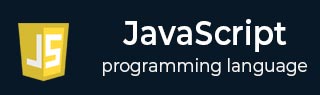
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Event Capturing
Event Capturing
Event capturing in JavaScript is the initial phase in the event propagation model where the event travels from the root of the document tree down to the target element. During this phase, the browser captures the event on the ancestors of the target element before reaching the target itself.
Event capturing and event bubbling are two phases in the JavaScript event propagation model. In event capturing, the browser captures and triggers events starting from the root of the document hierarchy and moving down to the target element. On the other hand, event bubbling occurs after the event reaches the target element and then bubbles up from the target to the root.
Capturing is useful for handling events at higher-level ancestors, while bubbling is the default behaviour where events propagate from the target back up the hierarchy. Understanding both phases is crucial for effective event handling and manipulation of the event flow.
Let us see some important aspects of event capturing.
Aspect | Description |
---|---|
Phase | Event capturing is the initial phase in the event propagation model. |
Direction | Capturing occurs in the reverse order of the element hierarchy, from the root of the document tree down to the target element. |
Use Case | Useful when you want to intercept and handle events at a higher-level ancestor before they reach the target element or trigger any bubbling phase handlers. |
Registration | Register event listeners for the capturing phase using the third parameter of addEventListener (e.g., element.addEventListener('click', myFunction, true);). |
Propagation | Automatic propagation that precedes the target and bubbling phases. The event flows down the hierarchy, triggering capturing phase handlers on each ancestor. |
Preventing Default | Use event.preventDefault() during the capturing phase to prevent the default behavior of the event before it reaches the target. |
Stopping Propagation | Use event.stopPropagation() in the capturing phase to stop further propagation of the event, preventing it from reaching the target or triggering bubbling phase handlers. |
Example: Basic Event Capturing
In this example, we have a container div (container) and a button (myButton) inside it. Two capturing phase event listeners are added using addEventListener with the true parameter to enable capturing. When you click the button, both capturing phase log messages (Container clicked and Button clicked) will be displayed. This illustrates the capturing phase as the event travels from the document root down to the target element.
<!DOCTYPE html> <html> <body> <div id="container"> <button id="myButton">Click me!</button> </div> <div id = "output"></div> <script> const output = document.getElementById('output'); document.getElementById('container').addEventListener('click', function(event) { output.innerHTML += 'Capturing phase - Container clicked' + "<br>"; }, true); document.getElementById('myButton').addEventListener('click', function(event) { output.innerHTML += 'Capturing phase - Button clicked' + "<br>"; }, true); </script> </body> </html>
Example: Preventing Default Behaviour
In this example, we have a hyperlink (<a>) with an id of "link". The capturing phase event listener is attached to the link during the capturing phase. When you click the link, the capturing phase log message (Link clicked) will be displayed, and the default behaviour (navigating to a new page) is prevented using event.preventDefault().
If the. preventDefault() was not used, it would have navigated the page to https://www.tutorialspoint.com.
<!DOCTYPE html> <html> <body> <a href="https://www.tutorialspoint.com" id="link">Click me to prevent default</a> <script> document.getElementById('link').addEventListener('click', function(event) { alert('Capturing phase - Link clicked'); event.preventDefault(); // Prevent the default behavior (e.g., navigating to a new page) }, true); </script> </body> </html>
Example: Capturing and Stopping Propagation
In this example, the parent div's capturing phase event listener actively halts propagation through the use of 'event.stopPropagation()'. Only upon clicking the button will you see a log message during capture phase ("Parent clicked") being displayed; however, it does not trigger any further action within child elements' capturing phases. This indeed showcases an effective method to arrest additional propagation in this particular instance.
<!DOCTYPE html> <html> <body> <div id="parent"> <button id="child">Click me!</button> </div> <div id = "output"></div> <script> const output = document.getElementById('output'); document.getElementById('parent').addEventListener('click', function(event) { output.innerHTML += 'Capturing phase - Parent clicked' + "<br>"; // Stop further propagation to the child element event.stopPropagation(); }, true); document.getElementById('child').addEventListener('click', function(event) { output.innerHTML += 'Capturing phase - Child clicked' + "<br>"; }, true); </script> </body> </html>