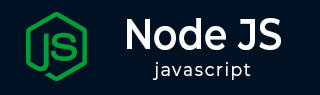
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Buffer.lastIndexOf() Method
The NodeJS Buffer.lastIndexOf() method takes care of searching a value inside the buffer.It returns the position at which the value is present and -1 if the value is not present. In case if there are multiple occurrences of the value in the buffer then the last position will be returned.
Syntax
Following is the syntax of the NodeJS lastIndexOf() Method −
buffer.lastIndexOf(value, byteoffset, encoding);
Parameters
The method buffer.lastIndexOf() takes in three parameters. The first parameter is a mandatory one and the rest are optional.
value − This is a mandatory parameter. It is the value to be searched inside the buffer. The value can be a string, a number or a buffer.
byteOffset − This is optional parameter. It tells about the offset from where to start searching. If negative offset value is given it will search from the end of the buffer. The default value is buffer.length-1.
encoding − If the value to be searched is a string then the encoding can be given using this parameter. It is an optional parameter. By default the encoding used is utf8.
Return value
The method Buffer.lastIndexOf() will return the last position it comes across for the value searched. It will give -1 if it is not present.
Example
In this example will try to search for a value inside the buffer created and test the result.
const buffer = Buffer.from('Welcome to TutorialsPoint'); if (buffer.lastIndexOf('Welcome') != -1) { console.log("The string welcome is present in the buffer"); } else { console.log("The string welcome is not present"); }
Output
We are searching for the string Welcome in the buffer created with the string "Welcome to Tutorialspoint". Since the given string is present we get below output. We check if the value is not equal to -1. Since -1 is returned when we don't have any match for the value given in the buffer.
The string welcome is present in the buffer
Example
Let us try by giving the byteOffset parameter in the example below −
const buffer = Buffer.from('Welcome to TutorialsPoint'); if (buffer.lastIndexOf('Point', -1) != -1) { console.log("The string point is present in the buffer"); } else { console.log("The string point is not present"); }
Output
We have used the byteOffset as -1. It will search in the string "Welcome to TutorialsPoint" from the end. Since the search string: Point is available you will get the output printed as "The string point is present in the buffer".
The string point is present in the buffer
Example
In this example will make use of a buffer as the value to check if it's available inside the given buffer.
const buffer = Buffer.from('Welcome to TutorialsPoint'); const result = buffer.lastIndexOf(Buffer.from('TutorialsPoint')); if (result != -1) { console.log("The string TutorialsPoint is present in the buffer"); } else { console.log("The string TutorialsPoint is not present"); }
Output
Inside the Buffer.lastIndexOf() method we are making use of a buffer that has the string value: TutorialsPoint. Our main buffer is having a string:Welcome to TutorialsPoint. So since the string is present the value returned is the start position of the string.
The string TutorialsPoint is present in the buffer
Example
Let us try a false case that is to check the response of Buffer.lastIndexOf() when the string is not present.
const buffer = Buffer.from('Welcome to TutorialsPoint'); if (buffer.lastIndexOf('Testing') != -1) { console.log("The string Testing is present in the buffer"); } else { console.log("The string Testing is not present"); }
Output
We are searching for the string: Testing inside the buffer with string: Welcome to TutorialsPoint. Since it is not present it will return the value -1. Hence you will get the output as: The string Testing is not present.
The string Testing is not present
Example
Let us test the encoding parameter inside Buffer.lastIndexOf().
const buffer = Buffer.from('Hello World'); if (buffer.lastIndexOf('Hello','hex') != -1) { console.log("The string Hello is present in the buffer"); } else { console.log("The string Hello is not present"); }
Output
We have used "hex" encoding. When checked with encoding and the string is present it returns the start position of the string.
The string Hello is present in the buffer
Example
In this example will make use of integer value i.e., the utf-8 encoding value to search in the buffer.
const buffer = Buffer.from('Hello World'); if (buffer.lastIndexOf(72) != -1) { console.log("The character H is present in the buffer"); } else { console.log("The string H is not present"); }
Output
Here 72 represents the character 'H' as per utf-8 encoding.When searched with the integer value in the buffer , it returns the position of character H since it is present in the buffer.
The string Hello is present in the buffer
Example
Let us check an example where there are multiple occurrences of the same string.
In the example we are checking the position using indexOf and lastIndexOf(). Using lastIndexOf() it gives the position of the string that appears last and using indexOf() it gives the position of the string that comes first.
const buffer = Buffer.from('She sells sea shells on the sea shore'); console.log("The position of sea using lastIndexOf() is "+ buffer.lastIndexOf("sea")); console.log("The position of sea using indexOf() is "+ buffer.indexOf("sea"));
Output
The position of sea using lastIndexOf() is 28 The position of sea using indexOf() is 10
To Continue Learning Please Login