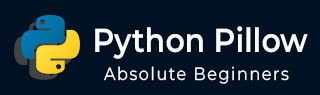
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow - ImageOps.scale() Function
The PIL.ImageOps.scale() function is used to obtain a rescaled image based on a specific factor provided as a parameter. The factor can be greater than 1 to expand the image or between 0 and 1 to contract the image.
Syntax
Following is the syntax of the function −
PIL.ImageOps.scale(image, factor, resample=Resampling.BICUBIC)
Parameters
Here are the details of this function parameters −
image − The image to be rescaled.
factor − The expansion or contraction factor, specified as a float.
resample − The resampling method to use, with the default being BICUBIC.
Return Value
The function returns an Image object representing the rescaled image.
Examples
Example 1
Here's an example that demonstrates how to use the ImageOps.scale function.
from PIL import Image, ImageOps # Open an image original_image = Image.open("Images/butterfly1.jpg") # Specify the rescaling factor rescale_factor = 1.1 # Apply the scale operation with BICUBIC resampling rescaled_image = ImageOps.scale(original_image, rescale_factor) # Save the original and rescaled images original_image.save('Function Reference\ImageOps Module\Scale Function\Ex1_Input Image.png') rescaled_image.save('Function Reference\ImageOps Module\Scale Function\Ex1_Output_Scale_FunC_result.png')
Output
If you visit the folder where the images are saved you can observe both the original and rescaled images as shown below −

Example 2
This example demonstrates process of contraction an image using the ImageOps.scale() function.
from PIL import Image, ImageOps # Open an image original_image = Image.open("Images/car.jpg") # Specify the rescaling factor # e.g., 0 for contraction, 1 for expansion rescale_factor = 0.5 # Apply the scale operation with BICUBIC resampling rescaled_image = ImageOps.scale(original_image, rescale_factor) # Save the original and rescaled images original_image.save('Function Reference\ImageOps Module\Scale Function\Ex2_Input Image.png') rescaled_image.save('Function Reference\ImageOps Module\Scale Function\Ex2_Output_Scale_FunC_result.png')
Output
If you visit the folder where the images are saved you can observe both the original and rescaled images as shown below −

Example 3
Here's another example demonstrating the use of PIL.ImageOps.scale to rescale an image with a different resampling method.
from PIL import Image, ImageOps, ImageFilter # Open an image original_image = Image.open("Images/butterfly.jpg") # Specify the rescaling factor rescale_factor = 1.5 # Apply the scale operation with LANCZOS resampling rescaled_image = ImageOps.scale(original_image, rescale_factor, resample=Image.Resampling.LANCZOS) # Save the original and rescaled images original_image.save('Function Reference\ImageOps Module\Scale Function\Ex3_Input Image.png') rescaled_image.save('Function Reference\ImageOps Module\Scale Function\Ex3_Output_Scale_FunC_result.png')
Output
If you visit the folder where the images are saved you can observe both the original and rescaled images as shown below −
