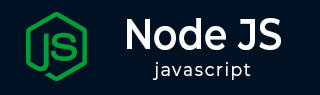
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Buffer Module
A Buffer is a space in computer memory that is temporarily used to store binary data. The Node.js Buffer module takes care of dealing with streams of binary data. The binary data is the data that the computer understands. It is labeled with a combination of 0's and 1's as per the binary numeral system. For example: 110011, 001110 etc.
In Node.js the buffer module is available globally. You don't have to use require to import it.
Class properties
Following are the list of properties −
Sr.No | Module & Description |
---|---|
1 |
It will give you an object of array buffer and the buffer created from it will be the same as the array buffer. |
2 |
It will give you an byteOffset value of the given buffer. |
3 |
It will give you the no of bytes in the buffer. |
4 |
It allocates the size in bytes towards Buffer instances which are later used by NodeJs for pooling. |
5 |
It helps to set or return the octet value at the index position in a buffer. |
Static Methods
Following are the list of methods −
Sr.No | Module & Description |
---|---|
1 |
Used to create a new buffer with the given size. |
2 |
Used to allocate a new buffer with size given. If size larger than buffer.constants.MAX_LENGTH or lesser than 0, ERR_INVALID_ARG_VALUE will be thrown. |
3 |
It will allocate a new buffer with given size. If size is larger than buffer.constants.MAX_LENGTH or lesser than 0, ERR_INVALID_ARG_VALUE will be thrown. |
4 |
Used to return the length in bytes for the buffer object. |
5 |
Used to compare two given buffer objects and returns a value that states the difference in comparison. |
6 |
Used to return a single buffer object by concatenating all the given buffer objects |
7 |
Used to create a new buffer using the array passed as bytes. |
8 |
It returns the true if the object given is a buffer. |
9 |
It returns true if the given encoding value is a supported character encoding and false if not. |
Buffer Class Methods
Following are the list of methods −
Sr.No | Module & Description |
---|---|
1 |
Used to read a signed 64-bit integer at a given offset from the buffer in little endian form. |
2 |
Used to deal with the comparison of two buffer objects and returns a value that states the difference in comparison. |
3 |
Used to read a signed big endian 64-bit integer at a given offset from a buffer object. |
4 |
It takes care of searching a value inside the buffer. It returns the position at which the value is present and -1 if the value is not present. |
5 |
Used to read an unsigned little-endian 64-bit integer at a given offset from the current buffer object. |
6 |
Used to read a big-endian double 64-bit number at a given offset from the current buffer object. |
7 |
Used to read a little-endian double 64-bit number at a given offset from the current buffer object. |
8 |
Used to read a big-endian float 32-bit number at a given offset from the current buffer object. |
9 |
Used to read a little-endian float 32-bit number at a given offset from the current buffer object. |
10 |
Used to read a 8-bit signed number at a given offset from the current buffer object. |
11 |
Used to write a big-endian double number at a given offset to the buffer created. |
12 |
Used to write a little-endian double number at a given offset to the buffer created. |
13 |
Used to write a big-endian float 32-bit number at a given offset to the current buffer object. |
14 |
Used to write a little-endian float 32-bit number at a given offset to the current buffer object. |
15 |
Used to copy the contents of the source buffer object into the target buffer. |
16 |
Used to create an iterator object from the buffer given and returns back a pair with [index, byte] from the contents of the buffer. |
17 |
Used to compare two buffers and returns true if it's equal and false if not. |
18 |
Used to fill the buffer with the given value . If the range inside the buffer is not specified it will fill the entire buffer. |
19 |
Used to check if the given value is available inside or included in the buffer. It returns true if present and false if not. |
20 |
It takes care of searching a value inside the buffer. It returns the position at which the value starts and -1 if the value is not present. |
21 |
Used to return an iterator object. When looped through that object , it will give the key for each byte in the buffer object. |
22 |
Used to read an unsigned big-endian 64-bit integer at a given offset from the current buffer object. |
23 |
Used to read a signed 16-bit integer at a given offset from the buffer in big endian form. |
24 |
Used to read a signed 16-bit integer at a given offset from the buffer in little endian form. |
25 |
Used to read a signed 32-bit integer at a given offset from the buffer in big endian form. |
26 |
Used to read a signed 32-bit integer at a given offset from the buffer in little endian form. |
27 |
Used to read a number of bytes in big endian format from a buffer at a given offset. |
28 |
Used to read a number of bytes in little endian format from a buffer at a given offset. |
29 |
Used to read a 8-bit unsigned number at a given offset from the current buffer object. |
30 |
Used to read an unsigned 16-bit integer at a given offset from the buffer in big endian form. |
31 |
Used to read an unsigned 16-bit integer at a given offset from the buffer in little endian form. |
32 |
Used to read an unsigned 32-bit integer at a given offset from the buffer in big endian form. |
33 |
Used to read an unsigned 32-bit integer at a given offset from the buffer in little endian form. |
34 |
Used to read a number of bytes as per the byte length and offset and return the unsigned integer in big endian format from a buffer used. |
35 |
Used to read a number of bytes as per the byte length and offset and return the unsigned integer in little endian format from a buffer used. |
36 |
Used to return a new buffer based on given start and end offset values on the original buffer. |
37 |
These considers the buffer as an array of unsigned 16 or 32 or 64 bit integers and swaps the byte order in-place and returns a reference to the buffer. |
38 |
Used to return a JSON object for the given buffer. |
39 |
Used to decode the string as per the encoding given. |
40 |
Used to return an iterator object that has details of all the bytes present in the buffer. |
41 |
Used to write the given string value to the buffer from the position given by the offset value. |
42 |
Used to write a signed 64-bit integer at a given offset to the buffer in big endian form. |
43 |
Used to write a signed 64-bit integer at a given offset to the buffer in little endian form. |
44 |
Used to write an unsigned 64-bit integer at a given offset to the buffer in little endian form. |
45 |
Used to write an signed 8-bit integer of value at a given offset to the buffer. |
46 |
Used to write a signed 16-bit integer at a given offset to the buffer in big endian form. |
47 |
Used to write a signed 16-bit integer at a given offset to the buffer in little endian form. |
48 |
Used to write a signed 32-bit integer at a given offset to the buffer in big endian form. |
49 |
Used to write a signed 32-bit integer at a given offset to the buffer in little endian form. |
50 |
Used to write a byte length bytes of value at a given offset to the buffer in big endian form. |
51 |
Used to write a bytelength bytes of value at a given offset to the buffer in little endian form. |
52 |
Used to write an unsigned 8-bit integer of value at a given offset to the buffer. |
53 |
Used to write a bytelength of an unsigned 16-bit integer of value at a given offset to the buffer in big endian form. |
54 |
Used to write an unsigned 16-bit integer of value at a given offset to the buffer in little endian form. |
55 |
Used to write an unsigned 32-bit integer of value at a given offset to the buffer in big endian form. |
56 |
Used to write an unsigned 32-bit integer of value at a given offset to the buffer in little endian form. |
57 |
Used to write a bytelength of unsigned bytes of value at a given offset to the buffer in big endian form. |
58 |
Used to write a bytelength of unsigned bytes of value at a given offset to the buffer in little endian form. |