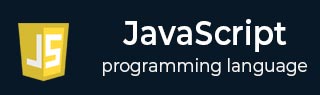
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - The Iterables Object
In JavaScript, iterables are objects that can be iterated through using the for...of loop. They can also be iterated over using other methods like forEach(), map(), etc.
Basically, you can traverse through each element of the iterable in JavaScript.
Here are some examples of the common iterables.
- Array
- String
- Map
- Set
- Arguments
- NodeList
- TypedArrays
- Generators
Iterate using the for...of loop
In this section, we will iterate through the array elements, string characters, set elements, and key-value pairs of the map using the for...of loop.
Example: Iterate over an array
In the below code, we have defined an array and used the for...of loop to iterate through the array.
The code prints each element of the array in the output.
<html> <body> <div>Iterating the array: </div> <div id = "demo"></div> <script> const output = document.getElementById("demo"); const array = ["Hello", "Hi", 10, 20, 30]; // Iterating array for (let ele of array) { output.innerHTML += ele + ", "; } </script> </body> </html>
Output
Iterating the array: Hello, Hi, 10, 20, 30,
Example: Iterate over a string
In the below code, we used the for...of loop to iterate through each string character.
The code prints the comma seprated string characters in the output.
<html> <body> <div id = "demo">Iterating a string: </div> <script> const output = document.getElementById("demo"); let str = "Hello"; // Iterating a string for (let char of str) { output.innerHTML += char + ", "; } </script> </body> </html>
Output
Iterating a string: H, e, l, l, o,
Example: Iterate over a set
In this example, we have created a set containing multiple elements. After that, we traverse through each element of the set and print in the output.
<html> <body> <p id = "demo">Iterating the Set: </p> <script> const output = document.getElementById("demo"); const set = new Set([10, 20, 30, 40, 40, 50, 50]); // Iterating a Set for (let ele of set) { output.innerHTML += ele + ", "; } </script> </body> </html>
Output
Iterating the Set: 10, 20, 30, 40, 50,
Example: Iterate over a map
In the example below, we have defined the map containing 3 key-value pairs. In each iteration of the for...of loop, we get a single key-value pair from the map.
<html> <body> <div id = "demo">Iterating the Map: <br></div> <script> const output = document.getElementById("demo"); const map = new Map([ ["1", "one"], ["2", "two"], ["3", "three"], ]); // Iterating array for (let ele of map) { output.innerHTML += ele + "<br>"; } </script> </body> </html>
Output
Iterating the Map: 1,one 2,two 3,three
Iterate using the forEach() method
In this section, we have used the forEach() method to iterate through the iterable.
Example
In the example below, we used the forEach() method with an array to iterate the array and print each element of the array in the output.
<html> <body> <div> Using the forEach() method: </div> <div id="demo"></div> <script> const output = document.getElementById("demo"); const array = [true, false, 50, 40, "Hi"]; // Iterating array array.forEach((ele) => { output.innerHTML += ele + ", "; }) </script> </body> </html>
Output
Using the forEach() method: true, false, 50, 40, Hi,
Iterate using the map() method
In this section, we have used the map() method to iterate through the iterable.
Example
In the example below, the map() method is used with the array. In the callback function of the map() method, we print the array elements.
<html> <body> <p id = "demo">Using the map() method: </p> <script> const output = document.getElementById("demo"); const array = [true, false, 50, 40, "Hi"]; // Iterating array array.map((ele) => { output.innerHTML += ele + ", "; }) </script> </body> </html>
Output
Using the map() method: true, false, 50, 40, Hi,
However, you can traverse the array, string, etc., using the loops like a for loop, while loop, etc. JavaScript also allows us to define custom iterators to traverse the iterable.