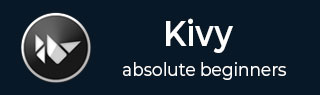
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Box Layouts
Kivy framework provides BoxLayout class with which we can arrange the widgets sequentially. The sequence is determined by the orientation property of BoxLayout object and can be a string: either 'vertical' or 'horizontal'.
The BoxLayout class is defined in the "kivy.uix.boxlayout" module. To declare a BoxLayout object, use.
from kivy.uix.boxlayout import BoxLayout blo = BoxLayout(**kwargs)
Properties
orientation − Orientation of the layout. orientation is an OptionProperty and defaults to 'horizontal'. Can be 'vertical' or 'horizontal'.
padding − Padding between layout box and children: [padding_left, padding_top, padding_right, padding_bottom]. padding also accepts a two argument form [padding_horizontal, padding_vertical] and a one argument form [padding].
minimum_height − Automatically computed minimum height needed to contain all children. The minimum_height is a NumericProperty and defaults to 0. It is read only.
minimum_size − Automatically computed minimum size needed to contain all children.minimum_size is a ReferenceListProperty of (minimum_width, minimum_height) properties. It is read only.
minimum_width − Automatically computed minimum width needed to contain all children. minimum_width is a NumericProperty and defaults to 0. It is read only.


The BoxLayout class also inherits the add_widget() and remove_widget() methods, which we have already discussed earlier.
Vertical BoxLayout
Typical use of BoxLayout is shown here. We add a label, a text input and a button in a vertical box layout.
Example
from kivy.app import App from kivy.uix.label import Label from kivy.uix.button import Button from kivy.uix.textinput import TextInput from kivy.uix.boxlayout import BoxLayout from kivy.core.window import Window Window.size = (720,200) class DemoApp(App): def build(self): lo = BoxLayout(orientation = 'vertical') self.l1 = Label(text='Enter your name', font_size=20) self.t1 = TextInput(font_size = 30) self.b1 = Button(text = 'Submit', size_hint = (None, None),pos_hint={'x':.4, 'y':.2}, size = (200,75)) lo.add_widget(self.l1) lo.add_widget(self.t1) lo.add_widget(self.b1) return lo if __name__ == '__main__': DemoApp().run()
Output
It will produce the following output −

You can use the following "Demo.kv" file to construct the above GUI −
BoxLayout: orientation : 'vertical' Label: id : l1 text : 'Enter your name' font_size : '20pt' TextInput: id : t1 font_size : 30 Button: id : b1 text : 'Submit' size_hint : (None, None) pos_hint : {'x':.4, 'y':.2} size : (200,75)
Horizontal BoxLayout
In the following program, we have placed a label, a text input box and a button in Box layout with horizontal orientation.
Example
from kivy.app import App from kivy.uix.label import Label from kivy.uix.button import Button from kivy.uix.textinput import TextInput from kivy.uix.boxlayout import BoxLayout from kivy.clock import Clock from kivy.core.window import Window Window.size = (720,200) class DemoApp(App): def build(self): self.lo = BoxLayout(orientation = 'horizontal') self.l1 = Label(text='Enter your name', font_size=20) self.t1 = TextInput(font_size = 30, pos_hint={'y':0.25}, pos = (0,100), size_hint = (None, None), size = (650,100)) self.b1 = Button(text = 'Submit', size_hint = (None, None),pos_hint={'x':.4, 'y':.35}, size = (75, 40)) self.lo.add_widget(self.l1) self.lo.add_widget(self.t1) self.lo.add_widget(self.b1) return self.lo if __name__ == '__main__': DemoApp().run()
Output
It will produce the following output −

You can obtain the same GUI design with the following "Demo.kv" file −
BoxLayout: orientation : 'horizontal' Label: text : 'Enter your name' font_size : '20pt' TextInput : font_size : '30pt' pos_hint : {'y':0.25} pos : (0,100) size_hint : (None, None) size : (650,100) Button : text : 'Submit' size_hint : (None, None) pos_hint : {'x':.4, 'y':.35} size : (75, 40)
To Continue Learning Please Login