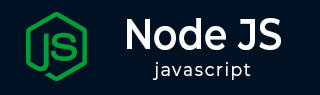
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - Buffer.readBigInt64LE() Method
The NodeJS Buffer.readBigInt64LE() method helps in reading a signed 64-bit integer at a given offset from the buffer in little endian form. The integer read from the buffer is represented as two's complement signed values.
A little bit of understanding on signed, unsigned integers and two's complement.
A signed 64-bit integer will have a minimum value as -9,223,372,036,854,775,808 and maximum value as 9,223,372,036,854,775,807. Whereas an unsigned 64-bit integer will have minimum value as 0 and maximum value as 264-1.
A signed integer can take positive and negative values whereas an unsigned integer will only have positive values.
Now coming to Two's complement it is a mathematical operation on binary numbers. To get the two's complete of any given binary number it will be as follows −
Binary No: 101011
Step 1 − First find the one's complement of binary number: 101011. To get the one's complete you need to make 1 as 0 and 0 as 1. So the one's complement of the above binary number is: 010100.
Step 2 − Now for the one's complement from step 1 add 1 to it.
I.e. 010100 + 1 = 010101. So 010101 is the two's complement of binary number 101011.
Syntax
Following is the syntax of the Node.JS Buffer.readBigInt64LE() Method −
buf.readBigInt64LE(offset)
Parameters
This method accepts a single parameter. The same is explained below.
offset − The offset here indicates the position to start reading. The offset has to be greater than or equal to 0 and less than or equal to buffer.length-8. The default value is 0.
Return value
This method returns the 64-bit signed integer value of the contents of the current buffer at the given offset.
Example
To create a buffer, we are going to make use of NodeJS Buffer.from() method −
const buffer = Buffer.from([0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff]); console.log("buffer stored in memory as", buffer); console.log("Reading at big integer at offset 0:", buffer.readBigInt64LE(0));
Output
The offset we have used with this method is 0. On execution the 64-bit signed integer at the 0th position will be returned. The buffer length created for the above is 8. So we can only use the offset with only value as 0. If any value >0 it will give an error ERR_OUT_OF_RANGE.
buffer stored in memory as <Buffer 00 00 00 00 ff ff ff ff> Reading at big integer at offset 0: -4294967296n
Example
Let us create a buffer with 16 bits and see the value returned using the Node.JS Buffer.readBigInt64LE() method.
const buffer = Buffer.from([0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff,0xff, 0xff, 0xff, 0xff,0xff, 0xff, 0xff, 0xff]); console.log("Length of buffer is ", buffer.length); console.log("Reading at big integer at offset 0:", buffer.readBigInt64LE(2));
Output
Length of buffer is 16 Reading at big integer at offset 0: -65536n
In the above example, the length of the buffer created is 16. So for offset, we can use values from 0 to 8. With offset as 8 the output is as follows −
Length of buffer is 16 Reading at big integer at offset 8: -1n
Example
This example will check on the error if the offset is greater than the buffer.length -8. Let us create a buffer having a length of 8 and with that, you can offset the value as 1.
const buffer = Buffer.from([0x00, 0x00, 0x00, 0x00, 0xff, 0xff, 0xff, 0xff]); console.log("buffer length is ", buffer.length); console.log("Reading at big integer at offset 1:", buffer.readBigInt64LE(1));
Output
buffer length is 8 internal/buffer.js:83 throw new ERR_OUT_OF_RANGE(type || 'offset', ^ RangeError [ERR_OUT_OF_RANGE]: The value of "offset" is out of range. It must be >= 0 and <= 0. Received 1 at boundsError (internal/buffer.js:83:9) at Buffer.readBigInt64LE (internal/buffer.js:134:5) at Object.<anonymous> (C:\nodejsProject\src\testbuffer.js:3:59) at Module._compile (internal/modules/cjs/loader.js:1063:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:1092:10) at Module.load (internal/modules/cjs/loader.js:928:32) at Function.Module._load (internal/modules/cjs/loader.js:769:14) at Function.executeUserEntryPoint [as runMain] (internal/modules/run_main.js:72:12) at internal/main/run_main_module.js:17:47 { code: 'ERR_OUT_OF_RANGE'
To Continue Learning Please Login