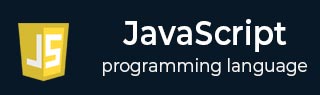
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - The TypedArray Object
What is a TypedArray?
A JavaScript TypedArray is an array-like object used to store binary data. Unlike the array, the size of the TypedArray can't be dynamic and can't hold the values of the different data types, improving the performance of the TypedArray.
A TypedArray is used in audio processing, graphics rendering, networking, etc.
Why TypedArray?
In other programming languages like C++, an array can contain data of only one data type, but a JavaScript array is a bit different. It can contain elements of multiple data types. So, JavaScript arrays are less efficient in dealing with binary data and when higher performance is needed.
It is one of the reasons that TypedArray is introduced in JavaScript, and it is also called the array buffer. A TypedArray is the best way to handle binary data while maintaining the memory.
TypedArray Objects
Here are the types of TypedArray objects available to store the data of the 8, 16, 32, or 64-bit data. You can choose any object to create a TypedArray according to the data you need to store.
Sr. No. | TypedArray | Data Type | Range | Example |
---|---|---|---|---|
1 | Int8Array |
8-bit two's complement Signed integer (byte) | -28 to 127 | new Int8Array([92, 17, -100]) |
2 | Uint8Array | 8-bit Unsigned integer | 0 to 255 | new Uint8Array([132, 210, 0]) |
3 | Uint8ClampedArray | 8-bit Unsigned integer | 0 to 255 | new Uint8ClampedArray([90, 17, 70]) |
4 | Int16Array |
Short integer | -32768 to 32767 | new Int16Array([1000, -2000, 150]) |
5 | Uint16Array | Unsigned short int | 0 to 65535 | new Uint16Array([50, -6535, 12000]) |
6 | Int32Array |
Signed long integer | -2147483648 to 2147483647 | new Int32Array([1000000, -2000000, 9876]) |
7 | Uint32Array | Unsigned long integer | 0 to 4294967295 | new Uint32Array([100, 42967295, 0]) |
8 | Float32Array | Float (7 significant digits) | ±1.2x10^-38 to ±3.4x10^38 | new Float32Array([3.134, 1.618, -0.5]) |
9 | Float64Array | Double (16 significant digits) | ±5.0x10^-324 to ±1.8x10^308 | new Float64Array([2.78, -12.35, 99.9]) |
10 | BigInt64Array | Big signed integer | -2^63 to 2^63 − 1 | new BigInt64Array([-9071954740991n, 9719925474991n]) |
11 | BigUint64Array | Big unsigned integer | 0 to 2^64 - 1 | new BigUint64Array([0n, 18446744709551615n]) |
A TypedArray doesn't support the methods like push(), pop, etc., but supported properties and methods are listed below.
TypedArray Properties
Here is a list of the properties of the TypedArray object along with their description −
Sr.No. | Property & Description |
---|---|
1 | Constructor It returns an array buffer constructor. |
2 | byteLength It returns the byte length of the TypedArray. |
3 | maxByteLength It returns the maximum byte length to expand the size of the TypedArray. |
4 | resizable To check whether the TypedArray is resizable. |
TypedArray Static Methods
Here is a list of the static methods of the TypedArray object along with their description −
Sr.No. | Methods | Description |
---|---|---|
1 | from() |
It returns a new Array instance. |
2 | It returns a new Array instance. |
TypedArray Instance Methods
Here is a list of the instance methods of the TypedArray object along with their description −
TypedArray instance methods
Sr.No. | Methods | Description |
---|---|---|
1 | It returns an element in the typed array that matches the given index. |
|
2 | It returns a modified TypedArray without changing the length of the original TypedArray. |
|
3 | It returns a new array iterable object. |
|
4 | It returns true if all elements in the typed array pass the test implemented by the callback function, and false otherwise. |
|
5 | It returns the modified typed array, that is filled with the specified value. |
|
6 | It returns a new copy of a typed array that includes only the elements that pass the test. |
|
7 | It returns the first element is TypedArray that satisfied the provided test function, 'undefined' otherwise. |
|
8 | It returns an index of the first element is TypedArray that satisfied the provided test function, '-1' otherwise. |
|
9 | It returns the last element in the typed array that satisfies the provided testing function, 'undefined' if not found. |
|
10 | It returns the last element in the typed array that passes the test, -1 if not found. |
|
11 | It returns none(undefined). |
|
12 | It returns 'true' if searchElement is found in the typed array; 'false' otherwise. |
|
13 | It returns the first index of the searchElement. |
|
14 | It returns a string by joining all the elements of a typed array. |
|
15 | It returns a new iterable iterator object. |
|
16 | It returns the last index of the searchElement in a typed array. If the search element is not present, it returns -1. |
|
17 | It returns a new typed array by executing the callback function on each element. |
|
18 | It returns a value that outputs from running the "reducer" callback function to completion over the entire typed array. |
|
19 | It returns a value that results from the reduction. |
|
20 | It returns the reference of the original typed array in reversed order. |
|
21 | It returns none(undefined). |
|
22 | It returns a new typed array containing the extracted elements of the original typed array. |
|
23 | It returns true, if atlesat one element in typed array pass the test implemented by provided function; false otherwise. |
|
24 | It returns the reference of the same typed array in sorted order. |
|
25 | It returns a new TypedArray object. |
|
26 | It returns a string that represents the elements of the typed array. |
|
27 | It returns a new typed array containing the elements in reversed order. |
|
28 | It returns a new typed array with the elements sorted in ascending order. |
|
29 | It returns a string that represents the elements of the typed array. |
|
30 | It returns a new array iterable object. |
|
31 | It returns a new typed array with the element at the index replaced with the specified value. |
Examples
Example 1
We used the Int8Array to create a TypedArray in the example below. We passed the array containing the multiple elements as an object constructor.
In the output, you can see that if any input element is greater than the 8-bit number, the constructor function automatically enforces it to the 8-bit number.
<html> <body> <p id = "output"> </p> <script> const array = new Int8Array([1000, 200, 30, 40]); document.getElementById("output").innerHTML = "The array is: " + array; </script> </body> </html>
Output
The array is: -24,-56,30,40
Example 2
In the example below, we used the Float32Array() constructor function to create a TypedArray. It is used to store the 32-bit floating point numbers.
Also, you can access or update TypedArray elements as the normal array.
<html> <body> <p id = "output"> </p> <script> const array = new Float32Array([100.212, 907.54, 90, 14562547356342.3454]); array[2] = 23.65; // Updating the 3rd element of the array document.getElementById("output").innerHTML = "The array is: " + array; </script> </body> </html>
Output
The array is: 100.21199798583984,907.5399780273438,23.649999618530273,14562546941952