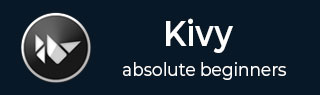
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Carousel
A carousel is a slideshow for cycling through a series of content. The Kivy framework includes a Carousel widget that allows you to easily create a browsable slideshow, particularly useful for touchscreen devices such as smartphones. The pages in a carousel can be moved horizontally or vertically.
The Carousel class is defined in the "kivy.uix.carousel" module.
from kivy.uix.carousel import Carousel carousel = Carousel(**kwargs)
A Python/Kivy program to create a simple slideshow with carousel is given below −
from kivy.app import App from kivy.uix.carousel import Carousel from kivy.uix.image import Image class CarouselApp(App): def build(self): carousel = Carousel(direction='right') img1=Image(source='1.png') carousel.add_widget(img1) img2=Image(source='2.png') carousel.add_widget(img2) img3=Image(source='3.png') carousel.add_widget(img3) return carousel CarouselApp().run()
You can also employ a "kv" language script to construct a carousel.
Carousel: direction: 'right' Image: source: '1.png' Image: source: '2.png' Image: source: '3.png' Image: source: '4.png'
The Carousel class defines following properties −
current_slide − The currently shown slide. current_slide is an AliasProperty.
direction − Specifies the direction in which the slides are ordered. This corresponds to the direction from which the user swipes to go from one slide to the next. It can be right, left, top, or bottom.
index − Get/Set the current slide based on the index. index defaults to 0 (the first item).
load_next(mode='next') − Animate to the next slide.
load_previous() − Animate to the previous slide.
load_slide(slide) − Animate to the slide that is passed as the argument.
loop − Allow the Carousel to loop infinitely. If True, when the user tries to swipe beyond last page, it will return to the first. If False, it will remain on the last page.
next_slide − The next slide in the Carousel. It is None if the current slide is the last slide in the Carousel.
previous_slide − The previous slide in the Carousel. It is None if the current slide is the first slide in the Carousel.
scroll_distance − Distance to move before scrolling the Carousel in pixels. Default distance id 20dp.
scroll_timeout − Timeout allowed to trigger the scroll_distance, in milliseconds. Defaults to 200 (milliseconds)
slides − List of slides inside the Carousel.
Example
As an example of Carousel in Kivy, have a look at the following code. The Carousel object is used as the root widget of the app, and we add a label, a button and an image as its slides.
from kivy.app import App from kivy.uix.button import Button from kivy.uix.carousel import Carousel from kivy.uix.image import Image from kivy.core.window import Window Window.size = (720,350) class CarouselApp(App): def build(self): carousel = Carousel(direction='right') carousel.add_widget(Button(text='Button 1', font_size=32)) src = "ganapati.png" image = Image(source=src, fit_mode="contain") carousel.add_widget(image) carousel.add_widget(Button(text="Button 2", font_size=32)) return carousel CarouselApp().run()
Output
This is a simple app to browse a series of slides by swiping across the display of your device. The direction parameter is set to right, which means that the subsequent slides are towards right.

Assembling the Carousel using "kv" Language
Let's use the "kv" language script to assemble the carousel. This time, the direction is set to top, which means you have to swipe up the screen to see the next display.
Example
Carousel: direction:'top' Button: text:'Button 1' font_size:32 Image: source:"kivy-logo.png" fit_mode:"contain" Button: text:"Button 2" font_size:32
Output
The slides are stacked one above the other.

To Continue Learning Please Login