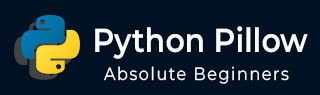
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow - ImageOps.equalize() Function
The PIL.ImageOps.equalize() function is used to equalize the histogram of an image, which means adjusting the intensity values of the pixels in the image so that the histogram becomes more uniform.
Syntax
Following is the syntax of the function −
PIL.ImageOps.equalize(image, mask=None)
Parameters
Here are the details of this function parameters −
image − The image to equalize. This is the input image on which the histogram equalization will be applied.
mask − An optional mask. If provided, only the pixels selected by the mask are included in the analysis. The mask is used to limit the equalization to specific regions of the image.
Return Value
An image − The function returns a new image object where the histogram has been equalized. The equalization process aims to create a uniform distribution of grayscale values in the output image.
Examples
Example 1
Here is an example of using the ImageOps.equalize() function to equalize the histogram of the input image.
from PIL import Image, ImageOps # Open an image file input_image = Image.open("Images/Car_2.jpg") # Apply histogram equalization equalized_image = ImageOps.equalize(input_image) # Display the original and equalized images input_image.show() equalized_image.show()
Output
Input Image
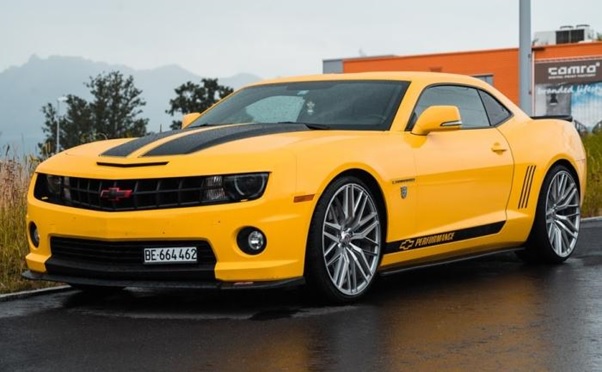
Output Image
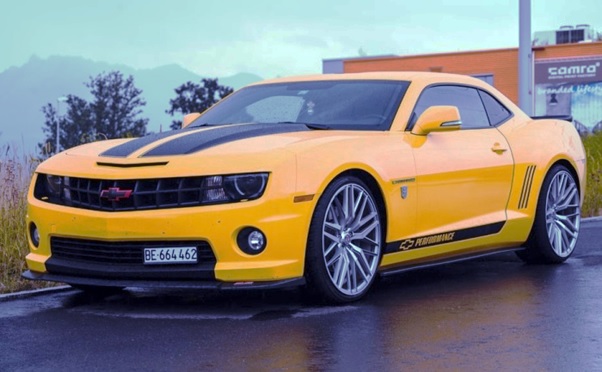
Example 2
This example demonstrates how to use histogram equalization with a mask to selectively enhance the contrast of the input image.
from PIL import Image, ImageOps, ImageDraw # Open an image file input_image = Image.open("Images/Car_2.jpg") # Define a mask mask = Image.new("L", input_image.size, 0) draw = ImageDraw.Draw(mask) draw.ellipse((140, 50, 260, 170), fill=255) # Apply histogram equalization with the mask equalized_image = ImageOps.equalize(input_image, mask) # Display the original and equalized images input_image.show() equalized_image.show()
Output
Input Image
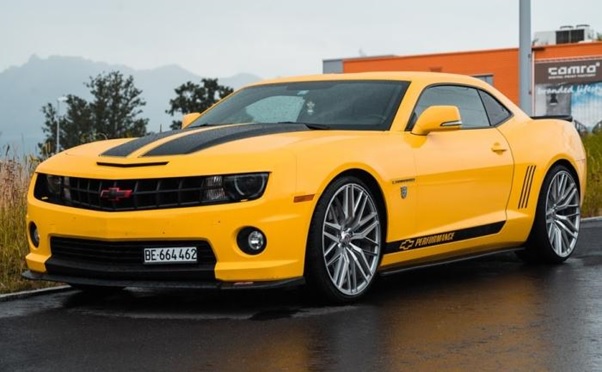
Output Image
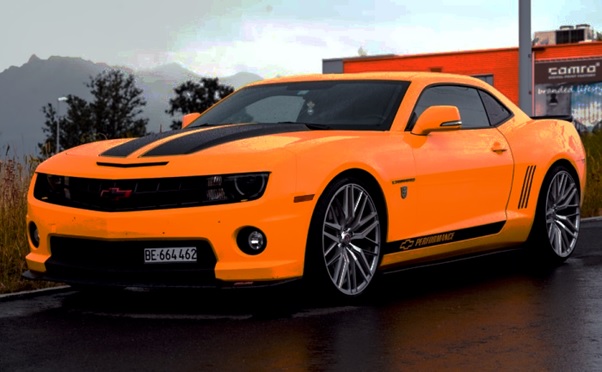
Example 3
Here's another example of using a rectangular mask to limit the histogram equalization of an input image.
from PIL import Image, ImageOps, ImageDraw # Open an image file input_image = Image.open("Images/Car_2.jpg") # Define a mask (you can create a mask using various techniques) mask = Image.new("L", input_image.size, 0) draw = ImageDraw.Draw(mask) draw.rectangle([(100, 100), (300, 300)], fill=255) # Apply histogram equalization with the mask equalized_image = ImageOps.equalize(input_image, mask=mask) # Display the original, and equalized image input_image.show() equalized_image.show()
Output
Input Image
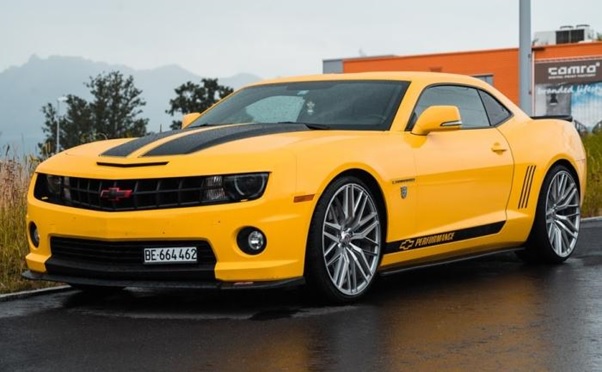
Output Image
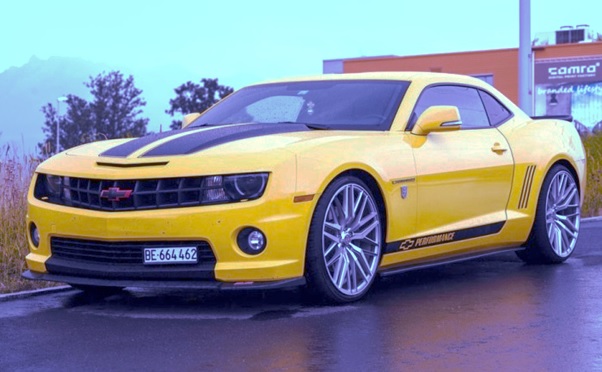