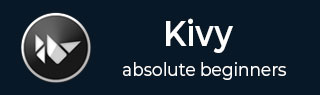
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Modal View
The ModalView widget in the Kivy framework is used to pop up a dialog box on top of the parent window. The behaviour of ModalView widget is similar to Kivy's Popup widget. In fact, the Popup class itself is a subclass of ModalView class, with a certain additional functionality, such as having a title and a separator.
By default, the size of Modalview is equal to that of "main" window. A widget's size_hint=(1, 1) by default. If you don't want your view to be fullscreen, either use size hints with values lower than 1 (for instance size_hint=(.8, .8)) or set the size_hint to None and use fixed size attributes.
The ModalView class is defined in kivy.uix.modalview import ModalView module. The following statements will produce a ModalView dialog −
from kivy.uix.modalview import ModalView view = ModalView(size_hint=(None, None), size=(300, 200)) view.add_widget(Label(text='ModalView Dialog'))
As in case of a Popup, the ModalView dialog is dismissed as you click outside it. To prevent this behavior, set auto-dismiss to False.
To make the ModalView dialog appear, you need to call its open() method.
view.open()
If auto_dismiss is False, you need to call its dismiss() method to close it.
view.dismiss()
Normally, both the open() and dismiss() method are invoked on a certain event such as on_press event of a button. You generally open a ModalView when a button on parent window is clicked, and dismissed when a buuton in ModalView layout is clicked.
ModalView Events
The ModalView emits events, before view is opened and dismissed, as well as when a view is opened and closed.
on_pre_open − Fired before the ModalView is opened. When this event is fired ModalView is not yet added to window.
on_open − Fired when the ModalView is opened.
on_pre_dismiss − Fired before the ModalView is closed.
on_dismiss − Fired when the ModalView is closed. If the callback returns True, the dismiss will be canceled.
Example
The following code presents an easy to implement example of ModalView dialog. The main application window displays a button caption as Click here. When clicked, a modal dialog pops up.
The ModalView is constructed by putting a label and a button inside a gridlayout. On the popup, we have a button with Ok as its caption. Its on_press event is bound to the dismiss() method of the ModalView object, causing it to disappear.
The complete code for the example is given below −
from kivy.app import App from kivy.uix.label import Label from kivy.uix.button import Button from kivy.uix.modalview import ModalView from kivy.uix.floatlayout import FloatLayout from kivy.uix.gridlayout import GridLayout from kivy.core.window import Window Window.size = (720, 300) class ModalViewDemoApp(App): def showmodal(self, obj): view = ModalView( auto_dismiss=False, size_hint=(None, None), size=(400, 100) ) grid = GridLayout(cols=1, padding=10) grid.add_widget(Label( text='ModalView Popup', font_size=32, color=[1, 0, 0, 1] )) btn = Button( text='ok', font_size=32, on_press=view.dismiss ) grid.add_widget(btn) view.add_widget(grid) view.open() def build(self): btn = Button( text='click here', font_size=32, on_press=self.showmodal, pos_hint={'center_x': .5, 'center_y': .1}, size_hint=(.3, None), size=(200, 100) ) return btn ModalViewDemoApp().run()
Output
The ModalView dialog will pop up when the button on the main app window is clicked.

To Continue Learning Please Login