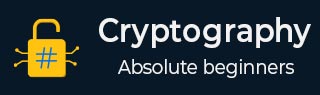
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Symmetric Algorithms
- Data Encryption Standard
- Triple DES
- Advanced Encryption Standard
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Electronic Code Book (ECB) Mode
The Electronic Code Book (ECB) is a basic block cipher mode of operation that is mostly used together with symmetric key encryption. It is a simple method for handling a list of message blocks that are listed in sequence.
There are several blocks in the input plaintext. The encryption key is used to independently and individually encrypt each block (ciphertext). Therefore, it is also possible to decrypt each encrypted block individually. Every kind of block can have a different encryption key supported by ECB.
Every plaintext block in ECB has a predefined ciphertext value that matches it, and vice versa. Hence, similar ciphertexts can always be encrypted from identical plaintexts using identical keys. This implies that the output ciphertext blocks will always be the same if plaintext blocks P1, P2, and so forth are encrypted several times using the same key.
In other words, the ciphertext value will always be equal to the plaintext value. This additionally holds true for plaintexts that include somewhat parts that are identical. For example, largely identical ciphertext parts will be included in plaintexts that have identical letter headers and are encrypted using the same key.
Operation
To create the first block of ciphertext, the user takes the first block of plaintext and encrypts it using the key.
He then uses the same procedure and key to go through the second block of plaintext, and so on.
Because the ECB mode is deterministic, the ciphertext blocks that are produced will be identical if plaintext blocks P1, P2,..., and Pm are encrypted twice with the same key.
In reality, we can technically generate a codebook of ciphertexts for every possible block of plaintext for a given key. Then, all that would be required for encryption would be to search for the necessary plaintext and choose the appropriate ciphertext. Because of this, the process is similar to assigning code words in a codebook, which is why it has an official name: Electronic Codebook mode of operation (ECB). Here's a visual representation of it −
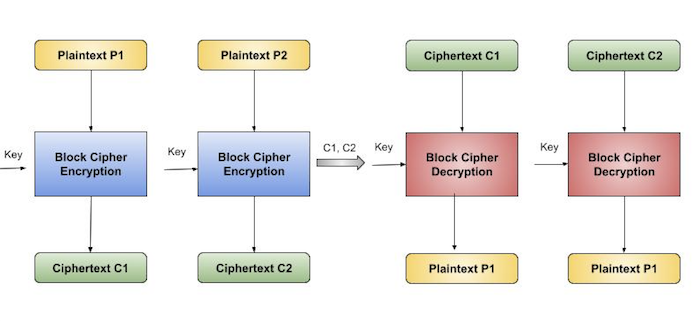
Analysis of ECB Mode
In real life, application data typically contain guessable partial information. For example, one can figure out the salary range. If the plaintext message is contained in a predictable area, an attacker may be able to decipher the ciphertext from ECB by trial and error.
For example, an attacker can retrieve a salary figure after a limited number of attempts if the salary figure is encrypted using a ciphertext from the ECB mode. Since most applications do not wish to use deterministic ciphers, the ECB mode should not be used in them.
Data Encryption Standard vs. Electronic Code Book
IBM created the Data Encryption Standard (DES) in the early 1970s, and in 1977 it was recognised as a Federal Information Processing Standard (FIPS). DES can encrypt data in five different ways. Among these is the original DES mode, or ECB.
FIPS Release 81 now includes three new options: Cipher Block Chaining (CBC), Cipher Feedback (CFB), and Output Feedback (OFB). Later, NIST Special Publication 800-38a was updated to include a fifth mode called Counter Mode. The design concepts of these modes vary, including whether using initialization vectors, whether to use blocks rather than streams, and whether or not encryption faults are likely to spread to subsequent blocks.
Implementation Using Python
This implementation performs ECB encryption and decryption using simple byte-level operations. It is crucial to keep in mind that, in most cases, ECB mode is not secure and needs to be substituted by more secure modes like CBC or GCM.
Below is a simple Python implementation of ECB mode encryption and decryption −
Example
# ECB encryption & decryption def pad(text, block_size): padding_length = block_size - (len(text) % block_size) padding = bytes([padding_length] * padding_length) return text + padding def unpad(padded_text): padding_length = padded_text[-1] return padded_text[:-padding_length] def xor_bytes(byte1, byte2): return bytes([a ^ b for a, b in zip(byte1, byte2)]) #Encryption Method def encrypt_ecb(key, plaintext): block_size = len(key) padded_plaintext = pad(plaintext, block_size) num_blocks = len(padded_plaintext) // block_size cipher_text = b'' for i in range(num_blocks): block_start = i * block_size block_end = block_start + block_size block = padded_plaintext[block_start:block_end] encrypted_block = xor_bytes(block, key) cipher_text += encrypted_block return cipher_text # Decryption Method def decrypt_ecb(key, ciphertext): block_size = len(key) num_blocks = len(ciphertext) // block_size plain_text = b'' for i in range(num_blocks): block_start = i * block_size block_end = block_start + block_size block = ciphertext[block_start:block_end] decrypted_block = xor_bytes(block, key) plain_text += decrypted_block return unpad(plain_text) # key and plaintext key = b'ABCDEFGHIJKLMNOP' # 16 bytes key for AES-128 plaintext = b'Hello, Tutorialspoint!' ciphertext = encrypt_ecb(key, plaintext) print("Ciphertext:", ciphertext) decrypted_plaintext = decrypt_ecb(key, ciphertext) print("Decrypted plaintext:", decrypted_plaintext.decode('utf-8'))
Output
Ciphertext: b"\t'/(*jg\x1c<>$>$/##1-**1gMBC@AFGDEZ" Decrypted plaintext: Hello, Tutorialspoint!
Drawbacks of ECB Mode
The following are some disadvantages of using ECB Mode −
ECB does not use chaining or an initialization vector; instead, it uses basic substitution. It is simple to implement because of these features. But this is also its biggest weakness. It is cryptologically weak because two identical blocks of plaintext provide two equally identical blocks of ciphertext.
When using identical encryption modes and small block sizes (less than 40 bits), ECB is not recommended. Some words and phrases may appear frequently in the plaintext when block sizes are short. It also means that the same repeating part-blocks of the ciphertext may appear, and that the ciphertext can carry patterns from the same plaintext. When plaintext patterns are easily recognised, hackers have a greater chance of figuring them out and executing a codebook attack.
Even though ECB security is not enough, each block's random pad bits could be added to improve it. Larger blocks (64 bits or more) may have enough entropy, or special characteristics, to prevent a codebook attack.
To Continue Learning Please Login