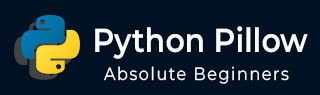
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow - Adding Borders to Images
Adding borders to images is one of the common task in image processing. Borders can help to frame the content, draw attention to specific areas, or add a decorative element. In Pillow (PIL) the expand() method of the ImageOps module is used to increase the dimensions of an image by adding a border or padding around it. This can be helpful for various purposes such as adding a border, creating a canvas of a specific size or resizing the image to fit a particular aspect ratio.
The expand() method
The expand() method is useful for adding borders to images and adjusting their dimensions while maintaining the aspect ratio. We can customize the size and border color to suit our specific requirements.
Here's the basic syntax for the expand() method −
PIL.Image.expand(size, fill=None)
Where,
size − A tuple specifying the new dimensions i.e. width and height for the expanded image.
fill (optional) − An optional color value to fill the border area. It should be specified as a color tuple (R, G, B) or an integer value representing the color.
Following is the input image used in all the examples of this chapter.
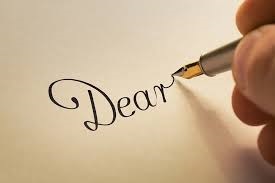
Example
In this example we are using the expand() method to create the expanded image with the border.
from PIL import Image, ImageOps #Open an image image = Image.open("Images/hand writing.jpg") #Define the new dimensions for the expanded image new_width = image.width + 40 #Add 40 pixels to the width new_height = image.height + 40 #Add 40 pixels to the height #Expand the image with a white border expanded_image = ImageOps.expand(image, border=20, fill="red") #Save or display the expanded image expanded_image.save("output Image/expanded_output.jpg") open_expand = Image.open("output Image/expanded_output.jpg") open_expand.show()
Output
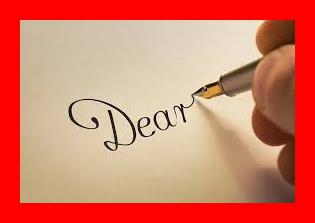
Example
Here this is another example we are expanding the image border with blue color by using the expand() method of the Image module.
from PIL import Image, ImageOps #Open an image image = Image.open("Images/hand writing.jpg") #Define the new dimensions for the expanded image new_width = image.width + 40 #Add 40 pixels to the width new_height = image.height + 40 #Add 40 pixels to the height #Expand the image with a white border expanded_image = ImageOps.expand(image, border=100, fill="blue") #Save or display the expanded image expanded_image.save("output Image/expanded_output.jpg") open_expand = Image.open("output Image/expanded_output.jpg") open_expand.show()
Output
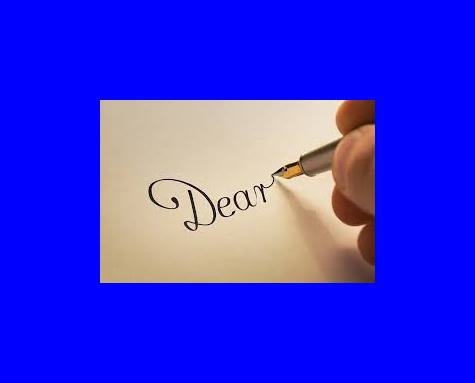