax of the median filter function in mahotas −
mahotas.median_filter(img, Bc={square}, mode='reflect', cval=0.0, out={np.empty(f.shape, f.dtype})
Where,
img − It is the input image.
Bc − It is the structuring element that defines the neighbourhood. By default, it is a square of side 3.
mode (optional) − It specifies how the function handles the borders of the image. It can take different values such as 'reflect', 'constant', 'nearest', 'mirror' or 'wrap'. By default, it is set to 'reflect'.
cval (optional) − The value to be used when mode='constant'. The default value is 0.0.
out (optional) − It specifies the output array where the filtered image will be stored. It must be of the same shape and data type as the input image.
Example
Following is the basic example to filter the image using the median_filter() function −
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('tree.tiff', as_grey = True) structuring_element = mh.disk(12) filtered_image = mh.median_filter(image, structuring_element) # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the median filtered image axes[1].imshow(filtered_image, cmap='gray') axes[1].set_title('Median Filtered') axes[1].axis('off') mtplt.show()
Output
After executing the above code, we get the following output −
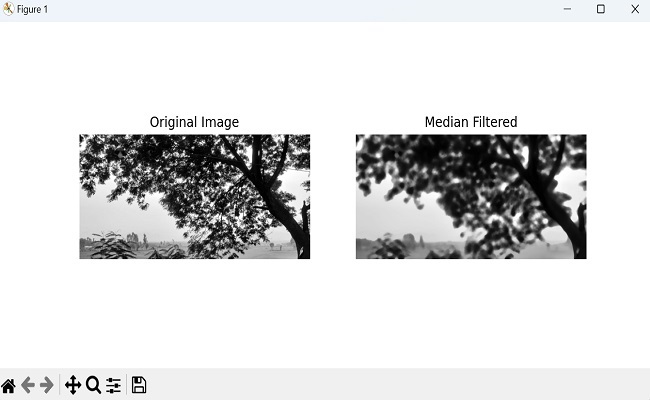
Median Filter with Reflect Mode
When we apply the median filter to an image, we need to consider the neighboring pixels around each pixel to calculate the median.
However, at the edges of the image, there are pixels that don't have neighbors on one or more sides.
To address this issue, we use the 'reflect' mode. Reflect mode creates a mirror−like effect along the edges of the image. It allows us to virtually extend the image by duplicating its pixels in a mirrored manner.
This way, we can provide the median filter with neighboring pixels even at the edges.
By reflecting the image values, the medan filter can now consider these mirrored pixels as if they were real neighbors.
It calculates the median value using these virtual neighbors, resulting in a more accurate smoothing process at the image edges.
Example
In here, we are trying to calculate the median filter with the reflect mode −
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('nature.jpeg', as_grey = True) structuring_element = mh.morph.dilate(mh.disk(12), Bc=mh.disk(12)) filtered_image = mh.median_filter(image, structuring_element, mode='reflect') # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the median filtered image axes[1].imshow(filtered_image, cmap='gray') axes[1].set_title('Median Filtered') axes[1].axis('off') mtplt.show()
Output
Output of the above code is as follows −
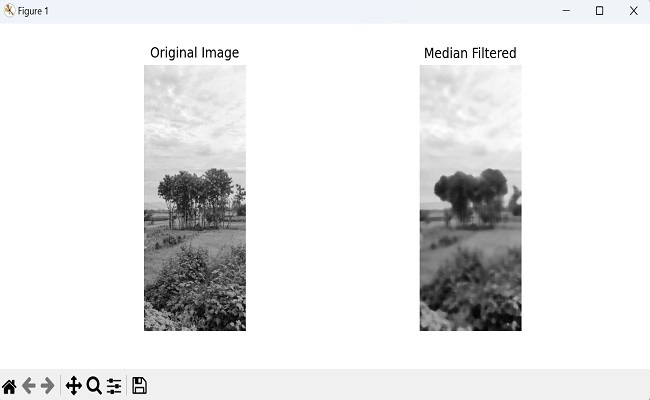
By Storing Result in an Output Array
We can store the result of the median filter in an output array as well using Mahotas. To achieve this, we first need to create an empty array using the NumPy library.
This array is initialized with the same shape and data type as the input image to store the resultant filtered image.
Finally, we store the resultant filtered image in the output array by passing it as a parameter to the median_filter() function.
Example
Now, we are trying to apply median filter to a grayscale image and store the result in a specific output array −
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('pic.jpg', as_grey = True) # Create an output array for the filtered image output = np.empty(image.shape) # store the result in the output array mh.median_filter(image, Bc=mh.disk(12), out=output) # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the median filtered image axes[1].imshow(output, cmap='gray') axes[1].set_title('Median Filtered') axes[1].axis('off') mtplt.show()
Output
Following is the output of the above code −
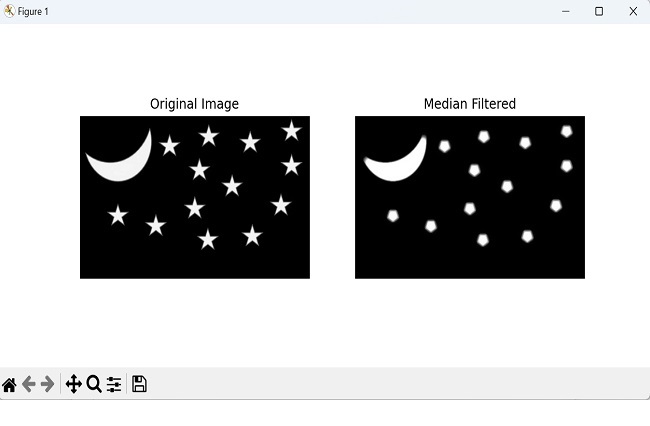