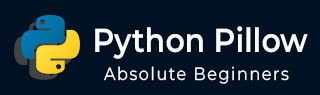
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow - Concatenating two Images
Concatenating two images with Pillow typically refers to combining or joining two separate images to create a single image either horizontally or vertically. This process allows us to merge the content of the two images into a larger image.
Pillow is a Python Imaging Library (PIL) which provides various methods and functions to perform image manipulation including image concatenation. When concatenating images we can choose to stack them on top of each other vertical concatenation or place them side by side horizontal concatenation.
There are no direct methods in pillow to concatenate the images but we can perform that by using the paste() method in python.
Here is a step-by-step guide on how to perform concatenation of two Images.
Import the necessary modules.
Load the two images that we want to concatenate.
Decide whether we want to concatenate the images horizontally or vertically.
Save the concatenated image to a file.
Optionally we can display the concatenated image. This step is useful for visualizing the result but it's not required.
Following is the input images used in all the examples of this chapter.
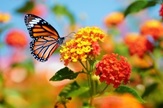
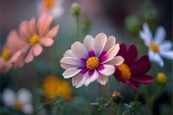
Example
In this example we are concatenating two input images horizontally.
from PIL import Image image1 = Image.open("Images/butterfly.jpg") image2 = Image.open("Images/flowers.jpg") result = Image.new("RGB", (image1.width + image2.width, image1.height)) result.paste(image1, (0, 0)) result.paste(image2, (image1.width, 0)) result.save("output Image/horizontal_concatenated_image.png") result.show()
Output

Example
Here in this example we are concatenating the given two input images vertically.
from PIL import Image image1 = Image.open("Images/butterfly.jpg") image2 = Image.open("Images/flowers.jpg") result = Image.new("RGB", (image1.width, image1.height + image2.height)) result.paste(image1, (0, 0)) result.paste(image2, (0, image1.height)) result.save("output Image/vertical_concatenated_image.png") result.show()
Output
