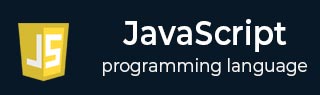
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Type Conversions
JavaScript Type Conversions
Type Conversions in JavaScript refer to the automatic or explicit process of converting data from one data type to another in JavaScript. These conversions are essential for JavaScript to perform operations and comparisons effectively. JavaScript variables can contain the values of any data type as it is a weakly typed language.
There are two types of type conversion in JavaScript −
- Implicit type conversion
- Explicit type conversion
The implicit type conversion is also known as coercion.
Implicit Type Conversion
When type conversion is done by JavaScript automatically, it is called implicit type conversion. For example, when we use the '+' operator with the string and number operands, JavaScript converts the number to a string and concatenates it with the string.
Here are some examples of the implicit type conversion.
Converting to String (Implicit conversion)
In this example, we used the '+' operator to implicitly convert different values to the string data type.
"100" + 24; // Converts 24 to string '100' + false; // Converts false boolean value to string "100" + null; // Converts null keyword to string
Please note that to convert a value to string using "+" operator, one operand should be string.
Let's try the example below, and check the output −
<html> <head> <title>Implicit conversion to string </title> </head> <body> <script> document.write("100" + 24 + "<br/>"); document.write('100' + false + "<br/>"); document.write("100" + null+ "<br/>"); document.write("100" + undefined+ "<br/>"); </script> </body> </html>
Converting to Number (Implicit conversion)
When you use the string values containing the digits with the arithmetic operators except for the '+' operator, it converts operands to numbers automatically and performs the arithmetic operation, which you can see in the example below.
Boolean values are also gets converted to a number.
'100' / 50; // Converts '100' to 100 '100' - '50'; // Converts '100' and '50' to 100 and 50 '100' * true; // Converts true to 1 '100' - false; // Converts false to 0 'tp' / 50 // converts 'tp' to NaN
Try the example below and check the output −
<html> <head> <title> Implicit conversion to Number </title> </head> <body> <script> document.write(('100' / 50) + "<br>"); document.write(('100' - '50') + "<br>"); document.write(('100' * true) + "<br>"); document.write(('100' - false) + "<br>"); document.write(('tp' / 50) + "<br>"); </script> </body> </html>
Converting to Boolean (Implicit conversion)
When you use the Nullish (!!) operator with any variable, it implicitly converts its value to the boolean value.
num = !!0; // !0 = true, !!0 = false num = !!1; // !1 = false, !!1 = true str = !!""; // !"" = true, !!"" = false str = !!"Hello"; // !"Hello" = false, !!"Hello" = true
Null to Number (Implicit conversion)
In JavaScript, the null represents the empty. So, null automatically gets converted to 0 when we use it as an operand of the arithmetic operator.
let num = 100 + null; // Converts null to 0 num = 100 * null; // Converts null to 0
Undefined with Number and Boolean (Implicit conversion)
Using the undefined with the 'number' or 'boolean' value always gives the NaN in the output. Here, NaN means not a number.
<html> <head> <title> Using undefined with a number and boolean value </title> </head> <body> <script> let num = 100 + undefined; // Prints NaN document.write("The value of the num is: " + num + "<br>"); num = false * undefined; // Prints NaN document.write("The value of the num is: " + num + "<br>"); </script> </body> </html>
Explicit Type Conversion
In many cases, programmers are required to convert the data type of the variable manually. It is called the explicit type conversion.
In JavaScript, you can use the constructor functions or built-in functions to convert the type of the variable.
Converting to String (Explicit conversion)
You can use the String() constructor to convert the numbers, boolean, or other data types into the string.
String(100); // number to string String(null); // null to string String(true); // boolean to string
Example
You can use the String() constructor function to convert a value to the string.You can also use typeof operator to check the type of the resultant value.
<html> <head> <title> Converting to string explicitly </title> </head> <body> <script> document.write(typeof String(100) + "<br/>"); document.write(typeof String(null)+ "<br/>"); document.write(typeof String(true) + "<br/>"); </script> </body> </html>
We can also use the toString() method of Number object to convert number to string.
const num = 100; num.toString() // converts 100 to '100'
Converting to Number (Explicit conversion)
You can use the Numer() constructor to convert a string into a number. We can also use unary plus (+) operator to convert a string to number.
Number('100'); // Converts '100' to 100 Number(false); // Converts false to 0 Number(null); // Converts null to 0 num = +"200"; // Using the unary operator
However, you can also use the below methods and variables to convert the string into numbers.
Sr.No. | Method / Operator | Description |
---|---|---|
1 | parseFloat() | To extract the floating point number from the string. |
2 | parseInt() | To extract the integer from the string. |
3 | + | It is an unary operator. |
Example
You can use the Number() constructor function or unary (+) operator to convert a string, boolean, or any other value to a number.
The Number() function also converts the exponential notation of a number to a decimal number.
<html> <head> <title> Converting to string explicitly </title> </head> <body> <script> document.write(Number("200") + "<br/>"); document.write(Number("1000e-2") + "<br/>"); document.write(Number(false) + "<br/>"); document.write(Number(null) + "<br/>"); document.write(Number(undefined) + "<br/>"); document.write(+"200" + "<br/>"); </script> </body> </html>
Converting to Boolean (Explicit conversion)
You can use the Boolean() constructor to convert the other data types into Boolean.
Boolean(100); // Converts number to boolean (true) Boolean(0); // 0 is falsy value (false) Boolean(""); // Empty string is falsy value (false) Boolean("Hi"); // Converts string to boolean (true) Boolean(null); // null is falsy value (false)
Example
You can use the Boolean() constructor to convert values to the Boolean. All false values like 0, empty string, null, undefined, etc., get converted to false and other values are converted to true.
<html> <head> <title> Converting to string explicitly </title> </head> <body> <script> document.write(Boolean(100) + "<br/>"); document.write(Boolean(0) + "<br/>"); document.write(Boolean("") + "<br/>"); document.write(Boolean("Hi") + "<br/>"); document.write(Boolean(null) + "<br/>"); </script> </body> </html>
Converting Date to String/Number
You can use the Date object's Number() constructor or getTime() method to convert the date string into the number. The numeric date represents the total number of milliseconds since 1st January 1970.
Follow the syntax below to convert the date into a number.
Number(date); OR date.getTime();
You can use the String() constructor or the toString() method to convert the date into a string.
Follow the syntax below to convert the date into the string.
String(date); OR date.toString();
Let's try to demonstrate this with the help of a program.
<html> <head> <title> Coverting date to string / number </title> </head> <body> <script> let date = new Date(); let numberDate = date.getTime(); document.write("The Numeric date is: " + numberDate + "<br/>"); let dateString = date.toString(); document.write("The string date is: " + dateString + "<br/>"); </script> </body> </html>
Conversion Table in JavaScript
In the below table, we have given the original values and their resultant value when we convert the original value to string, number, and boolean.
Value | String conversion | Number conversion | Boolean conversion |
---|---|---|---|
0 | "0" | 0 | false |
1 | "1" | 1 | true |
"1" | "1" | 1 | true |
"0" | "0" | 0 | true |
"" | "" | 0 | false |
"Hello" | "Hello" | NaN | true |
true | "true" | 1 | true |
false | "false" | 0 | false |
null | "null" | 0 | false |
undefined | "undefined" | NaN | false |
[50] | "50" | 50 | true |
[50, 100] | "[50, 100]" | NaN | true |