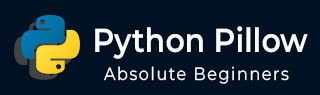
- Python Pillow Tutorial
- Python Pillow - Home
- Python Pillow - Overview
- Python Pillow - Environment Setup
- Basic Image Operations
- Python Pillow - Working with Images
- Python Pillow - Resizing an Image
- Python Pillow - Flip and Rotate Images
- Python Pillow - Cropping an Image
- Python Pillow - Adding Borders to Images
- Python Pillow - Identifying Image Files
- Python Pillow - Merging Images
- Python Pillow - Cutting and Pasting Images
- Python Pillow - Rolling an Image
- Python Pillow - Writing text on image
- Python Pillow - ImageDraw Module
- Python Pillow - Concatenating two Images
- Python Pillow - Creating Thumbnails
- Python Pillow - Creating a Watermark
- Python Pillow - Image Sequences
- Python Pillow Color Conversions
- Python Pillow - Colors on an Image
- Python Pillow - Creating Images With Colors
- Python Pillow - Converting Color String to RGB Color Values
- Python Pillow - Converting Color String to Grayscale Values
- Python Pillow - Change the Color by Changing the Pixel Values
- Image Manipulation
- Python Pillow - Reducing Noise
- Python Pillow - Changing Image Modes
- Python Pillow - Compositing Images
- Python Pillow - Working with Alpha Channels
- Python Pillow - Applying Perspective Transforms
- Image Filtering
- Python Pillow - Adding Filters to an Image
- Python Pillow - Convolution Filters
- Python Pillow - Blur an Image
- Python Pillow - Edge Detection
- Python Pillow - Embossing Images
- Python Pillow - Enhancing Edges
- Python Pillow - Unsharp Mask Filter
- Image Enhancement and Correction
- Python Pillow - Enhancing Contrast
- Python Pillow - Enhancing Sharpness
- Python Pillow - Enhancing Color
- Python Pillow - Correcting Color Balance
- Python Pillow - Removing Noise
- Image Analysis
- Python Pillow - Extracting Image Metadata
- Python Pillow - Identifying Colors
- Advanced Topics
- Python Pillow - Creating Animated GIFs
- Python Pillow - Batch Processing Images
- Python Pillow - Converting Image File Formats
- Python Pillow - Adding Padding to an Image
- Python Pillow - Color Inversion
- Python Pillow - M L with Numpy
- Python Pillow with Tkinter BitmapImage and PhotoImage objects
- Image Module
- Python Pillow - Image Blending
- Python Pillow Useful Resources
- Python Pillow - Quick Guide
- Python Pillow - Function Reference
- Python Pillow - Useful Resources
- Python Pillow - Discussion
Python Pillow - ImageChops.invert() Function
In the Python image processing library Pillow (PIL), the invert function, located in the ImageOps module, provides a convenient way to perform negative-positive inversion of pixel values in an image.
This PIL.ImageChops.invert function is used to invert the pixel values of an image or channel. It calculates the inverted values by subtracting each pixel value from the maximum possible pixel value. The operation is implemented as follows −
$$\mathrm{out\:=\:MAX\:−\:image}$$
Syntax
Following is the syntax of the function −
PIL.ImageChops.invert(image)
Parameters
Here are the details of this function parameters −
image − The input image whose pixel values will be inverted.
Return Value
The return type of this function is an Image.
Examples
Example 1
Here is an example that uses the ImageChops.invert() function on a JPEG image file to perform negative-positive inversion of pixel values in an image.
from PIL import Image, ImageChops # Open a PNG image file original_image = Image.open('Images/Car_2.jpg') # Invert the pixel values of the image inverted_image = ImageChops.invert(original_image) # Display the input and resulting images original_image.show() inverted_image.show()
Output
Input Image
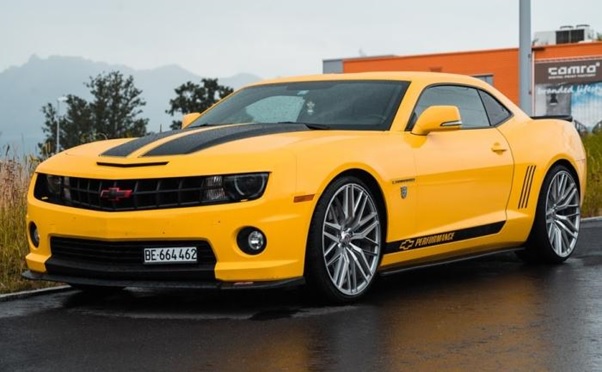
Output Image
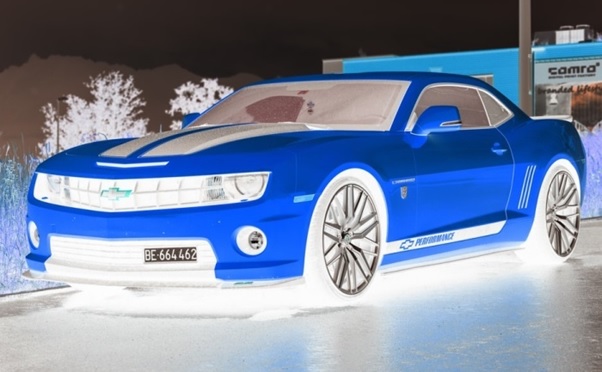
Example 2
Here's an example demonstrating the pixel values before and after applying ImageChops.invert() to the input image of type BMP.
from PIL import Image, ImageChops # Open an image file original_image = Image.open('Images/lena.bmp') # Display the pixel values before inversion print("Pixel values in the original image at (0, 0) before inversion:", original_image.getpixel((0, 0))) # Apply the ImageChops.invert() function to invert pixel values inverted_image = ImageChops.invert(original_image) # Display the pixel values after inversion print("Pixel values in the inverted image at (0, 0) after inversion:", inverted_image.getpixel((0, 0))) # Display the input and resulting images original_image.show() inverted_image.show()
Output
Input Image

Output Image
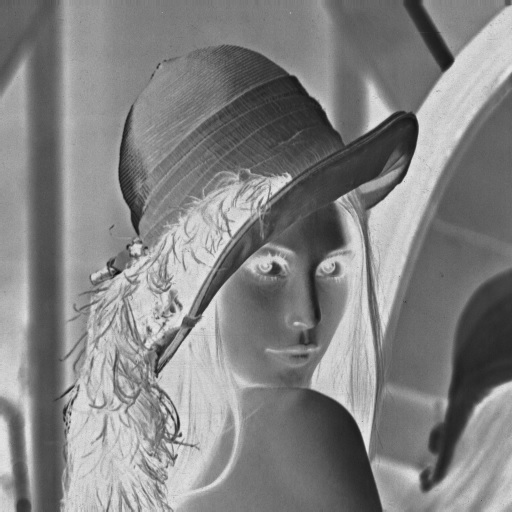
Example 3
The following example demonstrates how to apply the inversion operation on a single channel of an RGB image using the ImageChops.invert() function.
from PIL import Image, ImageChops # Open an image file original_image = Image.open('Images/flowers.jpg') # Split the channels of the image red, green, blue = original_image.split() # Invert the green channel inverted_green_channel = ImageChops.invert(green) # Merge the channels back into an RGB image image_with_inverted_green = Image.merge('RGB', (red, inverted_green_channel, blue)) # Display the input and resulting images original_image.show() image_with_inverted_green.show()
Output
Input Image
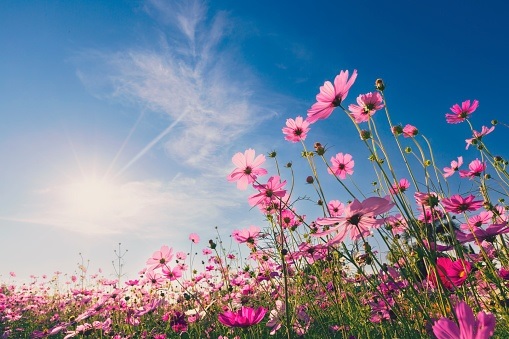
Output Image
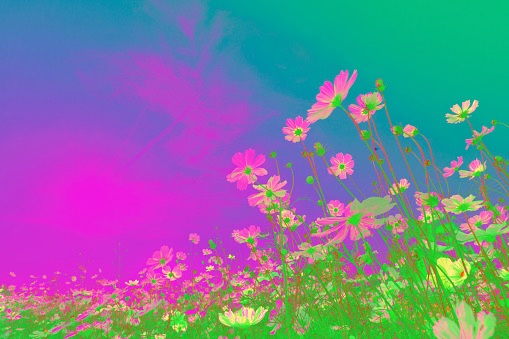