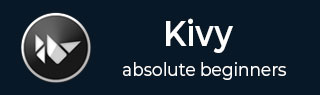
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Image Viewer
In this chapter, we shall build a simple Image Viewer app in Kivy. The code below uses Kivy's Image widget and buttons for navigation through the list of images in the selected folder. There is also a button which opens a FileChooser to let the user select different folder to view the images in.
First, we need to build a list of all the image files in the currently selected folder. We use the os.listdir() method for this purpose.
import os self.idx=0 self.fillist=[] dir_path = '.' for file in os.listdir(dir_path): if file.endswith('.png'): self.fillist.append(file)
The construction of app interface involves a vertical box layout, in which the Image object is placed on top and another horizontal box layout holding three buttons.
lo=BoxLayout(orientation='vertical') self.img= Image(source=self.fillist[self.idx]) lo.add_widget(self.img) hlo=BoxLayout(orientation='horizontal', size_hint=(1, .1)) self.b1 = Button(text = 'Dir', on_press=self.onButtonPress) self.b2 = Button(text = 'Prev', on_press=self.previmg) self.b3 = Button(text = 'Next', on_press=self.nextimg) hlo.add_widget(self.b1) hlo.add_widget(self.b2) hlo.add_widget(self.b3) lo.add_widget(hlo)
The Image widget displays the first available image to start with. When the button captioned "Next" is clicked, the index pointing to the filelist is incremented; and when the "Previous" button is clicked, it is decremented, loading the indexed image file in the Image object.
def previmg(self, instance): self.idx-=1 if self.idx<0: self.idx=0 self.img.source=self.fillist[self.idx] def nextimg(self, instance): self.idx+=1 if self.idx>=len(self.fillist): self.idx=len(self.fillist)-1 self.img.source=self.fillist[self.idx]
The third button (captioned Dir) pops up a FileChooser dialog. You can choose the required folder to view.
def onButtonPress(self, button): layout=GridLayout(cols=1) fw=FileChooserListView(dirselect=True, filters=["!*.sys"]) fw.bind(on_selection=self.onselect) closeButton = Button( text = "OK", size_hint=(None, None), size=(100,75) ) layout.add_widget(fw) layout.add_widget(closeButton) self.popup = Popup( title='Choose Folder', content=layout, auto_dismiss=False, size_hint=(None, None), size=(400, 400) ) self.popup.open()
Example
Images in the current folder populate the fillist to start with. Here is the complete code −
from kivy.app import App from kivy.uix.boxlayout import BoxLayout from kivy.uix.gridlayout import GridLayout from kivy.uix.label import Label from kivy.uix.popup import Popup from kivy.uix.image import Image from kivy.uix.button import Button from kivy.uix.filechooser import FileChooserListView from kivy.core.window import Window Window.size = (720, 400) class ImageViewerApp(App): def previmg(self, instance): self.idx -= 1 if self.idx < 0: self.idx = 0 self.img.source = self.fillist[self.idx] def nextimg(self, instance): self.idx += 1 if self.idx >= len(self.fillist): self.idx = len(self.fillist) - 1 self.img.source = self.fillist[self.idx] def onButtonPress(self, button): layout = GridLayout(cols=1) fw = FileChooserListView(dirselect=True, filters=["!*.sys"]) fw.bind(on_selection=self.onselect) closeButton = Button( text="OK", size_hint=(None, None), size=(100, 75) ) layout.add_widget(fw) layout.add_widget(closeButton) self.popup = Popup( title='Choose Folder', content=layout, auto_dismiss=False, size_hint=(None, None), size=(400, 400) ) self.popup.open() closeButton.bind(on_press=self.on_close) def onselect(self, *args): print(args[1][0]) def on_close(self, event): self.popup.dismiss() def build(self): import os self.idx = 0 self.fillist = [] dir_path = '.' for file in os.listdir(dir_path): if file.endswith('.png'): self.fillist.append(file) lo = BoxLayout(orientation='vertical') self.img = Image(source=self.fillist[self.idx]) lo.add_widget(self.img) hlo = BoxLayout(orientation='horizontal', size_hint=(1, .1)) self.b1 = Button(text='Dir', on_press=self.onButtonPress) self.b2 = Button(text='Prev', on_press=self.previmg) self.b3 = Button(text='Next', on_press=self.nextimg) hlo.add_widget(self.b1) hlo.add_widget(self.b2) hlo.add_widget(self.b3) lo.add_widget(hlo) return lo ImageViewerApp().run()
Output
When you run this code, it will display the image at index "0" −

Click the navigation buttons to go forward and back. Click the "Dir" button to select a new folder.

To Continue Learning Please Login