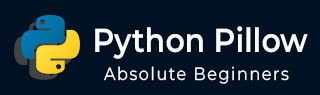
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow - Enhancing Contrast
Enhancing contrast refers to the process of improving the visibility and quality of an image. It is an image processing technique that involves increasing the difference between the various elements within an image, such as objects, shapes, edges, and textures, by adjusting the distribution of intensity values or colors. This technique is widely used in fields such as medical imaging, computer vision, remote sensing, and photography to improve image quality and obtain more detailed visual information.
The Python Pillow (PIL) library offers the Contrast() class within its ImageEnhance module, for the application of contrast enhancement to images.
Enhancing Contrast of an Image
To adjust the contrast of an image, you can apply ImageEnhance.Contrast() class to an image object with the enhancment factor. It controls the contrast of an image, much like adjusting the contrast on a television set.
Below is the syntax of the ImageEnhance.Contrast() class −
class PIL.ImageEnhance.Contrast(image)
Following are the steps to achieve contrast enhancement of an image −
Create a contrast object using the ImageEnhance.Contrast() class.
Then apply the enhancement factor with the help of contrast_object.enhance() method.
An enhancement factor is a floating-point value passed to the common single interface method, enhance(factor), which plays an important role in adjusting image contrast. When a factor of 0.0 is used, it will produce a solid grey image. A factor of 1.0 will give the original image, and when greater values are used, the contrast of the image is increased, making it visually more distinct.
Example
The following example demonstrates how to achieve a highly contrasted image using the PIL.ImageEnhance module.
from PIL import Image, ImageEnhance # Open the input image image = Image.open('Images/flowers.jpg') # Create an ImageEnhance object for adjusting contrast enhancer = ImageEnhance.Contrast(image) # Display the original image image.show() # Enhance the contrast by a factor of 2 and display the result enhancer.enhance(2).show()
Input Image
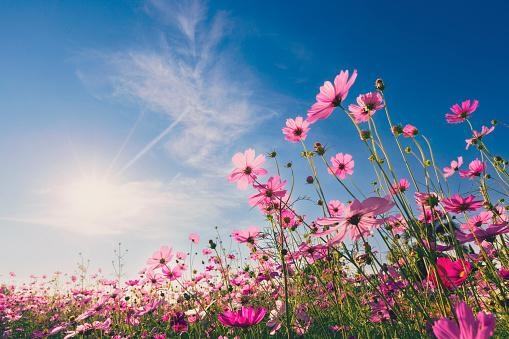
Output Image
Output a highly contrasted version of the input image −

Example
To reduce the contrast of an image, you can use a contrast enhancement factor less than 1. Here's an example illustrating the creation of a low-contrast version of the input image using the PIL.ImageEnhance.Contrast class.
from PIL import Image, ImageEnhance # Open the input image image = Image.open('Images/flowers.jpg') # Create an ImageEnhance object for adjusting contrast enhancer = ImageEnhance.Contrast(image) # Display the original image image.show() # Reduce the contrast by a factor of 0.5 and display the result enhancer.enhance(0.5).show()
Input Image
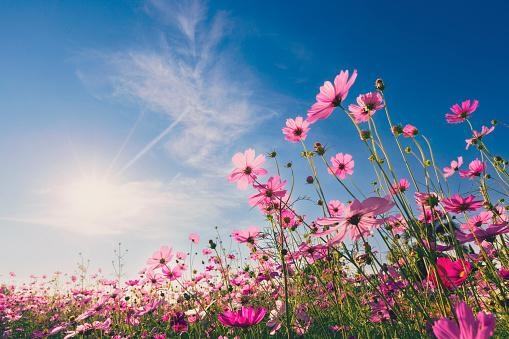
Output Image
Output a low contrasted version of the input image −
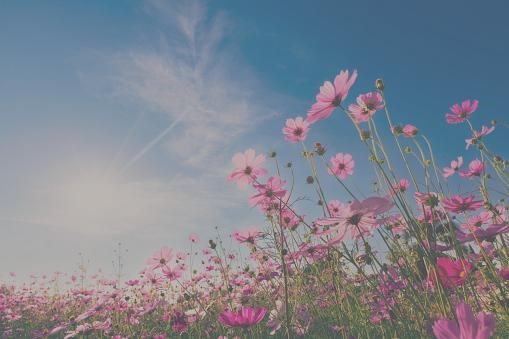