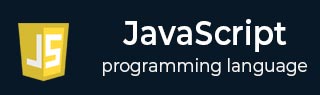
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - ECMAScript 2020
The ECMAScript 2020 version of JavaScript was released in 2020. Notable features added in this version include the nullish coalescing operator (??) for more concise default value assignment and dynamic import() for on-demand module loading. BigInt provides a way to safely work with very large integers. This chapter will discuss all the newly added features in ECMAScript 2020.
Features Added in ECMAScript 2020
Here are the new methods, features, etc., added to the ECMAScript 2020 version of JavaScript.
- BigInt
- Promise allSettled()
- String matchAll()
- The Nullish Coalescing Operator (??)
- The Optional Chaining Operator (?.)
Here, we have explained each feature in detail.
BigInt Primitive DataType
The ECMAScript 2020 introduced BigInt to the primitive data types. You can use the Big Int when you need to store a large number, which can’t be represented using the 64-bit representation.
To convert the number into the Big int, you can write the number followed by n.
Example
In the below code, we have defined the number of big int data type.
<html> <body> <div id = "output">The number of bigint type is: </div> <script> const bigNum = 1325461651565143545565n; document.getElementById("output").innerHTML += bigNum; </script> </body> </html>
Output
The number of bigint type is: 1325461651565143545565
The Nullish Coalescing Operator (??)
The JavaScript Nullish Coalescing operator returns the left operand if it is not undefined or null. Otherwise, it returns the right operand. It is used to set default values to the variables.
Example
In the below code, the left operand for the nullish coalescing operator is undefined. So, it returns the value of the right operand.
<html> <body> <div id = "output">The value of the str is: </div> <script> let str = undefined ?? "Hello"; document.getElementById("output").innerHTML += str; </script> </body> </html>
Output
The value of the str is: Hello
Promise allSettled() Method
The Promise.allSettled() method returns the status of all promises once all promises get fulfilled.
Example
In the code below, we have defined the array of promises.
After that, we used the promise.allSettled() method to fulfill all promises. In the output, you can see that method returns the array of objects, representing the status and result of each promise.
<html> <body> <div id = "output"> </div> <script> const promises = [ Promise.resolve("Hello"), Promise.reject("Error message"), Promise.resolve(21342) ]; Promise.allSettled(promises) .then(results => { document.getElementById("output").innerHTML += JSON.stringify(results); }); </script> </body> </html>
Output
[{"status":"fulfilled","value":"Hello"},{"status":"rejected","reason":"Error message"},{"status":"fulfilled","value":21342}]
The String matchAll() Method
The string matchAll() method matches all occurrences of the particular string substring. It takes the string or regular expression as a parameter.
Example
In the below code, we used the String.matchAll() method to match the ‘abcd’ substring in the str string. The method returns an iterator of all matching occurrences.
<html> <body> <div id = "output">The matching results are: <br></div> <script> let str = "Abcd abcd abcd"; let matches = str.matchAll('abcd'); for (let x of matches) { document.getElementById("output").innerHTML += x + "<br>" } </script> </body> </html>
Output
The matching results are: abcd abcd
The Optional Chaining Operator (?.)
The Optional chaining operator is used to access the nested object properties. If any nested object is undefined, it returns undefined rather than throwing an error.
Example
In the code below, the obj object contians the obj1 nested object, containing the name property.
We try to access the obj2 object’s name property with an optional chaining operator. Obj2 is not defined here, so it returns the undefined rather than throwing an error.
<html> <body> <div id = "output">The name of obj2 is: </div> <script> let obj = { obj1: { name: "JavaScript", } } document.getElementById("output").innerHTML += obj?.obj2?.name; </script> </body> </html>
Output
The name of obj2 is: undefined
Warning – Some of the above features are not supported by some browsers. So, you can use the polyfill to avoid errors.