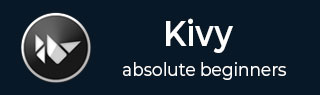
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Input Recorder
The functionality of Recorder class in Kivy framework is still under development, and is still at experimental stage. The Recorder object records the input events such as touch events, key events and click events. They are recorded in a file with a ".kvi" extension. Kivy uses this file and replays the events by generating equivalent fake events and dispatches them to the event loop.
The Recorder class is defined in the "kivy.input.recorder" module −
from kivy.input.recorder import Recorder rec = Recorder(**kwargs)
To start recording, press F8 after the Recorder object is instantiated. The event data is recorded in the "recorder.kvi" file in the current folder. You can specify any other filename to the file attribute.
rec = Recorder(filename='myrecorder.kvi')
Press F7 to replay the events.
To control the recording and replay manually, use the record and play properties of the Recorder object.
To start recording −
rec = Recorder(filename='myrecorder.kvi') rec.record = True rec.start()
To stop recording −
rec.record = False rec.stop()
Similarly, to start replay −
rec.play = True rec.start()
and, to stop playback −
rec.play = False rec.stop()
You can make the replay go on in a loop −
def playloop(instance, value): if value is False: instance.play = True rec = Recorder(filename='myrecorder.kvi') rec.bind(play=playloop) rec.play = True
Example
In the code given below, a Label is added to a Scatter widget, so that you can perform rotation, zooming and transformation. Further, the text property of the label updates as the user changes the contents of the TextInput box.
The recording and replay of events is defined on the on_press event of two buttons.
Here's the complete code −
from kivy.app import App from kivy.uix.label import Label from kivy.uix.scatter import Scatter from kivy.uix.boxlayout import BoxLayout from kivy.uix.textinput import TextInput from kivy.uix.togglebutton import ToggleButton from kivy.input.recorder import Recorder from kivy.core.window import Window Window.size = (720,400) class scatterdemoapp(App): def build(self): self.rec = Recorder(filename='myrecorder.kvi') box=BoxLayout(orientation='vertical') box1=BoxLayout(orientation='horizontal') text1=TextInput(text='Hi', pos_hint={'top':1},height=100, size_hint=(.5, None)) b1=ToggleButton(text='record',pos_hint={'top':1}, height=100, size_hint=(.25, None)) b1.bind(on_press=self.on_recording) b2=ToggleButton(text='play', pos_hint={'top':1},height=100, size_hint=(.25, None)) b2.bind(on_press=self.on_playing) box1.add_widget(text1) box1.add_widget(b1) box1.add_widget(b2) box.add_widget(box1) scatr=Scatter() self.lbl=Label(text="Hi", font_size=60, pos=(Window.width/2-100,200 )) text1.bind(text=self.lbl.setter('text')) scatr.add_widget(self.lbl) box.add_widget(scatr) return box def on_recording(self, obj): if obj.state=='down': self.rec.record=True self.rec.start() else: self.rec.record=False self.rec.stop() def on_playing(self, obj): if obj.state=='down': self.rec.play=True self.rec.start() else: self.rec.play=False self.rec.stop() scatterdemoapp().run()
Output
The App window appears as shown here −

Hit the record button and all the screen activities including the key_down events are recorded in the ".kvi" file. The console window shows that inputs have been recorded.
[INFO ] [Recorder ] Recording inputs to 'myrecorder.kvi' [INFO ] [Recorder ] Recorded 901 events in 'myrecorder.kvi'
On pressing the play button to replay the recorded events. Accordingly the console echoes the corresponding log.
[INFO ] [Recorder ] Start playing 901 events from 'myrecorder.kvi'
To Continue Learning Please Login