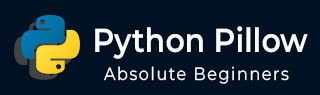
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow - ImageOps.grayscale() Function
The PIL.ImageOps.grayscale function is used to convert an image to grayscale. Grayscale is a single-channel representation of an image where each pixel's intensity is represented by a single value, typically ranging from 0 (black) to 255 (white).
Syntax
Following is the syntax of the function −
PIL.ImageOps.grayscale(image)
Parameters
Here are the details of this function parameters −
image − The image is to be converted to grayscale.
Return Value
The function returns a new image object where the input image has been converted to grayscale.
Examples
Example 1
In this example, ImageOps.grayscale() function is used to convert a color image to grayscale.
from PIL import Image, ImageOps # Open an image file color_image = Image.open("Images/Tajmahal_2.jpg") # Convert the image to grayscale grayscale_image = ImageOps.grayscale(color_image) # Display the original and grayscale images color_image.show() grayscale_image.show()
Output
Input Image
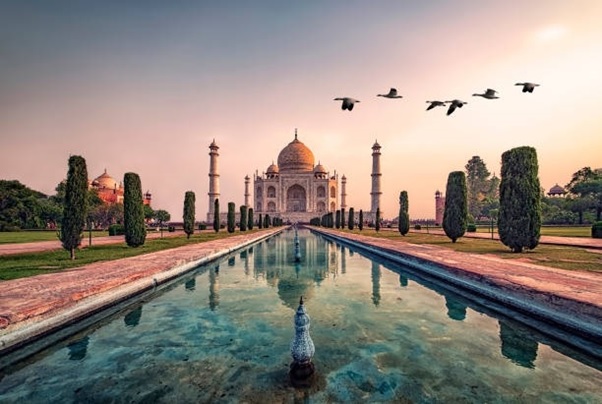
Output Image
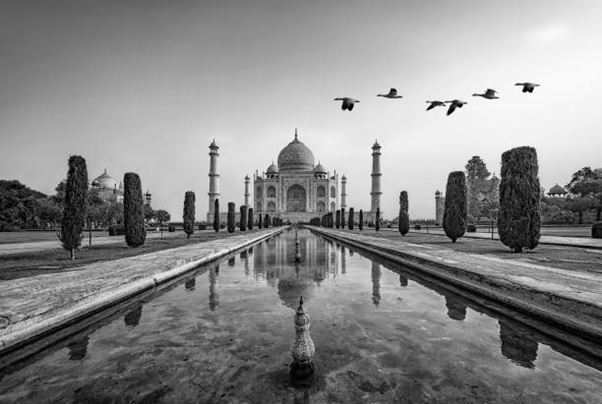
Example 2
Here's an example illustrating the use of the grayscale() function to convert a PNG image with RGBA channels to a grayscale image.
from PIL import Image, ImageOps # Open an image file color_image = Image.open("Images/pillow-logo-w.png") # Convert the image to grayscale grayscale_image = ImageOps.grayscale(color_image) # Display the original and grayscale images color_image.show() grayscale_image.show()
Output
Input Image
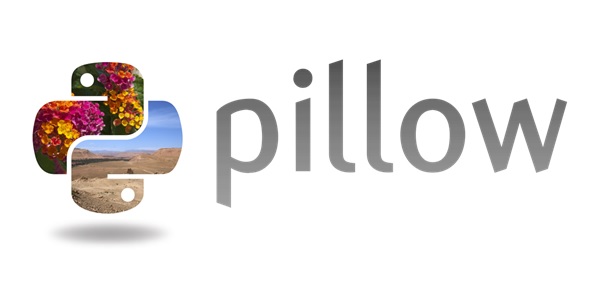
Output Image

Example 3
In this example, we open two images—one in RGB format and the other in RGBA format. Then we apply the ImageOps.grayscale() function to convert them to grayscale. Finally, we print the mode attribute for each image to determine the mode of each image.
The mode attribute will indicate "RGB" or "RGBA" for color images and "L" for grayscale images.
from PIL import Image, ImageOps import numpy as np # Open an RGB image file input_image = Image.open("Images/tutorialspoint2.jpg") # Open an RGBA image file image2 = Image.open('Images/pillow-logo-w.png') # Convert the images to grayscale grayscale_image = ImageOps.grayscale(input_image) grayscale_image2 = ImageOps.grayscale(image2) # Display the color mode of each image print("Input Image Mode:", input_image.mode) # Should print 'RGB' print("Grayscale Image Mode:", grayscale_image.mode) # Should print 'L' for grayscale print("Image2 Mode:", image2.mode) # Should print 'RGB' print("Grayscale Image2 Mode:", grayscale_image2.mode) # Should print 'L' for grayscale
Output
Input Image Mode: RGB Grayscale Image Mode: L Image2 Mode: RGBA Grayscale Image2 Mode: L