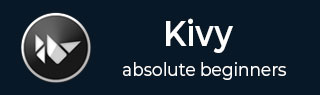
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Data Loader
A Loader class in Kivy framework is an asynchronous data loader that makes it possible to load an image even if its data is not yet available. This feature is of particular use when you want to load image from an internet URL.
The Loader class is defined in kivy.loader module. Typical use of Loader class is like this −
from kivy.loader import Loader image = Loader.image('http://mysite.com/test.png')
Use the loading_image property to specify a default image.
Loader.loading_image = Image(default.png')
The Loader class is equipped with the following properties −
error_image − Image used for error. For example −
Loader.error_image = 'error.png'
image(filename) − Load a image using the Loader. A ProxyImage is returned with a loading image.
loading_image − Image used for loading. For example −
Loader.loading_image = 'loading.png'
max_upload_per_frame − The number of images to upload per frame. By default, we'll upload only 2 images to the GPU per frame.
num_workers − Number of workers to use while loading. This setting impacts the loader only on initialization. Once the loader is started, the setting has no impact.
from kivy.loader import Loader Loader.num_workers = 4
The default value is "2" for giving a smooth user experience.
ProxyImage() − Image returned by the Loader.image() function.
proxyImage = Loader.image("test.jpg")
pause() − Pause the loader.
resume() − Resume the loader, after a pause().
run() − Main loop for the loader.
start() − Start the loader thread/process.
stop() − Stop the loader thread/process.
The "on_load" event is fired when the image is loaded or changed. Similarly, the "on_error" is fired when the image cannot be loaded. "error: Exception data that occurred".
Example
In the code given below, the Loader object loads an image from an internet URL. The ProxyImage object returned by the Loader binds to a method on its on_load event. The callback method uses its texture as the texture property of the Image object.
from kivy.app import App from kivy.uix.image import Image from kivy.loader import Loader from kivy.core.window import Window Window.size = (720,400) class MyApp(App): title='Loader' def _image_loaded(self, proxyImage): if proxyImage.image.texture: self.image.texture = proxyImage.image.texture def build(self): proxyImage = Loader.image('https://source.unsplash.com/user/c_v_r/640x480') proxyImage.bind(on_load=self._image_loaded) self.image = Image() return self.image MyApp().run()
Output
On execution, it will produce the following output −

To Continue Learning Please Login