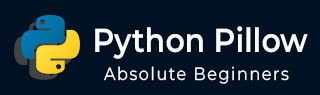
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow with Tkinter BitmapImage and PhotoImage objects
The ImageTk module in the python pillow library provides functionality to create and manipulate Tkinter BitmapImage and PhotoImage objects from PIL images. This tutorial discusses the descriptions of the key classes and methods within the ImageTk module.
These classes and methods provide convenient ways to work with Tkinter images using PIL in Python, allowing for integration of images into graphical user interfaces.
Tkinter BitmapImage class in Python Pillow
The ImageTk.BitmapImage class represents a Tkinter-compatible bitmap image that can be utilized wherever Tkinter expects an image object.
Here are the key details about the class −
The provided image must have a mode of "1".
Pixels with a value of 0 are considered transparent.
Additional options, if provided, are forwarded to Tkinter.
A commonly used option is foreground, allowing specification of the color for non-transparent parts.
Following is the syntax of the ImageTk.BitmapImage() class −
class PIL.ImageTk.BitmapImage(image=None, **kw)
Where,
image − A PIL image with mode "1". Pixels with value 0 are treated as transparent.
**kw − Additional options passed on to Tkinter. The most commonly used option is foreground, which specifies the color for the non-transparent parts.
Following are the list of methods provided by the BitmapImage class −
height() − Returns the height of the image in pixels.
width() − Returns the width of the image in pixels.
Example
Here is an example demonstrating creating Tkinter windows, loading images with PIL, and then using ImageTk.BitmapImage to create Tkinter-compatible image objects for display in a Tkinter Label.
from tkinter import * from PIL import ImageTk, Image # Create a Tkinter window root = Tk() # Create a sample image 1-bit mode image (black and white) pil_image = Image.new("1", (700, 300), color=1) # Create a BitmapImage from the PIL image bitmap_image = ImageTk.BitmapImage(pil_image, background='', foreground='gray') # Display the BitmapImage in a Tkinter Label label = Label(root, image=bitmap_image) label.pack() # Run the Tkinter event loop root.mainloop()
Output

The PhotoImage class in Python Pillow
The ImageTk.PhotoImage() class represents a Tkinter-compatible photo image in Pillow, it suitable for use wherever Tkinter expects an image object.
Here are the key details about the class −
If the image is in RGBA format, pixels with an alpha value of 0 are treated as transparent.
The constructor can be initialized with either a PIL image, or a mode and size. Alternatively, you have the option to use the file or data parameters to initialize the photo image object.
Here is the syntax of the ImageTk.PhotoImage() class −
class PIL.ImageTk.PhotoImage(image=None, size=None, **kw)
Where,
image − Represents either a PIL image or a mode string. If a mode string is used, a size must also be given.
size − If the first argument is a mode string, this defines the size of the image.
file − A filename to load the image from using Image.open(file).
data − An 8-bit string containing image data, as loaded from an image file.
Following are the list of methods provided by the PhotoImage() class −
height() − Get the height of the image in pixels.
width() − Get the width of the image in pixels.
paste(im) − Paste a PIL image into the photo image. Note that this can be slow if the photo image is displayed.
Parameters − im - A PIL image. The size must match the target region. If the mode does not match, the image is converted to the mode of the bitmap image.
Example
Here is an example demonstrating creating Tkinter windows, loading images with PIL, and then using ImageTk.PhotoImage to create Tkinter-compatible image objects for display in a Tkinter Label.
from tkinter import * from PIL import ImageTk, Image # Create a Tkinter window root = Tk() # Create a canvas widget canvas = Canvas(root, width=700, height=400, bg='white') canvas.grid(row=2, column=3) # Load an image using PIL and create a PhotoImage pil_image = Image.open("Images/pillow-logo-w.jpg") tk_image = ImageTk.PhotoImage(pil_image) # Draw the image on the canvas canvas.create_image(20, 20, anchor=NW, image=tk_image) # Start the Tkinter event loop mainloop()
Output
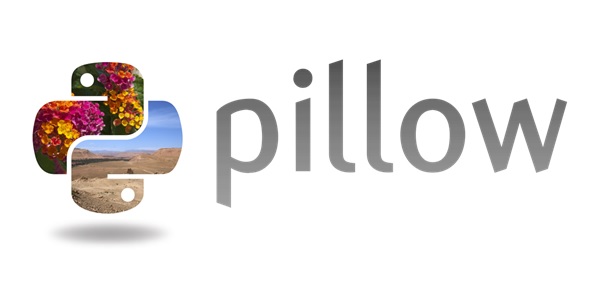