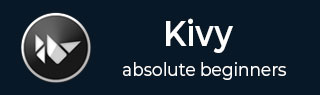
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Page Layout
The PageLayout class in Kivy is a little different from the other container widgets in Kivy. With PageLayout, you can create a simple multi-page layout so that it allows easy flipping from one page to another using borders.
Transitions from one page to the next are made by swiping in from the border areas on the right or left hand side. As PageLayout does not support the size_hint, size_hint_min, size_hint_max, or pos_hint properties, only one widget per page can be displayed. However, you can put multiple widgets in a page by putting a compound layout object such as box layout, grid layout or float layout in a single page.
The PageLayout class is defined in the "kivy.uix.pagelayout" module.
from kivy.uix.pagelayout import PageLayout pg = PageLayout(**kwargs)
You can define following properties as keyword arguments for the PageLayout constructor −
anim_kwargs − The animation kwargs used to construct the animation. It is a DictProperty and defaults to {'d': .5, 't': 'in_quad'}.
border − The width of the border around the current page used to display the previous/next page swipe areas when needed. It is a NumericProperty and defaults to 50dp.
page − The currently displayed page, which is a NumericProperty and defaults to 0.
swipe_threshold − The threshold used to trigger swipes as ratio of the widget size. swipe_threshold is a NumericProperty and defaults to 0.5.
PageLayout recognizes the touch events and you can override the following event handlers −
on_touch_down(touch) − Receive a touch down event with touch parameter as MotionEvent class. It returns a bool. If True, the dispatching of the touch event will stop. If False, the event will continue to be dispatched to the rest of the widget tree.
on_touch_move(touch) − Receive a touch move event. The touch is in parent coordinates.
n_touch_up(touch) − Receive a touch up event. The touch is in parent coordinates.
Here is a simple PageLayout example. We place three buttons, each as a separate page.
class PageApp(App): def build(self): pg = PageLayout() btn1 = Button(text ='Page 1') btn2 = Button(text ='Page 2') btn3 = Button(text ='Page 3') pg.add_widget(btn1) pg.add_widget(btn2) pg.add_widget(btn3) return pg
When run, the Button with page 1 caption displays. Swipe from right to left with the mouse pressed, to display the second and third pages. Swiping from left to right brings the previous pages in focus.

Example
In the following example, we add two float layouts in the PageLayout. The "kv" file is used to design the UI.
First, the Python code to run the PageLayout app −
from kivy.app import App from kivy.uix.label import Label from kivy.uix.button import Button from kivy.uix.textinput import TextInput from kivy.uix.floatlayout import FloatLayout from kivy.uix.pagelayout import PageLayout from kivy.config import Config Config.set('graphics', 'width', '720') Config.set('graphics', 'height', '400') Config.set('graphics', 'resizable', '1') class PageDemoApp(App): def build(self): pass if __name__ == '__main__': PageDemoApp().run()
Given below is the "PageDemo.kv" file script. The PageLayout embeds to FloatLayout objects. Upper float layout is the design of a registration page, and the bottom float layout is the second page consisting of login screen.
PageLayout: FloatLayout: orientation:'vertical' Label: text:'Register' font_size:'20pt' pos_hint:{'center_x': .5, 'center_y': .9} Label: text:"Name" size_hint:(.2, .1) pos_hint:{'x':.2, 'y':.75} TextInput: size_hint:(.4, .1) pos_hint:{'x':.3, 'y':.65} Label: text:"email" size_hint:(.2, .1) pos_hint:{'x':.2, 'y':.55} TextInput: size_hint:(.4, .1) pos_hint:{'x':.3, 'y':.45} Label: text:"Password" size_hint:(.2, .1) pos_hint:{'x':.2, 'y':.35} TextInput: password:True size_hint:(.4, .1) pos:(400, 150) pos_hint:{'x':.3, 'y':.25} Button: text:'Submit' size_hint : (.2, .1) pos_hint : {'center_x':.5, 'center_y':.09} FloatLayout: orientation:'vertical' size:(720,400) canvas.before: Color: rgba: 0,0,0, 1 Rectangle: pos: self.pos size: self.size Label: text:"Login" font_size: '30pt' pos_hint:{'center_x': .5, 'center_y': .75} Label: text:"email" size_hint:(.2, .1) pos_hint:{'x':.2, 'y':.55} TextInput: size_hint:(.4, .1) pos_hint:{'x':.3, 'y':.45} Label: text:"Password" size_hint:(.2, .1) pos_hint:{'x':.2, 'y':.35} TextInput: password:True size_hint:(.4, .1) pos:(400, 150) pos_hint:{'x':.3, 'y':.25} Button: text:'Submit' size_hint : (.2, .1) pos_hint : {'center_x':.5, 'center_y':.09}
Output
Save both the "PageDemoApp.py" and "PageDemo.kv" files, and run the Python script. You should first get the registration page.

Now, swipe the screen from right to left to make the login page appear in the application window −

To Continue Learning Please Login