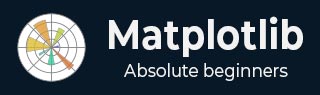
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - Linear and Logarthmic Scales
- Matplotlib - Symmetrical Logarithmic and Logit Scales
- Matplotlib - LaTeX
- Matplotlib - What is LaTeX?
- Matplotlib - LaTeX for Mathematical Expressions
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Enabling LaTex Rendering in Annotations
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Reverse Axes
What is Reverse Axes?
In Matplotlib reverse axes refers to changing the direction of an axis, flipping it from its default orientation. This action alters the visual representation of the plot by reversing the order of the data along a specific axis usually the x-axis or y-axis.
Reversing X-axis
To reverse the x-axis in Matplotlib we can use the plt.gca().invert_xaxis() function. This method inverts the direction of the x-axis effectively flipping the plot horizontally. Data points that were originally plotted from left to right will now be displayed from right to left.
Here is a detailed breakdown of how to reverse the x-axis:
Steps to Reverse the X-axis
The below are the steps to be followed to reverse the x-axis.
Create a Plot
Generate a plot with our data using Matplotlib.
Example
import matplotlib.pyplot as plt # Sample data x = [1, 2, 3, 4, 5] y = [2, 4, 6, 8, 10] # Plot with default axis orientation plt.plot(x, y, marker='o') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Default X-axis') plt.show()
Output
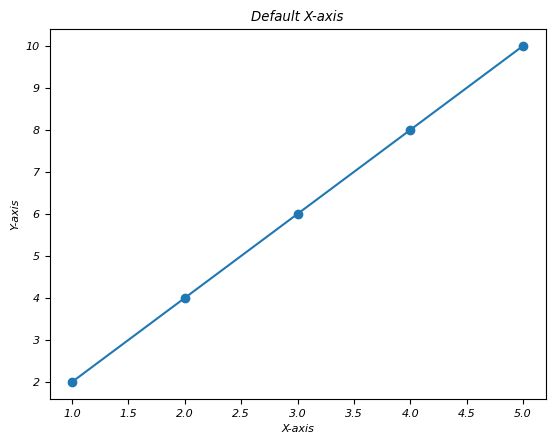
Reverse the X-axis
After creating the plot use plt.gca().invert_xaxis() to reverse the x-axis.
Example
import matplotlib.pyplot as plt # Sample data x = [1, 2, 3, 4, 5] y = [2, 4, 6, 8, 10] # Plot with default axis orientation plt.plot(x, y, marker='o') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Default X-axis') plt.show() # Reversing the x-axis plt.plot(x, y, marker='o') plt.gca().invert_xaxis() # Reverse x-axis plt.xlabel('Reversed X-axis') plt.ylabel('Y-axis') plt.title('Reversed X-axis') plt.show()
Output
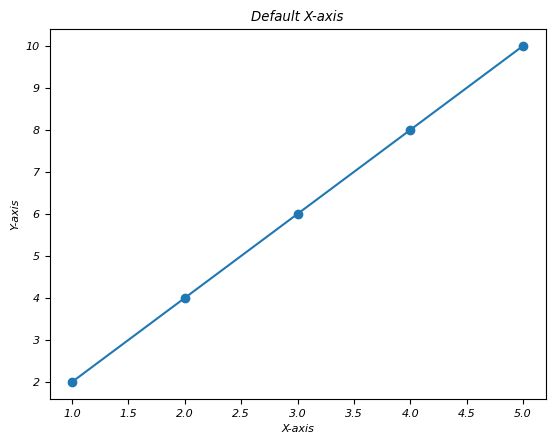

The second plot will display the same data as the first one but the x-axis will be reversed. Data points that were initially on the left side will now appear on the right side by altering the visual representation of the data.
Use Cases for Reversing the X-axis
Flipping Time Series Data − When we are plotting time series data then reversing the x-axis might better align with the chronological order.
Reorienting Geographical Plots − In some geographical plots reversing the x-axis could match the expected orientation or conventions.
Reversing the x-axis provides an alternative perspective for visualizing data allowing us to present information in a different order or orientation for clearer insights or better alignment with conventions.
This function reverses the direction of the x-axis by flipping the plot horizontally. Data points that were originally plotted from left to right will now be displayed from right to left.
Reversing Y-axis
The plt.gca().invert_yaxis() function reverses the direction of the y-axis by flipping the plot vertically. Data points that were originally plotted from bottom to top will now be displayed from top to bottom.
The reversing of Y - axis is also as same as the reversing the X - axis of a plot which we seen in the upper section. The below are the steps to be followed for reversing the Y - axis.
Create a Plot
Generate a plot with our data using Matplotlib.
Example
import matplotlib.pyplot as plt # Sample data x = [1, 2, 3, 4, 5] y = [2, 4, 6, 8, 10] # Plot with default axis orientation plt.plot(x, y, marker='o') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Default Y-axis') plt.show()
Output

Reverse the Y-axis
After creating the plot use plt.gca().invert_yaxis() to reverse the y-axis.
Example
import matplotlib.pyplot as plt # Sample data x = [1, 2, 3, 4, 5] y = [2, 4, 6, 8, 10] # Plot with default axis orientation plt.plot(x, y, marker='o') plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Default Y-axis') plt.show() # Reversing the x-axis # Reversing the y-axis plt.plot(x, y, marker='o') plt.gca().invert_yaxis() # Reverse y-axis plt.xlabel('X-axis') plt.ylabel('Reversed Y-axis') plt.title('Reversed Y-axis') plt.show()
Output

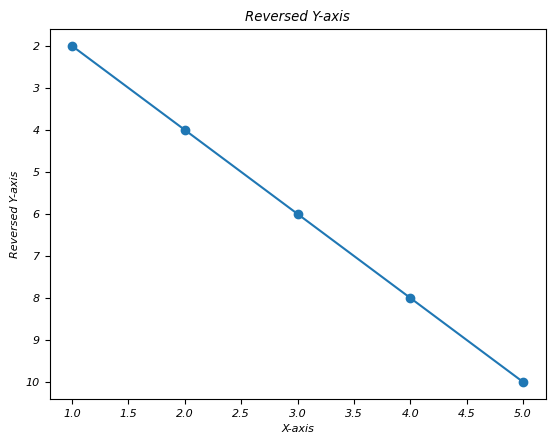