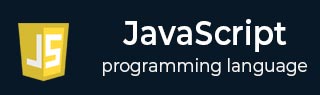
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Worker API
Web Worker API
The worker API is a web API that allows us to run JavaScript code in the background thread. Whenever the web page loads in the browser, it becomes interactive after every <script> tag loads in the browser. Web workers allows users to interact with the web page without loading the whole JavaScript code in the browser. It increases the response time of the web page.
Create a Web Worker File
To create a web worker, you need to write a script in the external file, which you need to execute in a different file.
The filename should have a '.js' extension.
In the below JavaScript code, we defined the counter() function. We used the setTimeout() method inside the function to call the counter() function after every 1000 milliseconds.
The important part of the code is the postMessage() method. It is used to send the data in the main thread.
function counter() { postMessage(data); // To send data to the main thread setTimeout("counter()", 1000); } counter();
Check for Web Worker Support
You should check that your browser supports the web worker or not before creating the web worker. You can use the typeof operator to check for this.
if (typeof(Worker) !== "undefined") { //"Web worker is supported by your browser!"; } else { //"Web worker is not supported by your browser!"; }
Create a Web Worker Object
After creating the external JavaScript file, you need to create a new Worker object by passing the path of the external JavaScript file as an argument, as shown below.
const workerObj = new Worker("testWorker.js");
To get the message main thread from the worker file, which we send using the postMessage() method, you can use the 'onmessage' event on the worker object, as shown below.
workerObj.onmessage = function(e) { // Use the event.data };
Terminate the Execution of the Web Worker
When you start the execution of the web worker script, it continues the execution until you terminate the execution.
You can use the terminate() method to terminate the execution of the web worker as shown below.
workerObj.terminate();
Example: Complete Program of Web Worker
Filename: - index.html
In the below code, we have defined the startWorker() and stopWorker() functions to start and stop the execution of the worker.
In the startWorker() function, first, we check whether the browser supports the workers. After that, we check whether any instance of the worker is running. If not, we create a new instance of the Worker object using the script defined in the external file.
After that, we added the ‘onmessage’ event on the worker object. So, whenever it gets data from the external script file, it prints it and performs other operations.
In the stopWorker() function, we use the terminate() method with the workerObj object to terminate the execution of the worker.
<html> <body> <button onclick = "startWorker()"> Start Counter </button> <button onclick = "stopWorker()"> Stop Counter </button> <div id = "output"></div> <script> let output = document.getElementById('output'); let workerObj; function startWorker() { if (typeof (Worker) !== "undefined") { if (typeof workerObj === "undefined") { workerObj = new Worker("app.js"); workerObj.onmessage = function (event) {//Getting the message from web worker output.innerHTML += "Event data is: " + event.data + "<br>"; }; } } else { output.innerHTML += "Web worker is not supported by your browser."; } } function stopWorker() { // To stop the web worker. if (typeof workerObj !== "undefined") { workerObj.terminate(); workerObj = undefined; } } </script> </body> </html>
Filename: - app.js
In the below code, we have defined the counter() function. In the counter() function, we used the setTimeOut() method to call the counter() function after every second. It also posts the data into the main thread using the postMessage() method.
var i = 0; function timedCount() { i = i + 1; postMessage(i); setTimeout("timedCount()",500); } timedCount();
Output
To run the above code, you need to make sure that the index.html and app.js file is on the live web server. You can also use the localhost. Also, make sure to add the correct path for the app.js file in the Worker object inside the index.html file.
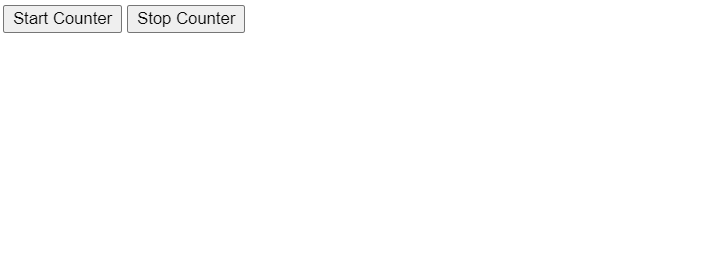
You can also use multiple workers in the same file to run multiple scripts in the background.
Web Worker Use Cases
The above example is simple, and in such cases, you don’t need to use web workers, but it is only for demonstrations.
Here are the real-time use cases of the web workers.
When you need to execute large or complex mathematical script
In the HTML games, you may use web workers
If you want to improve the website performance
In parallel downloads, when you need to execute multiple threads
For the background data synchronization
In the machine learning
For generating reports
To process audio and videos
Web Workers and the DOM
As you need to define the scripts for the web workers in the external file, you can't use the below objects in the external file.
The window object
The document object
The parent object
However, you can use the below objects in the web workers.
The location object
The navigator object
The Application Cache
Importing external script using importScripts()
XMLHttpRequest