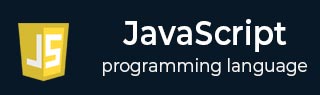
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript DataView getUint32() Method
The JavaScript DataView getUint32() method is used to read 4 bytes data starting at the specified byte offset of this data view and interprets them as a 32-bit unsigned integer. If we do not pass any parameter to this method, it will return the stored value automatically.
If the byteOffset parameter values are passed correctly, and the littleEndian parameter value passes anything other than 0, it will always return the maximum possible value of this data view.
It will throw a 'RangeError' exception if the byteOffset parameter value is outside the bounds of this data view.
Syntax
Following is the syntax of the JavaScript DataView getUint32() method −
getUint32(byteOffset, littleEndian)
Parameters
This method accepts two parameters named "byteOffset" and "littleEndian", which are described below −
- byteOffset − The position in the DataView from which to read the data.
- littleEndian (optional) − It indicates whether the data is stored in little-endian or big-endian format.
Return value
This method returns an integer from 0 to 4294967295, inclusive.
Example 1
The following is the basic example of the JavaScript DataView getUint32() method.
<html> <body> <script> //creating array buffer const buffer = new ArrayBuffer(16); const data_view = new DataView(buffer); const byteOffset = 1; const value = 230; document.write("The byte offset: ", byteOffset); document.write("<br>The data value: ", value); //lets set the data using the setUnit32() method data_view.setUint32(1, 230); //using the getUnit32() method document.write("<br>The data_view.getUnit32(1) method returns: ", data_view.getUint32(1)); </script> </body> </html>
Output
The above program reads the data and returns as −
The byte offset: 1 The data value: 230 The data_view.getUnit32(1) method returns: 230
Example 2
If we do not pass any parameter to this method, it will automatically return the stored value.
The following is another example of the JavaScript DataView getUint32() method. We use this method to read the 4-byte data 4294967295 stored by the setUnit32() method at the specified byte offset 0 of this data view.
<html> <body> <script> //creating array buffer const buffer = new ArrayBuffer(16); const data_view = new DataView(buffer); const byteOffset = 0; const value = 4294967295; document.write("The byte offset: ", byteOffset); document.write("<br>The data value: ", value); //lets set the data using the setUnit32() method data_view.setUint32(byteOffset, value); //using the getUnit32() method try { document.write("<br>The data_view.getUnit32() method returns: ", data_view.getUint32()); } catch (error) { document.write("<br>", error); } </script> </body> </html>
Output
After executing the above program, it will return the stored value as −
The byte offset: 0 The data value: 4294967295 The data_view.getUnit32() method returns: 4294967295
Example 3
If the byteOffset parameter value is outside of the bounds of this data view, it will throw a 'RangeError' exception.
<html> <body> <script> //creating array buffer const buffer = new ArrayBuffer(16); const data_view = new DataView(buffer); const byteOffset = 1; const value = 255; document.write("The byte offset: ", byteOffset); document.write("<br>The data value: ", value); //lets set the data using the setUnit32() method data_view.setUint32(byteOffset, value); //using the getUnit32() method try { document.write("<br>The data_view.getUnit32(-1) method returns: ", data_view.getUint32(-1)); } catch (error) { document.write("<br>", error); } </script> </body> </html>
Ouput
Once the above program is executed, it will throw a 'RangeError' exception.
The byte offset: 1 The data value: 255 RangeError: Offset is outside the bounds of the DataView
Example 4
If you pass anything other than 0 to the littleEndian parameter, this method will always return the maximum value of this data view (which is 4294967295). However, with the correct byte offset and a littleEndian parameter set to 0, it will return the stored value.
<html> <body> <script> //creating array buffer const buffer = new ArrayBuffer(16); const data_view = new DataView(buffer); const byteOffset = 1; const value = 255; const littleEndian1 = 0; const littleEndian2 = 1; document.write("The byte offset: ", byteOffset); document.write("<br>The data value: ", value); //lets set the data using the setUnit32() method data_view.setUint32(byteOffset, value); //using the getUnit32() method document.write("<br>The littleEndian parameter with a value of 0: ", data_view.getUint32(byteOffset, littleEndian1)); document.write("<br>The littleEndian parameter with a value of 1: ", data_view.getUint32(byteOffset, littleEndian2)); </script> </body> </html>
Output
The above program returns stored value and the default maximum value of this data view as −
The byte offset: 1 The data value: 255 The littleEndian parameter with a value of 0: 255 The littleEndian parameter with a value of 1: 4278190080