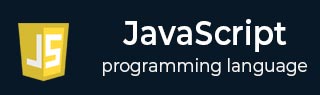
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - DOM Elements
The DOM Elements
The HTML DOM elements is the acronym for the Document Object Model elements. With JavaScript, we can manipulate the HTM elements. We can access the HTML elements using id, attribute, tag name, class name, etc.
When a web page loads in the browser, it creates a DOM tree representing the web page's structure. The web page contains various HTML elements, which you can manipulate using the properties and methods in JavaScript. Here we will discuss to access, modify, or replace, etc. DOM elements.
Accessing DOM elements
You can use different methods to access HTML elements, as given below.
Get HTML Element using its "id"
In the web page, each HTML element can have a unique id value. You can use the getElementById() method to access the HTML element using the id.
Syntax
Follow the syntax below to use the getElemenetById() method to access the HTML element using its id.
document.getElementById('id')
In the above syntax, you need to replace the 'id' with the actual id of the element.
Example
In the below code, we access the div element using id and change its inner HTML using the 'innerHTML' property.
<html> <body> <div id = "output"> </div> <script> document.getElementById('output').innerHTML = "The element is accessed using the id."; </script> </body> </html>
Output
The element is accessed using the id.
Get an HTML element using the "name"
An HTML can have a 'name' attribute. You can use the getElementByName() method to access the HTML element using the 'name' attribute's value.
Syntax
Follow the syntax below to use the getElementByName() method.
document.getElementsByName('name')
Here, you need to replace the 'name' with a value of the element's name attribute.
Example
In the below code, we access the div element using the name. It returns the array of nodes with the name passed as an argument.
<html> <body> <div name = "text"> Hello World! </div> <div id = "output">The content of the div elment is: </div> <script> const divEle = document.getElementsByName('text'); document.getElementById("output").innerHTML += divEle[0].innerHTML; </script> </body> </html>
Output
Hello World! The content of the div elment is: Hello World!
Get HTML element using the "className"
The class attribute of the HTML contains the string value. A single HTML element can also have multiple classes. You can use the getElementByClassName() method to access the HTML element using any single class name among multiples.
Syntax
Follow the syntax below to use the getElementByClassName() method.
document.getElementsByClassName('className');
In the above syntax, replace the 'className' with the value of the elment's 'class' attribute.
Example
In the below code, we access the div element using the class name.
<html> <body> <div class = "fruit"> Apple </div> <div id = "output">The name of the fruit is: </div> <script> const divEle = document.getElementsByClassName('fruit'); document.getElementById("output").innerHTML += divEle[0].innerHTML; </script> </body> </html>
Output
Apple The name of the fruit is: Apple
Get an HTML element using the "tag" name
Each HTML element is defined using the HTML tag. You can use the tag getElementByTagName() method to access the HTML elements using the tag name.
Syntax
Follow the syntax below to use the getElementByTagName() method.
document.getElementsByTagName('tag');
In the above syntax, replace the 'tag' with the HTML tag like 'p', 'a', 'img', etc.
Example
In the below code, we access the <p> elements using the getElementTagName() method.
It returns the Nodelist of <p> elements.
<html> <body> <p>This is the first paragraph.</p> <p>This is the second paragrraph.</p> <div id = "output"> </div> <script> const numbers = document.getElementsByTagName('p'); document.getElementById("output").innerHTML = "The first 'p' element is: " + numbers[0].innerHTML + "<br>" + "The second 'p' element is: " + numbers[1].innerHTML; </script> </body> </html>
Output
This is the first paragraph. This is the second paragrraph. The first 'p' element is: This is the first paragraph. The second 'p' element is: This is the second paragrraph.
Modifying the DOM Elements
JavaScript allows you to modify and replace the HTML DOM elements.
In this section, we will cover modifying DOM elements' content and replacing child elements.
Modify the Content of the DOM Element
You can use the 'textContent' property of the element to modify the text of the HTML element. However, you can use other properties like innerHTML, outerHTML, outerText, etc., to modify the HTML element's content.
Syntax
Follow the syntax below to use the textContent property to modify the text content of the HTML element.
element.textContent = newText;
In the above syntax, we have assigned the 'newText' dynamic value to the 'textContent' property to update the content of the 'element'.
Example
In the output, when you click the button, it will invoke the updateText() function and uses the textContent property to change the text of the div element.
<html> <body> <button onclick = "updateText()"> Update Text </button> <p id = "text"> Hello World! </p> <script> function updateText() { document.getElementById("text").textContent = "This is updaetd text!"; } </script> </body> </html>
Replace the Child Element
In JavaScript, you can use the replaceChild() method to replace the child element of the particular HTML element.
Syntax
Follow the syntax below to use the replaceChild() method in JavaScript.
textDiv.replaceChild(newNode,replacableNode);
The replaceChild() method takes a new node as a first parameter and a node that needs to be replaced as a second parameter.
Example
In the below code, we used the replaceChild() method to replace the second child of the div element with a new node. Here, we have also used the createTextNode() to create a new node with a 'Hello World!' text.
<html> <body> <button onclick = "replaceText()"> Replace Child </button> <div id = "text"> <p> Hi Users! </p> </div> <script> function replaceText() { let textDiv = document.getElementById("text"); let newText = document.createTextNode("Hello World"); textDiv.replaceChild(newText, textDiv.childNodes[1]); } </script> </body> </html>
Adding Events to the DOM Elements
You can use addEventListner() method to add events to the DOM elements to interact with the HTML elements.
Syntax
Follow the syntax below to use the addEventListner() method.
element.addEventListener(eventName, callback);
The addEventListner() method takes an event name as the first parameter and a callback function as a second parameter.
Example
We added the click event on the div element in the code below. The div element will print a message on the web page whenever you click it.
<html> <body> <div id = "text" style = "background-color: red;color:white"> <p> Some text </p> <p> Some other text </p> </div> <div id = "output"> </div> <script> let text = document.getElementById("text"); text.addEventListener("click", function () { document.getElementById("output").innerHTML = "You clicked on the div element"; }); </script> </body> </html>
List of DOM Element Properties
The table below contains the properties you can use with HTML elements in JavaScript.
Property | Description |
---|---|
accessKey | It is used to access or set the accessKey attribute of an HTML element. |
attributes | It returns all attributes of the element in the NamedNodeMap format. |
childElementCount | It returns the total number of children of the particular HTML element. |
childNodes | It is used to get the NodeList of the child nodes of a particular HTML element. |
children | It is used to get the HTML collection of child nodes of the HTML element. |
classList | It returns the array containing the class names of the HTML element. |
className | To get or set the value of the class attribute. |
clientHeight | To get the height of the HTML element. (It includes the padding) |
clientLeft | To get the width of the left border. |
clientTop | To get the width of the right border. |
clientWidth | To get the width of the HTML element. (It includes the padding) |
contentEditable | To set the boolean value, representing whether the content of the HTML element should be editable. |
dir | To set or get the direction of the text as ltr (left to right) or rtl (right to left). |
firstChild | To get the first child node of the element. |
firstElementChild | To get the first child element of the particular HTML element. |
id | To get or set the value of the 'id' attribute. |
innerHTML | To get or update the HTML of the element. |
innerText | To set or get the text content of the HTML element and its children. |
isContentEditable | It returns a Boolean value based on whether the content is editable. |
isDefaultNamespace() | It returns a boolean value based on the namespace URI, which is the same as default. |
lang | To set or get the value of the lang attribute. |
lastChild | To get the last child node of the HTML element. |
lastElementChild | To get the last child of the HTML element. |
namespaceURI | To get the namespaceURI of the tree. |
nextSibling | To get the next node at the same level in the DOM tree. |
nextElementSibling | To get the next HTML element at the same level in the DOM tree. |
nodeName | To get the name of the node. |
nodeType | To get the type of the node. |
nodeValue | To get or set the value of the node. |
offsetHeight | To get the height of the element. It includes padding, a border, and a scroll bar. |
offsetWidth | To get the width of the element. It includes padding, a border, and a scroll bar. |
offsetLeft | To get the horizontal offset position of the HTML element. |
offsetParent | To get the offset container of the HTML element. |
offsetTop | To get the vertical offset position of the HTML element. |
outerHTML | To set or get the outer HTML. It includes the start and end tags. |
outerText | To set or get the outer text of the HTML element. |
ownerDocument | To get the root element of the document. |
parentNode | To get the parent Node of the HTML element. |
parentElement | To get the parent element of the HTML element. |
previousSibling | To get the previous node at the same level in the DOM tree. |
previousElementSibling | To get the previous element at the same level in the DOM tree. |
scrollHeight | To get the scrollable height of the element, including the padding. |
scrollLeft | To get or set a number of pixels by that element content is scrolled horizontally. |
scrollTop | To get or set a number of pixels by that element content is scrolled vertically. |
scrollWidth | To get the scrollable width of the element, including the padding. |
style | To set or get the style of the element. |
tabIndex | To set or get the value of the tabIndex attribute's value. |
tagName | To get the tag name. |
textContent | To get the text of the HTML element. |
title | To set or get the title of the element. |
List of DOM Element Methods
The table below contains the method you can use with HTML elements to manipulate them in JavaScript.
Method | Description |
---|---|
addEventListener() | To add an event handler to the HTML element. |
appendChild() | To add a new child element at the last node of the HTML element. |
blur() | To remove focus from the HTML element. |
click() | To click on the element. |
cloneNode() | To clone an element. |
closest() | To find the closest element in the DOM tree based on the CSS selector. |
compareDocumentPosition() | To compare the document position of two HTML elements. |
contains() | To check whether the HTML node contains the particular node. |
focus() | To focus on the HTML element. |
getAttribute() | To get the particular attribute's value of the HTML element. |
getAttributeNode() | To get the attribute node. |
getBoundingClientRect() | To get the element's size and position relative to the viewport. |
getElementById() | To access HTML element by id. |
getElementByName() | To access HTML elements using its name. |
getElementsByClassName() | To get a collection of child HTML elements that match the class name. |
getElementsByTagName() | To get a collection of child HTML elements that match the tag name. |
hasAttribute() | To check whether the HTML element contains the particular attribute. |
hasAttributes() | To check whether the HTML element contains at least 1 attribute. |
hasChildNodes() | To check whether the HTML element has child nodes. |
insertAdjacentElement() | To add an HTML element at a position relative to an element. |
insertAdjacentHTML() | To insert HTML at a position relative to the element. |
insertAdjacentText() | Insert text at a position relative to the text. |
insertBefore() | To insert another child node before the existing child node. |
isEqualNode() | To check whether two nodes are equal. |
isSameNode() | To check whether the two elements are the same node. |
matches() | It returns a boolean value based on whether the element matches the CSS selector. |
querySelector() | To access the first element that matches the CSS selector. |
querySelectorAll() | To access all elements that match the CSS selector. |
remove() | It is used to remove the element from the DOM. |
removeAttribute() | It returns the particular attribute from the element. |
removeAttributeNode() | It removes the attribute node. |
removeChild() | It removes the child node from the element. |
removeEventListener() | It is used to remove the event listener from the element. |
replaceChild() | It is used to replace the particular child node. |
scrollIntoView() | It scrolls the element in the viewport or visible area of the browser. |
setAttribute() | To update the particular attribute's value. |
setAttributeNode() | To set or update the attribute node. |