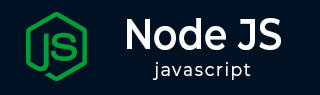
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Buffer.readIntLE() Method
The NodeJS Buffer.readIntLE() method will read a number of bytes in little endian format from a buffer at a given offset.
Syntax
Following is the syntax of the Node.JS Buffer.readIntLE() Method −
buf.readIntLE(offset, byteLength)
Parameters
This method accepts a single parameter. The same is explained below.
offset − The offset that indicates the position to start reading. The offset is greater than or equal to 0 and also less than or equal to buffer.length-bytelength. The default value is 0.
byteLength − The number of bytes you want to read. The byteLength has to be between 0 to 6.
Return value
This method returns the result in little endian with a bytelength number of bytes at a given offset value from the buffer.
Example
To create a buffer, we are going to make use of NodeJS Buffer.from() method −
const buffer = Buffer.from([11, 12, 13, 14, 15, 16]); console.log(buffer); console.log(buffer.readIntLE(0, 2));
Output
The offset we have used with this method is 0. The bytelength used is 2. So from 0th offset it will read the bytes with bytelength 2 and return the result in little endian format.
<Buffer 0b 0c 0d 0e 0f 10> 3083
Example
In this example let us print the output we get in hexadecimal form as shown below −
const buffer = Buffer.from([11, 12, 13, 14, 15, 16]); console.log(buffer); console.log(buffer.readIntLE(0, 2).toString(16));
Output
<Buffer 0b 0c 0d 0e 0f 10> c0b
Example
As we know we can make use of offset from 0 to buffer.length-bytelength. So if the buffer length is 6, and bytelength used is 6 we can use offset as 0. Using a value greater than 0 will give you an error : ERR_OUT_OF_RANGE.
const buffer = Buffer.from([11, 12, 13, 14, 15, 16]); console.log(buffer); console.log("The buffer length is :"+ buffer.length); console.log(buffer.readIntLE(1, 6).toString(16));
Output
<Buffer 0b 0c 0d 0e 0f 10> The buffer length is :6 internal/buffer.js:58 throw new ERR_OUT_OF_RANGE(type || 'offset', ^ RangeError [ERR_OUT_OF_RANGE]: The value of "offset" is out of range. It must be >= 0 and <= 0. Received 1 at boundsError (internal/buffer.js:58:9) at readInt48LE (internal/buffer.js:257:5) at Buffer.readIntLE (internal/buffer.js:237:12) at Object.<anonymous> (C:\nodejsProject\src\testbuffer.js:4:20) at Module._compile (internal/modules/cjs/loader.js:816:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:827:10) at Module.load (internal/modules/cjs/loader.js:685:32) at Function.Module._load (internal/modules/cjs/loader.js:620:12) at Function.Module.runMain (internal/modules/cjs/loader.js:877:12) at internal/main/run_main_module.js:21:11