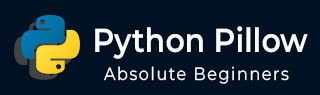
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow - Correcting Color Balance
Correcting color balance, in the context of image processing, refers to the adjustment of the intensities of different colors within an image to achieve a desired visual outcome. This adjustment typically involves the primary colors of red, green, and blue and is aimed at rendering specific colors, especially neutral colors like white or gray, correctly. Correct color balance ensures that the colors in an image appear as they would in the real world, making it look more naturalistic and pleasing to the human eye.
The Python pillow library provides several options to correct the color balance of an image. Let's explore them below.
Correcting Color Balance of an Image
Correcting or adjusting an image's color balance can simply be done by using the PIL.ImageEnhance.Color() class. This class provides the ability to fine-tune the color balance of an image by changing the enhancement factor.
Below is the syntax of the ImageEnhance.Color() class −
class PIL.ImageEnhance.Color(image)
An enhancement factor is a floating-point value passed to the common single interface method, enhance(factor), which plays an important role in adjusting image color balance. When a factor of 0.0 is used, it will produce a solid grey image. A factor of 1.0 will give the original image.
Following are the steps to adjust the color balance of an image −
- Create a color enhancer object using the ImageEnhance.Color() class.
- Then apply the enhancement factor using the enhancer_object.enhance() method.
Example
Here is an example that uses the PIL.ImageEnhance.Color class to adjust the color balance of an image.
from PIL import Image, ImageEnhance # Open the image input_image = Image.open("Images/Tajmahal_2.jpg") # Create a ColorEnhance object color_enhancer = ImageEnhance.Color(input_image) # Adjust the color balance by setting the enhancement factor enhancement_factor = 1.5 color_balanced_image = color_enhancer.enhance(enhancement_factor) # Display the original and color balanced images input_image.show() color_balanced_image.show()
Input Image

Output Color Balanced Image −

Correcting Color Balance using the Point Transforms
Here is another approach of applying point transforms to color channels of an image for adjusting the color balance of an image. Point transforms can generally be used to adjust various aspects of an image, including color balance, brightness, and contrast. This can be done by using the point() method, it helps to Process the individual bands/ channels of an image.
Example
Here is an example that demonstrates the application of point transforms to correct the color balance of an image.
from PIL import Image # Open the image input_image = Image.open("Images/tree.jpg") # Extract the red (R), green (G), and blue (B) channels red_channel, green_channel, blue_channel = input_image.split() # Adjust the color balance by scaling the intensity of each channel # Increase green intensity green_channel = green_channel.point(lambda i: i * 1.2) # Decrease blue intensity blue_channel = blue_channel.point(lambda i: i * 0.8) # Merge the channels back into an RGB image corrected_image = Image.merge("RGB", (red_channel, green_channel, blue_channel)) # Display the input and corrected images input_image.show() corrected_image.show()
Input Image
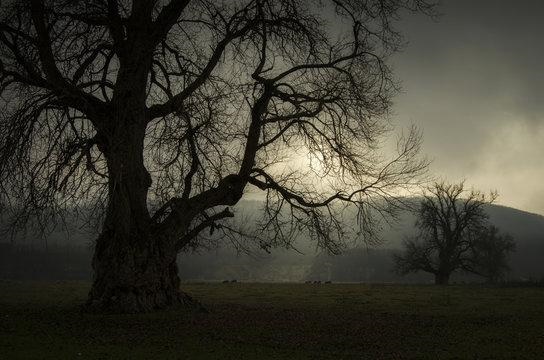
Output Image
Output Color Balanced Image −

Example
The following example adjusts the color balance of an image by applying a transformation matrix to its color channels.
from PIL import Image # Open the input image input_image = Image.open("Images/Tajmahal_2.jpg") # Define a transformation matrix to adjust color channels # Multiply R (red) by 1.2, leaving G (green) and B (blue) unchanged transformation_matrix = (1.5, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0) # Apply the color channel transformation and create the corrected image corrected_image = input_image.convert("RGB", transformation_matrix) # Show the original and corrected images input_image.show() corrected_image.show()
Input Image

Output Image
Output Color Balanced Image −
