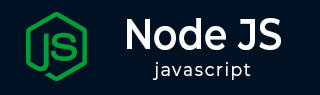
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Buffer.values() Method
The NodeJS Buffer.values() method returns an iterator object that has details of all the bytes present in the buffer. You can make use of for…of loop to iterate over iterator objects.
Syntax
Following is the syntax of the Node.JS Buffer.values() Method −
buf.values()
Parameters
There are no parameters for this method.
Return value
The method buffer.values() returns an iterator object.
Example
To create a buffer, we are going to make use of NodeJS Buffer.from() method −
const buffer = Buffer.from("Hello"); console.log(buffer); for (const val of buffer.values()) { console.log(val); }
Output
We have used the string "hello" to create a buffer. Using Buffer.values() will get the bytes from the buffer created. On executing the above program, it will generate the following output −
<Buffer 48 65 6c 6c 6f> 72 101 108 108 111
Example
In the below example we have used the buffer.values() method to get the bytes from the buffer created. We can also iterate directly over the buffer to get the same result.
const buffer = Buffer.from("Hello"); console.log(buffer); for (const val of buffer.values()) { console.log(val); } console.log("\n"); for (const val of buffer) { console.log(val); }
Output
We have used the string "hello" to create a buffer. To get the bytes we have iterated over Buffer.values() and later directly on Buffer.from() output. On executing the above program, it will generate the following output −
<Buffer 48 65 6c 6c 6f> 72 101 108 108 111 72 101 108 108 111
Example
In the example we have used Buffer.alloc() to allocate memory for the buffer. Later buffer.fill() method is used to fill the buffer with a value. Buffer.values() is used to see the bytes in the buffer created.
const buffer = Buffer.alloc(5); buffer.fill("h"); console.log(buffer); for (const val of buffer.values()) { console.log(val); }
Output
<Buffer 68 68 68 68 68> 104 104 104 104 104