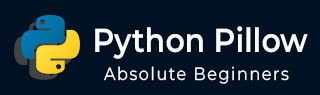
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow - Enhancing Color
Enhancing color is an image processing technique that focuses on improving the visual appeal and quality of a digital image by fine-tuning the balance and saturation of its colors. The objective of image color enhancement is to get finer image details while highlighting the useful information. This technique is especially useful in scenarios where images have poor illumination conditions, resulting in darker and low-contrast appearances that require refinement. This technique is widely used in various fields, including graphic design, photography, and digital art, to enhance the visual impact of images.
The Python Pillow library (PIL) offers the Color() class within its ImageEnhance module, which enables you to apply color enhancement to images.
Enhancing Color of an Image
To enhance the color of an image using the Python Pillow library, you can use the ImageEnhance.Color() class, which allows you to adjust the color balance of an image, just as controls on a color TV set.
Following is the syntax of the ImageEnhance.Color() class −
class PIL.ImageEnhance.Color(image)
An enhancement factor represented as a floating-point value, is passed as an argument to the common single interface method, enhance(factor). This factor plays an important role in adjusting the color balance. A factor of 0.0 will give a black and white image. A factor of 1.0 gives the original image.
Following are the steps to achieve color enhancement of an image −
Create a color enhancer object using the ImageEnhance.Color() class.
Then apply the enhancement factor to the enhancer object using the enhance() method.
Example
Here is an example that enhances the color of an image with a factor of 3.0.
from PIL import Image, ImageEnhance # Open an image file image = Image.open('Images/Tajmahal_2.jpg') # Create a Color object color_enhancer = ImageEnhance.Color(image) # Display the original image image.show() # Enhance the color with a factor of 3.0 colorful_image = color_enhancer.enhance(3.0) # Display the enhanced color image colorful_image.show()
Output
Input image −

Output highly color enhanced image −

Example
Here is an example that enhances the color of an image with a lower enhancement factor(0.0).
from PIL import Image, ImageEnhance # Open an image file image = Image.open('Images/Tajmahal_2.jpg') # Create a Color object color_enhancer = ImageEnhance.Color(image) # Display the original image image.show() # Enhance the color with a factor of 0.0 colorful_image = color_enhancer.enhance(0.0) # Display the enhanced color image colorful_image.show()
Output
Input image −

Output color enhanced image with a factor of 0.0 −
