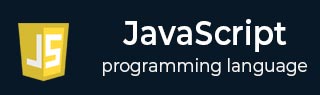
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Constants
JavaScript Constants
JavaScript constants are the variables whose values remain unchanged throughout the execution of the program. You can declare constants using the const keyword.
The const keyword is introduced in the ES6 version of JavaScript with the let keyword. The const keyword is used to define the variables having constant reference. A variable defined with const can't be redeclared, reassigned. The const declaration have block as well as function scope.
Declaring JavaScript Constants
You always need to assign a value at the time of declaration if the variable is declared using the const keyword.
const x = 10; // Correct Way
In any case, you can't declare the variables with the const keyword without initialization.
const y; // Incorrect way y = 20;
Can't be Reassigned
You can't update the value of the variables declared with the const keyword.
const y = 20; y = 40; // This is not possible
Block Scope
A JavaScript variable declared with const keyword has block-scope. This means same variable is treated as different outside the blcok.
In the below example, the x declared within block is different from x declared outside the blcok. So we can redeclare the same variable outsite the block
{ const x = "john"; } const x = "Doe"
But we can't redeclare the const varaible within the same block.
{ const x = "john"; const x = "Doe" // incorrect }
Constant Arrays and Objects in JavaScript
We can declare the arrays and objects using the const keyword, but there is a little twist in the array and object declaration.
The variable with the const keyword keeps the constant reference but not the constant value. So, you can update the same array or object declared with the const keyword, but you can't reassign the reference of the new array or object to the constant variable.
Example (Constant Arrays)
In the example below, we have defined the array named 'arr' using the const keyword. After that, we update the array element at the 0th index and insert the 'fence' string at the end of the array.
In the output, you can observe that it prints the updated array.
<html> <head> <title> Consant Arrays </title> </head> <body> <script> // Defining the constant array const arr = ["door", "window", "roof", "wall"]; // Updating arr[0] arr[0] = "gate"; // Inserting an element to the array arr.push("fence"); //arr = ["table", "chair"] // reassiging array will cause error. // Printing the array document.write(arr); </script> </body> </html>
When you execute the above code, it will produce the following result −
gate,window,roof,wall,fence
Example (Constant Objects)
In the below example, we created the 'obj' object with the const keyword. Next, we update the 'animal' property of the object and insert the 'legs' property in the object. In the output, the code prints the updated object.
<html> <head> <title> Constant Objects </title> </head> <body> <script> // Defining the constant object const obj = { animal: "Lion", color: "Yellow", }; // Changing animal name obj.animal = "Tiger"; // Inserting legs property obj.legs = 4; // Printing the object document.write(JSON.stringify(obj)); // obj = { name: "cow" } // This is not possible </script> </body> </html>
It will produce the following result −
{"animal":"Tiger","color":"Yellow","legs":4}
So, we can't change the reference to the variables (arrays and objects) declared with the const keyword but update the elements and properties.
No Const Hoisting
Varaibles defined with const keyword are not hoisted at the top of the code.
In the example below, the const variable x is accessed before it defined. It will cause an error. We can catch the error using try-catch statement.
<html> <body> <script> document.write(x); const x = 10; </script> </body> </html>
Here are some other properties of the variables declared with the const keyword.
- Block scope.
- It can't be redeclared in the same scope.
- Variables declared with the const keyword can't be hoisted at the top of the code.
- Constant variables value is a primitive value.
Difference between var, let and const
We have given the comparison table between the variables declared with the var, let, and const keywords.
Comparison basis | var | let | const |
---|---|---|---|
Scope | Function | Block | Block |
Hoisted | Yes | No | No |
Reassign | Yes | Yes | No |
Redeclare | Yes | No | No |
Bind This | Yes | No | No |
Which should you use among var, let, and const?
- For the block scope, you should use the let keyword.
- If you need to assign the constant reference to any value, use the const keyword.
- When you require to define the variable inside any particular block, like a loop, 'if statement', etc. and need to access outside the block but inside the function, you may use the var keyword.
- However, you can use any keyword to define global variables.
- Redeclaring variables is not a good practice. So, you should avoid it, but if necessary, you may use the var keyword.