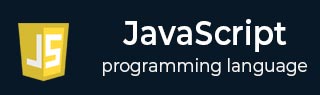
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Smart Function Parameters
The concept of smart function parameters in JavaScript is a way to make a function adaptable to different use cases. It allows the function to handle the different kinds of arguments passed to it while invoking it.
In JavaScript, the function is an important concept for reusing the code. In many situations, we need to ensure the function is flexible to handle different use cases.
Here are different ways to define a function with smart parameters.
Default Function Parameters
In JavaScript, the use of default function parameters is a way to handle the undefined argument values or arguments that haven't passed to the function during invocation of the function.
In the below code snippet, we set default values of the parameters, a and b to 100 and 50.
function division (a = 100, b = 50) { // Function body }
Example
In the below code, the division() function returns the division of the parameters a and b. The default value of parameter a is 100, and parameter b is 50 whenever you want to pass any argument or pass an undefined argument, parameters with initialized with their default value which you can observe from the values printed in the output.
<html> <head> <title> JavaScript - Default parameters </title> </head> <body> <p id = "output"> </p> <script> function division(a = 100, b = 50) { return a / b; } document.getElementById("output").innerHTML = division(10, 2) + "<br>" + division(10, undefined) + "<br>" + division(); </script> </body> </html>
Output
5 0.2 2
JavaScript Rest Parameter
When the number of arguments that need to pass to the function is not fixed, you can use the rest parameters. The JavaScript rest parameters allow us to collect all the reaming (rest) arguments in a single array. The rest parameter is represented with three dots (...) followed by a parameter. Here this parameter works as the array inside the function.
Syntax
Follow the below syntax to use the rest parameters in the function declaration.
function funcName(p1, p2, ...args) { // Function Body; }In the above syntax, 'args' is rest parameter, and all remaining arguments will be stored in the array named args.
Example
In the example below, the sum() function returns the sum of all values passed as an argument. We can pass an unknown number of arguments to the sum() function. The function definition will collect all arguments in the 'nums' array. After that, we can traverse the 'nums' array in the function body and calculate the sum of all argument values.
The sum() function will also handle the 0 arguments also.
<html> <head> <title> JavaScript - Rest parameters </title> </head> <body> <p id = "demo"> </p> <script> function sum(...nums) { let totalSum = 0; for (let p = 0; p < nums.length; p++) { totalSum += nums[p]; } return totalSum; } document.getElementById("demo").innerHTML = sum(1, 5, 8, 20, 23, 45) + "<br>" + sum(10, 20, 30) + "<br>" + sum() + "<br>"; </script> </body> </html>
Output
102 60 0
Note – You should always use the rest parameter as a last parameter.
JavaScript Destructuring or Named parameters
You can pass the single object as a function argument and destructuring the object in the function definition to get only the required values from the object. It is also called the named parameters, as we get parameters based on the named properties of the object.
Syntax
Follow the below syntax to use the destructuring parameters with the function.
function funcName({ prop1, prop2, ... }) { }
In the above syntax, prop1 and prop2 are properties of the object passed as an argument of the function funcName.
Example
In the example below, we have defined the 'watch' object containing three properties and passed it to the printWatch() function.
The printWatch() function destructuring the object passed as an argument and takes the 'brand' and 'price' properties as a parameter. In this way, you can ignore the arguments in the function parameter which are not necessary.
<html> <head> <title> JavaScript - Parameter Destructuring </title> </head> <body> <p id = "output"> </p> <script> function printWatch({ brand, price }) { return "The price of the " + brand + "\'s watch is " + price; } const watch = { brand: "Titan", price: 10000, currency: "INR", } document.getElementById("output").innerHTML = printWatch(watch); </script> </body> </html>
Output
The price of the Titan's watch is 10000
The above three concepts give us flexibility to pass the function arguments.