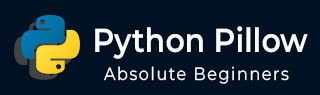
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow - Rolling an Image
Pillow (Python Imaging Library) allows us to roll or shift the image's pixel data within the image. This operation moves the pixel data either horizontally (left or right) or vertically (up or down) to create a rolling effect.
There is no direct method in pillow to roll or shift the image pixel data but it can be performed by using the copy and paste operations on the image. The below are the steps to be followed to perform rolling of the image.
Import the necessary modules.
Next load the image that we want to roll.
Define the rolling offset −
The rolling offset determines how much we want to shift the image. A positive value for the offset will shift the image right i.e. horizontal rolling or down i.e. vertical rolling and a negative value will shift the image left or up. We can choose the offset value based on our desired rolling effect.
Create a new image with the same size as the original. This new image will serve as the canvas for the rolled result.
Perform the rolling operations i.e. either horizontal rolling or vertical rolling.
Save the rolled image to a file.
Optionally display the rolled image. This step is useful for visualizing the result but it's not necessary.
Following is the input image used in all the examples of this chapter.
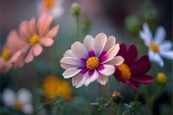
Example
In this example we are performing the horizontal rolling on the input image by specifying the horizontal offset as 50.
from PIL import Image image = Image.open("Images/flowers.jpg") horizontal_offset = 50 #Change this value as needed rolled_image = Image.new("RGB", image.size) for y in range(image.height): for x in range(image.width): new_x = (x + horizontal_offset) % image.width pixel = image.getpixel((x, y)) rolled_image.putpixel((new_x, y), pixel) rolled_image.save("output Image/horizontal_rolled_image.png") rolled_image.show()
Output

Example
In this example we are rolling the image vertically by specifying the offset value as 50.
from PIL import Image image = Image.open("Images/flowers.jpg") vertical_offset = 50 #Change this value as needed rolled_image = Image.new("RGB", image.size) for x in range(image.width): for y in range(image.height): new_y = (y + vertical_offset) % image.height pixel = image.getpixel((x, y)) rolled_image.putpixel((x, new_y), pixel) rolled_image.save("output Image/vertical_rolled_image.png") rolled_image.show()
Output
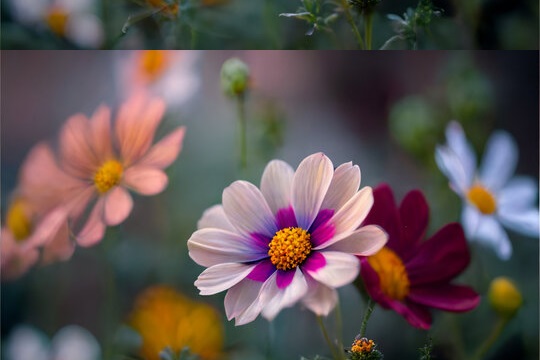