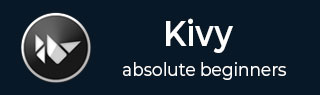
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Tabbed Panel
Many of the GUI toolkits include a tabbed panel, as it is very convenient to display the interface controls in groups instead of a big form, going well beyond the dimensions of the display device. TabbedPanel widget in Kivy makes it possible to display a widget or a layout in different panels, without making the GUI design look clumsy. The controls in different panels can share the data among themselves.
Different tabs are shown as a menu on top with a header area for the actual tab buttons and a content area for showing the current tab content.

The TabbedPanel object is the top level container for one or more panels. Corresponding to each panel, a TabbedPanelItem object is added. Each TabbedPAnelItem in turn, can hold any one widget or a layout that contains multiple widgets (such as GridLayout or BoxLayout etc)
Both these classes are defined in kivy.uix.tabbedpanel module.
from kivy.uix.tabbedpanel import TabbedPanel, TabbedPanelItem
A schematic statement flow to constructed a tabbed panel may be as follows −
main=TabbedPanel() tab1=TabbedPanelItem(text='Tab 1') Label=Label(text='Label') tab1.add_widget(label) tab2=TabbedPanelItem(text='Tab 2') btn=Button(text="Button") tab2.add_widget(btn) main.add_widget(tab1) main.add_widget(tab2)
The Tabbed panel can be further customized by tweaking a few properties −
You can choose the position in which the tabs are displayed, by setting the tab_pos property to either of the values - left_top, left_mid, left_bottom, top_left, top_mid, top_right, right_top, right_mid, right_bottom, bottom_left, bottom_mid, bottom_right.
Each tab has a special button TabbedPAnelHeader, containing a content property.
The tabbed panel comes with a default tab, which you can get rid of, by setting do_default_tab to False.
If the default tab is displayed, an on_default_tab event is provided for associating a callback −
tp.bind(default_tab = my_default_tab_callback)
Tabs and content can be removed in several ways −
tp.remove_widget() removes the tab and its content.
tp.clear_widgets() clears all the widgets in the content area.
tp.clear_tabs() removes the TabbedPanelHeaders
Example
In the example below, we use two tabbed panels, first to display a simple registration form, and in the other, a login form.
We shall us the "kv" language script to construct the design.
The default tab is removed.
The first tab holds a 2-column grid layout and contains labels and text input boxes for the user to enter his details, followed by a Submit button.
The second tab also has a two-column grid, with provision to enter email and password by the registered user.
TabbedPanel: size_hint: .8, .8 pos_hint: {'center_x': .5, 'center_y': .5} do_default_tab: False TabbedPanelItem: text:"Register Tab" GridLayout: cols:2 Label: text:"Name" size_hint:(.2, .1) pos_hint:{'x':.2, 'y':.75} TextInput: size_hint:(.4, .1) pos_hint:{'x':.3, 'y':.65} Label: text:"email" size_hint:(.2, .1) pos_hint:{'x':.2, 'y':.55} TextInput: size_hint:(.4, .1) pos_hint:{'x':.3, 'y':.45} Label: text:"Password" size_hint:(.2, .1) pos_hint:{'x':.2, 'y':.35} TextInput: password:True size_hint:(.4, .1) pos:(400, 150) pos_hint:{'x':.3, 'y':.25} Button: text:'Submit' size_hint : (.2, .1) pos_hint : {'center_x':.5, 'center_y':.09} TabbedPanelItem: text:'Login Tab' GridLayout: cols:2 Label: text:"email" size_hint:(.2, .1) pos_hint:{'x':.2, 'y':.55} TextInput: size_hint:(.4, .1) pos_hint:{'x':.3, 'y':.45} Label: text:"Password" size_hint:(.2, .1) pos_hint:{'x':.2, 'y':.35} TextInput: password:True size_hint:(.4, .1) pos:(400, 150) pos_hint:{'x':.3, 'y':.25} Button: text:'Submit' size_hint : (.2, .1) pos_hint : {'center_x':.5, 'center_y':.09}
The App code utilizing the above "kv" script design is as follows −
from kivy.app import App from kivy.core.window import Window Window.size = (720,300) class TabDemoApp(App): def build(self): pass TabDemoApp().run()
Output
When you run the above code, the app window shows the tabbed panel with the first tab contents exposed. Click the Login Tab to see the contents of the second tab.

To Continue Learning Please Login