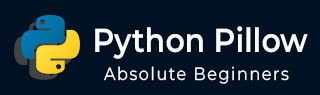
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow - ImageGrab.grabclipboard()Function
The PIL.ImageGrab.grabclipboard() function is used to take a snapshot of the clipboard image, if available.
On Linux, the function requires either wl-paste or xclip.
Syntax
Following is the syntax of the function −
PIL.ImageGrab.grabclipboard()
Return Value
The return value of this function varies depending on the Operation system. Here are the key details −
Windows − Returns an image, a list of filenames, or None if the clipboard does not contain image data or filenames. If a list is returned, the filenames may not necessarily represent image files.
Mac − Returns an image or None if the clipboard does not contain image data.
Linux − Returns an image.
Examples
Example 1
This code is a simple example of how to use the grabclipboard() method to fetch an image from the clipboard and display it.
from PIL import Image, ImageGrab # Use the grabclipboard method to capture the clipboard image clipboard_image = ImageGrab.grabclipboard() # Display the captured image clipboard_image.show()
Output
AttributeError: 'NoneType' object has no attribute 'show'
This error indicates that no image data was copied to the clipboard.
Example 2
To prevent the AttributeError and handle the case where grabclipboard() returns None, you can check if the result is not None before attempting to use the show() method.
from PIL import ImageGrab # Take a snapshot of the clipboard image clipboard_image = ImageGrab.grabclipboard() # Check clipboard_image object if clipboard_image: # Display or process the clipboard image as needed clipboard_image.show() else: print("The clipboard does not contain image data.")
Output
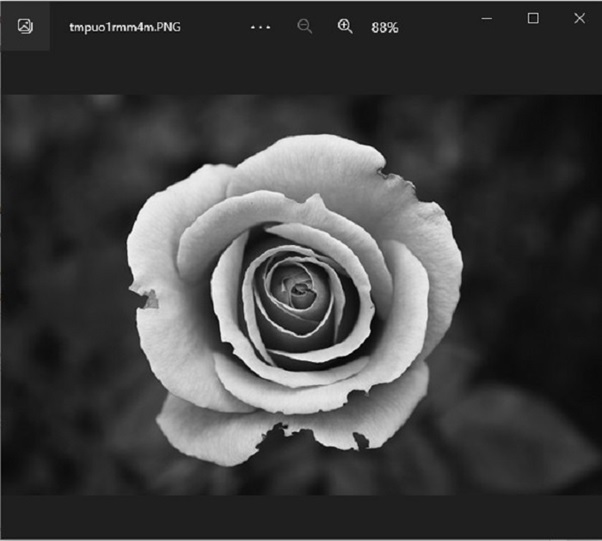
This example prevents the AttributeError and handles the case the clipboard contains non-image data, such as text.
Example 3
The example is similar to the previous one, but it explicitly checks whether the clipboard object is an image, instead of directly checking if the clipboard object is not None. This uses isinstance() function.
from PIL import ImageGrab # Take a snapshot of the clipboard image clipboard_image = ImageGrab.grabclipboard() # Check for the clipboard object is an image or not if isinstance(clipboard_image, Image.Image): # Display or save the clipboard image clipboard_image.show() else: print("The clipboard does not contain image data.")
Output
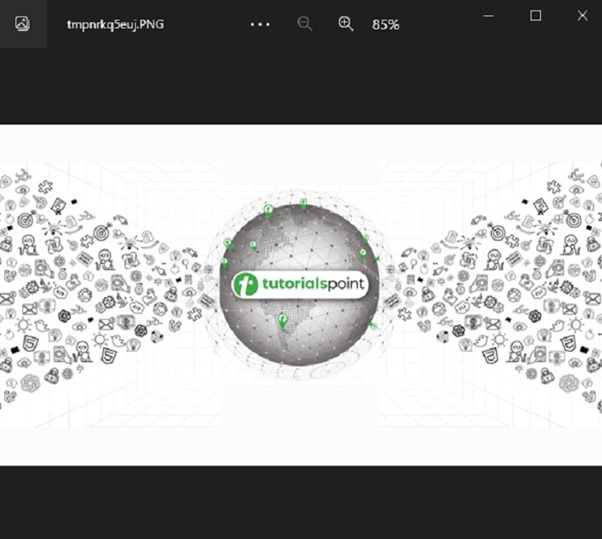