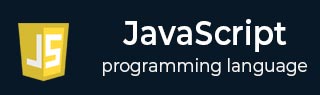
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Document Object
Window Document Object
The document object is a JavaScript object that provides the access to all elements of an HTML document. When the HTML document is loaded in the web browser, it creates a document object. It is the root of the HTML document.
The document object contains the various properties and methods you can use to get details about HTML elements and customize them.
The JavaScript document object is a property of the window object. It can be accessed using window.document syntax. It can also be accessed without prefixing window object.
JavaScript Document Object Properties
The JavaScript Document Object represents the entire HTML document, and it comes with several properties that allow us to interact with and manipulate the document. Some common Document object properties are as follows –
document.title − Gets or sets the title of the document.
document.URL − Returns the URL of the document.
document.body − Returns the <body> element of the document.
document.head − Returns the <head> element of the document.
document.documentElement − Returns the <html> element of the document.
document.doctype − Returns the Document Type Declaration (DTD) of the document.
These properties provide a way to access and modify different parts of the HTML document using JavaScript.
Example: Accessing the document title
In the example below, we use the document.title property to access the property odd the document.
<html> <head> <title> JavaScript - DOM Object </title> </head> <body> <div id = "output">The title of the document is: </div> <script> document.getElementById("output").innerHTML += document.title; </script> </body> </html>
Output
The title of the document is: JavaScript - DOM Object
Example: Accessing the document URL
In the example below, we have used the document.URL property to access the current URL of the page.
<html> <head> <title> JavaScript - DOM Object </title> </head> <body> <div id = "output">The URL of the document is: </div> <script> document.getElementById("output").innerHTML += document.URL; </script> </body> </html>
Output
The URL of the document is: https://www.tutorialspoint.com/javascript/javascript_document_object.htm
JavaScript Document Object Methods
The JavaScript Document Object provides us with various methods that allow us to interact with and manipulate the HTML document. Some common Document object methods are as follows –
getElementById(id) − Returns the element with the specified ID.
getElementsByClassName(className) − Returns a collection of elements with the specified class name.
getElementsByTagName(tagName) − Returns a collection of elements with the specified tag name.
createElement(tagName) − Creates a new HTML element with the specified tag name.
createTextNode(text) − Creates a new text node with the specified text.
appendChild(node) − Appends a node as the last child of a node.
removeChild(node) − Removes a child node from the DOM.
setAttribute(name, value) − Sets the value of an attribute on the specified element.
getAttribute(name) − Returns the value of the specified attribute on the element.
These methods enable us to dynamically manipulate the structure and content of the HTML document using JavaScript.
Example: Accessing HTML element using its id
In the example below, we use document.getElementById() method to access the DIV element with id "output" and then use the innerHTML property of the HTML element to display a message.
<html> <body> <div id = "result"> </div> <script> // accessing the DIV element. document.getElementById("result").innerHTML += "Hello User! You have accessed the DIV element using its id."; </script> </body> </html>
Output
Hello User! You have accessed the DIV element using its id.
Example: Adding an event to the document
In the example below, we use document.addEventListener() method to add a mouseover event to the document.
<html> <body> <div id = "result"> <h2> Mouseover Event </h2> <p> Hover over here to change background color </p> </div> <script> document.addEventListener('mouseover', function () { document.getElementById("result").innerHTML = "Mouseover event occurred."; }); </script> </body> </html>
Document Object Properties List
Here, we have listed all properties of the document object.
Property | Description |
---|---|
document.activeElement | To get the currently focused element in the HTML document. |
adoptedStyleSheets | It sets the array of the newly constructed stylesheets to the document. |
baseURI | To get the absolute base URI of the document. |
body | To set or get the document’s <body> tag. |
characterSet | To get the character encoding for the document. |
childElementCount | To get a count of the number of child elements of the document. |
children | To get all children of the document. |
compatMode | To get a boolean value representing whether the document is rendered in the standard modes. |
contentType | It returns the MIME type of the document. |
cookie | To get the cookies related to the document. |
currentScript | It returns the script of the document whose code is currently executing. |
defaultView | To get the window object associated with the document. |
designMode | To change the editability of the document. |
dir | To get the direction of the text of the document. |
doctype | To get the document type declaration. |
documentElement | To get the <html> element. |
documentURI | To set or get the location of the document. |
embeds | To get all embedded (<embed>) elements of the document. |
firstElementChild | To get the first child element of the document. |
forms | It returns an array of <form> elements of the document. |
fullScreenElement | To get the element that is being presented in the full screen. |
fullScreenEnabled | It returns the boolean value, indicating whether the full screen is enabled in the document. |
head | It returns the <head> tag of the document. |
hidden | It returns a boolean value, representing whether the document is considered hidden. |
images | It returns the collection of the <img> elements. |
lastElementChild | It returns the last child element of the document. |
lastModified | To get the last modified date and time of the document. |
links | To get the collection of all <a> and <area> elements. |
location | To get the location of the document. |
readyState | To get the current state of the document. |
referrer | To get the URL of the document, which has opened the current document. |
scripts | To get the collection of all <script> elements in the document. |
scrollingElement | To get the reference to the element which scrolls the document. |
styleSheets | It returns the style sheet list of the CSSStyleSheet object. |
timeLine | It represents the default timeline of the document. |
title | To set or get the title of the document. |
URL | To get the full URL of the HTML document. |
visibilityState | It returns the boolean value, representing the visibility status of the document. |
Document Object Methods List
The following is a list of all JavaScript document object methods −
Method | Description |
---|---|
addEventListener() | It is used to add an event listener to the document. |
adoptNode() | It is used to adopt the node from the other documents. |
append() | It appends the new node or HTML after the last child node of the document. |
caretPositionFromPoint() | It returns the caretPosition object, containing the DOM node based on the coordinates passed as an argument. |
close() | It closes the output stream opened using the document.open() method. |
createAttribute() | It creates a new attribute node. |
createAttributeNS() | It creates a new attribute node with the particular namespace URI. |
createComment() | It creates a new comment node with a specific text message. |
createDocumentFragment() | It creates a DocumentFragment node. |
createElement() | It creates a new element node to insert into the web page. |
createElementNS() | It is used to create a new element node with a particular namespace URI. |
createEvent() | It creates a new event node. |
createTextNode() | It creates a new text node. |
elementFromPoint() | It accesses the element from the specified coordinates. |
elementsFromPoint() | It returns the array of elements that are at the specified coordinates. |
getAnimations() | It returns the array of all animations applied on the document. |
getElementById() | It accesses the HTML element using the id. |
getElementsByClassName() | It accesses the HTML element using the class name. |
getElementsByName() | It accesses the HTML element using the name. |
getElementsByTagName() | It accesses the HTML element using the tag name. |
hasFocus() | It returns the boolean value based on whether any element or document itself is in the focus. |
importNode() | It is used to import the node from another document. |
normalize() | It removes the text nodes, which are empty, and joins other nodes. |
open() | It is used to open a new output stream. |
prepand() | It is used to insert the particular node before all nodes. |
querySelector() | It is used to select the first element that matches the css selector passed as an argument. |
querySelectorAll() | It returns the nodelist of the HTML elements, which matches the multiple CSS selectors. |
removeEventListener() | It is used to remove the event listener from the document. |
replaceChildren() | It replaces the children nodes of the document. |
write() | It is used to write text, HTML, etc., into the document. |
writeln() | It is similar to the write() method but writes each statement in the new line. |