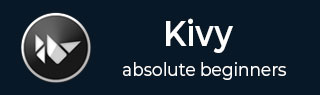
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Behaviors
In Kivy, the "kivy.uix.behaviors" module defines behavior mixins, which are also called "reusable classes" that provide additional functionality to widgets. They encapsulate common functionality and can be mixed in with multiple widgets to extend their behavior.
Behaviors help in keeping the code modular, reusable, and maintainable. They allow you to define your own implementation for standard kivy widgets that can act as drop-in replacements.
One of the applications of behavior mixins can be the use of an image as a button. We can define a custom class that extends ButtonBehavior to make it respond to events like "on_press" or "on_touch" so that the image itself can behave as a button. Later in this chapter, we shall have a look at the example of converting an image into a button.
The "kivy.uix.behaviors" module defines several mixins. Some of the most frequently used classes are explained below −
ButtonBehavior
This behavior provides button-like functionality to widgets. It adds features such as press/release visual feedback, automatic triggering of the "on_press" and "on_release" events, and handling of touch events.
It is often used with widgets like Button, ToggleButton, or custom widgets that need button-like behavior.
DragBehavior
This behavior class allows widgets to be dragged and moved by touch input. It handles touch events such as on_touch_down, on_touch_move, and on_touch_up to implement dragging functionality.
It is useful for creating draggable widgets in your application.
FocusBehavior
This behavior provides support for managing focus among widgets. It allows widgets to receive keyboard input and handle focus-related events.
It is useful for implementing keyboard navigation and managing focus traversal within your application.
SelectableBehavior
This behavior adds selection functionality to widgets. It allows users to select one or more items from a group of selectable widgets. It handles selection state, visual feedback, and triggering of selection-related events.
It is often used with widgets like ListView, RecycleView, or custom widgets that require selection functionality.
ButtonBehavior Example
We shall now develop a Kivy program to implement ButtonBehavior. We use Kivy's Image object to display an image on Kivy window. However, to add button-like behavior to it, we first define a custom class called imgbtn that extends Image as well as ButtonBehavior classes.
The source property of Image class is assigned a string which is the path to the image file. We then override the on_press() method as shown below −
from kivy.uix.image import Image from kivy.uix.behaviors import ButtonBehavior class imgbtn(ButtonBehavior, Image): def __init__(self, **kwargs): super(imgbtn, self).__init__(**kwargs) self.source = 'Logo.jpg' def on_press(self): print("Button pressed")
After this, the imgbtn class is defined. Let the build() method of the App class return its object.
Here is the ready-to-run code. You can save and run it −
from kivy.app import App from kivy.uix.image import Image from kivy.uix.behaviors import ButtonBehavior from kivy.config import Config # Configuration Config.set('graphics', 'width', '720') Config.set('graphics', 'height', '400') Config.set('graphics', 'resizable', '1') class imgbtn(ButtonBehavior, Image): def __init__(self, **kwargs): super(imgbtn, self).__init__(**kwargs) self.source = 'Logo.jpg' def on_press(self): print("Button pressed") class ImageApp(App): def build(self): return imgbtn() if __name__ == '__main__': ImageApp().run()
Output
Run the above program. It will display a Kivy window with the image at its center −

Note that the image itself acts as a button. To test, click the image and it will print the following message on the console −
Button pressed
To Continue Learning Please Login