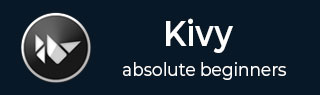
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Slider
In the Kivy framework, the Slider widget is an extremely useful control when you want to set value of a continuously variable numeric property. For example, the brightness of TV screen or mobile device or speaker sound.
The appearance of the slider widget is a horizontal or vertical bar with a knob sliding over it to set the value. When the knob is to the left of a horizontal slider, it corresponds to a minimum value; and when it is to the extreme right, it corresponds to the maximum.
The Slider class is defined in the "kivy.uix.slider" class.
from kivy.uix.slider import Slider slider = Slider(**kwargs)
To create a basic slider control in Kivy, we can use the following code −
from kivy.uix.slider import Slider s = Slider(min=0, max=100, value=25)
The default orientation of the slider control is horizontal. Set orientation='vertical' if needed. You should get a slider as shown below on the Kivy application window.

The knob can be moved along the slider with a mouse or touch (in case of a touchscreen).
To invoke a certain action on the basis of change in slider value, bind the value property to a certain callback.
def on_value_changed(self, instance, val): print (val) s.bind(value = on_value_changed)
Here are some of the important properties of the Slider class −
max − Maximum value allowed for value. max is a NumericProperty and defaults to 100.
min − Minimum value allowed for value. min is a NumericProperty and defaults to 0.
orientation − Orientation of the slider. orientation is an OptionProperty and defaults to 'horizontal'. Can take a value of 'vertical' or 'horizontal'.
padding − Padding of the slider. The padding is used for graphical representation and interaction. It prevents the cursor from going out of the bounds of the slider bounding box. padding is a NumericProperty and defaults to 16sp.
range − Range of the slider in the format (minimum value, maximum value) is a ReferenceListProperty of (min, max) properties.
sensitivity − Whether the touch collides with the whole body of the widget or with the slider handle part only. sensitivity is a OptionProperty and defaults to 'all'. Can take a value of 'all' or 'handle'.
step − Step size of the slider. Determines the size of each interval or step the slider takes between min and max. step is a NumericProperty and defaults to 0.
value − Current value used for the slider. value is a NumericProperty and defaults to 0.
value_track − Decides if slider should draw the line indicating the space between min and value properties values. It is a BooleanProperty and defaults to False.
value_track_color − Color of the value_line in rgba format. value_track_color is a ColorProperty and defaults to [1, 1, 1, 1].
value_track_width − Width of the track line, defaults to 3dp.
Example
In the code below, we use three slider widgets to let the user set RGB value for the desired color. The values of the sliders are used to Change the RGB values. For example, the RED slider change sets the value of 'r' component −
def on_red(self, instance, val): self.r = int(val)/255 self.colour=[self.r, self.g, self.b, self.t] self.redValue.text = str(int(val))
Similar callbacks for Green and Blue are also coded. These are assigned the value of colur variable which is of ColorProperty type. It is bound to the color property of a Label widget.
colour = ColorProperty([1,0,0,1]) def on_colour_change(self, instance, value): self.ttl.color=self.colour
As a result, setting RGB values by the sliders changes the text color of the Label.
Three slider controls along with the required labels are placed in an inner grid layout with four columns. An upper grid with one column houses a label, whose color is sought to be changed with the slider movement.
The complete code is given below −
from kivy.app import App from kivy.uix.gridlayout import GridLayout from kivy.uix.slider import Slider from kivy.uix.label import Label from kivy.properties import ColorProperty, NumericProperty from kivy.core.window import Window Window.size = (720, 400) class SliderExample(App): r = NumericProperty(0) g = NumericProperty(0) b = NumericProperty(0) t = NumericProperty(1) colour = ColorProperty([1, 0, 0, 1]) def on_colour_change(self, instance, value): self.ttl.color = self.colour def on_red(self, instance, val): self.r = int(val) / 255 self.colour = [self.r, self.g, self.b, self.t] self.redValue.text = str(int(val)) def on_green(self, instance, val): self.g = int(val) / 255 self.colour = [self.r, self.g, self.b, self.t] self.greenValue.text = str(int(val)) def on_blue(self, instance, val): self.b = int(val) / 255 self.colour = [self.r, self.g, self.b, self.t] self.blueValue.text = str(int(val)) def build(self): maingrid = GridLayout(cols=1) self.ttl = Label( text='Slider Example', color=self.colour, font_size=32 ) maingrid.add_widget(self.ttl) grid = GridLayout(cols=4) self.red = Slider(min=0, max=255) self.green = Slider(min=0, max=255) self.blue = Slider(min=0, max=255) grid.add_widget(Label(text='RED')) grid.add_widget(self.red) grid.add_widget(Label(text='Slider Value')) self.redValue = Label(text='0') grid.add_widget(self.redValue) self.red.bind(value=self.on_red) grid.add_widget(Label(text='GREEN')) grid.add_widget(self.green) grid.add_widget(Label(text='Slider Value')) self.greenValue = Label(text='0') grid.add_widget(self.greenValue) self.green.bind(value=self.on_green) grid.add_widget(Label(text='BLUE')) grid.add_widget(self.blue) grid.add_widget(Label(text='Slider Value')) self.blueValue = Label(text='0') grid.add_widget(self.blueValue) self.blue.bind(value=self.on_blue) self.bind(colour=self.on_colour_change) maingrid.add_widget(grid) return maingrid root = SliderExample() root.run()
Output
When you run this code, it will produce an output window like the one shown here −

To Continue Learning Please Login