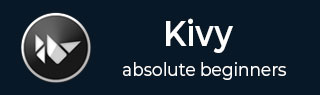
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Camera Handling
The Kivy framework supports Camera hardware through platform-specific providers. The "opncv-python" package enables camera support for Kivy on most operating systems. Hence it is recommended that the opencv-python package should be installed in Kivy's working environment.
In this chapter, we shall build a Camera app with Kivy library's Camera class. A Camera widget along with a ToggleButton and a normal Button are placed in a vertical box layout to construct the app interface.
The Camera instance starts with the initial play state as True, which means the app window will start the video stream from the camera as soon as it is loaded. The Toggle button stops the camera when it is down. It is bound to play() method. The Capture button will be in enabled state only when the camera is playing.
def play(self, instance): if instance.state=='down': self.mycam.play=False instance.text='Play' self.cb.disabled=True else: self.mycam.play=True instance.text='Stop' self.cb.disabled=False
The Capture button saves the current frame to a PNG file by calling export_to_png() method of the Camera object.
As the image is captured, Kivy pops up a message box with a title as Image Captured.
def capture(self, instance): if self.tb.text == 'Stop': self.mycam.export_to_png("IMG.png") layout = GridLayout(cols=1) popup = Popup( title='Image Captured', title_size=24, content=layout, auto_dismiss=True, size_hint=(None, None), size=(300, 100) ) popup.open()
The rest of the code involves the composition of the app interface inside the build() method.
Example
The complete code is given below −
from kivy.app import App from kivy.lang import Builder from kivy.uix.boxlayout import BoxLayout from kivy.uix.togglebutton import ToggleButton from kivy.uix.button import Button from kivy.uix.popup import Popup from kivy.uix.camera import Camera from kivy.core.window import Window Window.size = (720, 400) class TestCamera(App): def build(self): box = BoxLayout(orientation='vertical') self.mycam = Camera(play=True, resolution=(640, 480)) box.add_widget(self.mycam) self.tb = ToggleButton( text='Stop', size_hint_y=None, height='48dp', on_press=self.play ) box.add_widget(self.tb) self.cb = Button( text='Capture', size_hint_y=None, height='48dp', disabled=False, on_press=self.capture ) box.add_widget(self.cb) return box def play(self, instance): if instance.state == 'down': self.mycam.play = False instance.text = 'Play' self.cb.disabled = True else: self.mycam.play = True instance.text = 'Stop' self.cb.disabled = False def capture(self, instance): if self.tb.text == 'Stop': self.mycam.export_to_png("IMG.png") layout = GridLayout(cols=1) popup = Popup( title='Image Captured', title_size=24, content=layout, auto_dismiss=True, size_hint=(None, None), size=(300, 100) ) popup.open() TestCamera().run()
Output
The camera instance loads as the application starts. Note that depending on the system and the camera device, it may take a few seconds to start. The toggle button's caption is stop and the capture button is enabled.

If you press the Stop button down, the capture button gets disabled. When in enabled state, press the Capture button. The current frame will be saved as "img.png" with a popup box coming up.

To Continue Learning Please Login