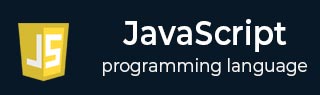
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript Number parseInt() Method
The JavaScript Number parseInt() method is used to convert a string into an integer according to a specified 'radix'. The Radix parameter represents the base in a mathematical numeral system, and must be an integer within the range of 2 to 36, inclusive. If the input string is invalid, or if the specified radix is outside of this range, the method returns 'NaN'.
Please find the important points listed below −
- If the input string has any leading whitespace, plus or minus signs, they will be removed from the string while converting, and if the string starts with "0x" or "0X", the radix is assumed to be 16, and the rest of the string will be treated as a hexadecimal number and converted accordingly.
- If the passed string begins with any other value, the radix(base) is 10 (decimal).
Syntax
Following is the syntax of JavaScript Number parseInt() method −
parseInt(string, radix)
Parameters
This method accepts two parameters: a "string" and an optional "radix", which are described below −
- string − The string start with an intger to be parsed.
- radix (optional) − It is an integer represents base in mathematical numeral system.
Return value
This method returns an integer value from the passed string.
Example 1
The following example demonstrates the usage of the JavaScript number parseInt() method.
<html> <head> <title>JavaScript parseInt() Method</title> </head> <body> <script> let val = " 10f "; document.write("Given value = " , val) document.write("<br>Integer value = ", Number.parseInt(val)); </script> </body> </html>
Output
Once the above program is executed, it will return an inetger value '10' −
Given value = 10f Integer value = 10
Example 2
If the passed radix value is not in the range of [2, 36], it will return 'NaN' in the output.
<html> <head> <title>JavaScript parseInt() Method</title> </head> <body> <script> let val = "10fcs"; let radix = 40; document.write("Given value = ", val); document.write("<br>Radix = ", radix); document.write("<br>Integer value = ", Number.parseInt(val, radix)); </script> </body> </html>
Output
If we execute the above program, it returns 'NaN'.
Given value = 10fcs Radix = 40 Integer value = NaN
Example 3
If a string starts with 'white spaces' and possibly 'plus' or 'minus' signs, they will be removed while converting to an integer value, and in this case, the radix is assumed to be 16.
<html> <head> <title>JavaScript parseInt() Method</title> </head> <body> <script> let val = " +23abc12"; let radix = 16; document.write("Given value = ", val); document.write("<br>Radix = ", radix); document.write("<br>Integer value = ", Number.parseInt(val, radix)); </script> </body> </html>
Output
The above program returns an integer value "37403666" for input string " +23abc12" −
Given value = +23abc12 Radix = 16 Integer value = 37403666
Example 4
Let's test what happens if the input string doesn't start with a valid number, but the radix is within the range of [2, 36].
<html> <head> <title>JavaScript parseInt() Method</title> </head> <body> <script> let val = "abc123"; let radix = 8; document.write("Given value = ", val) document.write("<br>Radix = ", radix); document.write("<br>Integer value = ", Number.parseInt(val, radix)); </script> </body> </html>
Output
If the input string is invalid, it will return 'NaN' in the output as −
Given value = abc123 Radix = 8 Integer value = NaN