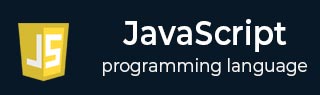
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript Number isNAN() Method
The JavaScript Number isNaN() method is used to determine whether a given number is a "NaN" or not. The "NaN" stands for 'Not a Number'. If you are familiar with this acronym, you have an idea about the purpose of this method. The method returns a boolean value 'true', if the given value is not a number, and 'false' otherwise.
isNan() vs Number.isNan()
The isNaN() method returns true; if the value is not a number, whereas the Number.isNaN() returns true; if the number is not a number(NaN).
Note: The isNaN() method converts the value to the number; if neccessary.
Syntax
Following is the syntax of JavaScript Number isNaN() method −
Number.isNaN(value)
Parameters
This method accepts a parameter named 'value'. The same is described below −
value − The value to be checked.
Return value
This method returns 'true'; if the value is not a number (NaN); 'false' otherwise.
Example 1
If the passed value is a number, this method returns 'false'.
In the following example, we are passing the '1234' value as an argument to the isNaN() method to check whether it is a number or not.
<html> <head> <title>JavaScript isNAN() Method</title> </head> <body> <script> let value = 1234; document.write("Given value = ", value); document.write("<br>Value is 'NaN' or not? ", Number.isNaN(value));//return false </script> </body> </html>
Output
The above program returns 'false'.
Given value = 1234 Value is 'NaN' or not? false
Example 2
Here's another example of the JavaScript Number isNaN() method. We are passing 'NaN' as an argument to this method to determine whether the value 'NaN' is Not a number. Since it is not a number, it will return true.
<html> <head> <title>JavaScript isNAN() Method</title> </head> <body> <script> let value = NaN; document.write("Given value = ", value); document.write("<br>Value is 'NaN' or not? ", Number.isNaN(value));//return true </script> </body> </html>
Output
Once the above program is executed, it will return 'true'.
Given value = NaN Value is 'NaN' or not? true
Example 3
Let's compare the results of passing the same value to both 'isNaN()' and 'Number.isNaN()' methods.
<html> <head> <title>JavaScript isNAN() Method</title> </head> <body> <script> let value = 'Hello'; document.write("Given value = ", value); document.write("<br>The 'isNaN()' method returns: ", isNaN(value));//returns true document.write("<br>The 'Nunber.isNaN()' method returns: ", Number.isNaN(value));// returns false </script> </body> </html>
Output
The above program produces the following output −
Given value = Hello The 'isNaN()' method returns: true The 'Nunber.isNaN()' method returns: false
Example 4
Let's see the real-time usage of this method. We will use the method's result within the conditional statement to print the inner statement based on the result produced by this method.
<html> <head> <title>JavaScript isNAN() Method</title> </head> <body> <script> function verify(value){ if(Number.isNaN(value)){ return true; } else{ return false; } } let val1 = NaN; let val2 = 122; let val3 = 'abc'; document.write("'", val1, "' is (not a number) = ", verify(val1)); document.write("<br>'", val2, "' is (not a number) = ", verify(val2)) document.write("<br>'", val3, "' is (not a number) = ", verify(val3)); </script> </body> </html>
Output
After executing the above program, it displays the following statements −
'NaN' is (not a number) = true '122' is (not a number) = false 'abc' is (not a number) = false